mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Scribunto
synced 2024-11-24 08:14:09 +00:00
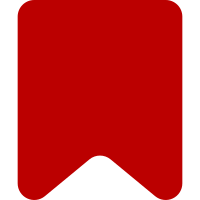
Two similar bugs are handled here: * mw.getCurrentFrame() doesn't work when the module is loaded (only when a function is called), which breaks os.date and os.time at module scope since I59ad364d. * mw.getCurrentFrame() gives access to frame args from inside mw.loadData, which allows for data leakage between #invokes. Bug: 67498 Bug: 65687 Change-Id: I82dde43e2601b59c03c6ed4b9365829c40a953a5
53 lines
1.3 KiB
PHP
53 lines
1.3 KiB
PHP
<?php
|
|
|
|
class Scribunto_LuaDataProvider implements Iterator {
|
|
protected $engine = null;
|
|
protected $exports = null;
|
|
protected $key = 1;
|
|
|
|
public function __construct( $engine, $moduleName ) {
|
|
$this->engine = $engine;
|
|
$this->key = 1;
|
|
$module = $engine->fetchModuleFromParser(
|
|
Title::makeTitle( NS_MODULE, $moduleName )
|
|
);
|
|
if ( $module === null ) {
|
|
throw new Exception( "Failed to load module $moduleName" );
|
|
}
|
|
// Calling executeModule with null isn't the best idea, since it brings
|
|
// the whole export table into PHP and throws away metatables and such,
|
|
// but for this use case, we don't have anything like that to worry about
|
|
$this->exports = $engine->executeModule( $module->getInitChunk(), null, null );
|
|
}
|
|
|
|
public function destroy() {
|
|
$this->engine = null;
|
|
$this->exports = null;
|
|
}
|
|
|
|
public function rewind() {
|
|
$this->key = 1;
|
|
}
|
|
|
|
public function valid() {
|
|
return $this->key <= $this->exports['count'];
|
|
}
|
|
|
|
public function key() {
|
|
return $this->key;
|
|
}
|
|
|
|
public function next() {
|
|
$this->key++;
|
|
}
|
|
|
|
public function current() {
|
|
return $this->engine->getInterpreter()->callFunction( $this->exports['provide'], $this->key );
|
|
}
|
|
|
|
public function run( $key ) {
|
|
list( $ret ) = $this->engine->getInterpreter()->callFunction( $this->exports['run'], $key );
|
|
return $ret;
|
|
}
|
|
}
|