mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Scribunto
synced 2024-11-15 11:59:42 +00:00
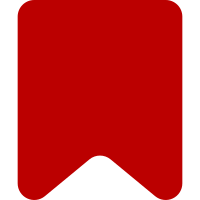
* Added tests for the engine classes. * Added some tests that run under Lua. * In the chunk names, fixed truncation of module names at 60 bytes by using an "=" prefix instead of @. * Fixed a bug in mw.clone() which was causing the metatable to be set on the source table instead of the destination. * Put restricted setfenv/getfenv in the cloned environment rather than the base environment, they work better that way. * In setfenv(), check for getfenv() == nil, since that's what our own restricted getfenv returns. * Fixed getfenv() handling of numeric arguments: add one where appropriate. Change-Id: I2b356fd65a3fcb348c4e99a3a4267408fb995739
119 lines
3.1 KiB
PHP
119 lines
3.1 KiB
PHP
<?php
|
|
|
|
abstract class Scribunto_LuaInterpreterTest extends MediaWikiTestCase {
|
|
abstract function newInterpreter( $opts = array() );
|
|
|
|
function setUp() {
|
|
try {
|
|
$this->newInterpreter();
|
|
} catch ( Scribunto_LuaInterpreterNotFoundError $e ) {
|
|
$this->markTestSkipped( "interpreter not available" );
|
|
}
|
|
}
|
|
|
|
function getBusyLoop( $interpreter ) {
|
|
$chunk = $interpreter->loadString( '
|
|
local args = {...}
|
|
local x, i
|
|
local s = string.rep("x", 1000000)
|
|
local n = args[1]
|
|
for i = 1, n do
|
|
x = x or string.find(s, "y", 1, true)
|
|
end',
|
|
'busy' );
|
|
return $chunk;
|
|
}
|
|
|
|
function getPassthru( $interpreter ) {
|
|
return $interpreter->loadString( 'return ...', 'passthru' );
|
|
}
|
|
|
|
/** @dataProvider provideRoundtrip */
|
|
function testRoundtrip( /*...*/ ) {
|
|
$args = func_get_args();
|
|
$args = $this->normalizeOrder( $args );
|
|
$interpreter = $this->newInterpreter();
|
|
$passthru = $interpreter->loadString( 'return ...', 'passthru' );
|
|
$finalArgs = $args;
|
|
array_unshift( $finalArgs, $passthru );
|
|
$ret = call_user_func_array( array( $interpreter, 'callFunction' ), $finalArgs );
|
|
$ret = $this->normalizeOrder( $ret );
|
|
$this->assertSame( $args, $ret );
|
|
}
|
|
|
|
/** @dataProvider provideRoundtrip */
|
|
function testDoubleRoundtrip( /* ... */ ) {
|
|
$args = func_get_args();
|
|
$args = $this->normalizeOrder( $args );
|
|
|
|
$interpreter = $this->newInterpreter();
|
|
$interpreter->registerLibrary( 'test',
|
|
array( 'passthru' => array( $this, 'passthru' ) ) );
|
|
$doublePassthru = $interpreter->loadString(
|
|
'return test.passthru(...)', 'doublePassthru' );
|
|
|
|
$finalArgs = $args;
|
|
array_unshift( $finalArgs, $doublePassthru );
|
|
$ret = call_user_func_array( array( $interpreter, 'callFunction' ), $finalArgs );
|
|
$ret = $this->normalizeOrder( $ret );
|
|
$this->assertSame( $args, $ret );
|
|
}
|
|
|
|
function normalizeOrder( $a ) {
|
|
ksort( $a );
|
|
foreach ( $a as &$value ) {
|
|
if ( is_array( $value ) ) {
|
|
$value = $this->normalizeOrder( $value );
|
|
}
|
|
}
|
|
return $a;
|
|
}
|
|
|
|
function passthru( /* ... */ ) {
|
|
$args = func_get_args();
|
|
return $args;
|
|
}
|
|
|
|
function provideRoundtrip() {
|
|
return array(
|
|
array( 1 ),
|
|
array( true ),
|
|
array( false ),
|
|
array( 'hello' ),
|
|
array( implode( '', array_map( 'chr', range( 0, 255 ) ) ) ),
|
|
array( 1, 2, 3 ),
|
|
array( array() ),
|
|
array( array( 0 => 'foo', 1 => 'bar' ) ),
|
|
array( array( 1 => 'foo', 2 => 'bar' ) ),
|
|
array( array( 'x' => 'foo', 'y' => 'bar', 'z' => array() ) )
|
|
);
|
|
}
|
|
|
|
/**
|
|
* @expectedException ScribuntoException
|
|
* @expectedExceptionMessage The time allocated for running scripts has expired.
|
|
*/
|
|
function testTimeLimit() {
|
|
$interpreter = $this->newInterpreter( array( 'cpuLimit' => 1 ) );
|
|
$chunk = $this->getBusyLoop( $interpreter );
|
|
$interpreter->callFunction( $chunk, 1e9 );
|
|
}
|
|
|
|
/**
|
|
* @expectedException ScribuntoException
|
|
* @expectedExceptionMessage Lua error: not enough memory
|
|
*/
|
|
function testTestMemoryLimit() {
|
|
$interpreter = $this->newInterpreter( array( 'memoryLimit' => 20 * 1e6 ) );
|
|
$chunk = $interpreter->loadString( '
|
|
t = {}
|
|
for i = 1, 10 do
|
|
t[#t + 1] = string.rep("x" .. i, 1000000)
|
|
end
|
|
',
|
|
'memoryLimit' );
|
|
$interpreter->callFunction( $chunk );
|
|
}
|
|
}
|
|
|