mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Scribunto
synced 2024-11-15 11:59:42 +00:00
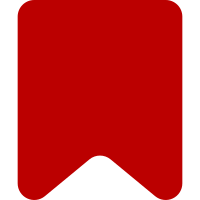
* Added tests for the engine classes. * Added some tests that run under Lua. * In the chunk names, fixed truncation of module names at 60 bytes by using an "=" prefix instead of @. * Fixed a bug in mw.clone() which was causing the metatable to be set on the source table instead of the destination. * Put restricted setfenv/getfenv in the cloned environment rather than the base environment, they work better that way. * In setfenv(), check for getfenv() == nil, since that's what our own restricted getfenv returns. * Fixed getfenv() handling of numeric arguments: add one where appropriate. Change-Id: I2b356fd65a3fcb348c4e99a3a4267408fb995739
95 lines
2.6 KiB
PHP
95 lines
2.6 KiB
PHP
<?php
|
|
|
|
abstract class Scribunto_LuaEngineTest extends MediaWikiTestCase {
|
|
|
|
abstract function newEngine( $opts = array() );
|
|
|
|
function setUp() {
|
|
try {
|
|
$this->getEngine()->getInterpreter();
|
|
} catch ( Scribunto_LuaInterpreterNotFoundError $e ) {
|
|
$this->markTestSkipped( "interpreter not available" );
|
|
}
|
|
}
|
|
|
|
function getEngine() {
|
|
$parser = new Parser;
|
|
$options = new ParserOptions;
|
|
$options->setTemplateCallback( array( $this, 'templateCallback' ) );
|
|
$parser->startExternalParse( Title::newMainPage(), $options, Parser::OT_HTML, true );
|
|
return $this->newEngine( array( 'parser' => $parser ) );
|
|
}
|
|
|
|
function getFrame( $engine ) {
|
|
return $engine->getParser()->getPreprocessor()->newFrame();
|
|
}
|
|
|
|
function getTestModules() {
|
|
return array(
|
|
'CommonTests' => dirname( __FILE__ ) . '/CommonTests.lua'
|
|
);
|
|
}
|
|
|
|
function templateCallback( $title, $parser ) {
|
|
$modules = $this->getTestModules();
|
|
foreach ( $modules as $name => $fileName ) {
|
|
$modTitle = Title::makeTitle( NS_MODULE, $name );
|
|
if ( $modTitle->equals( $title ) ) {
|
|
return array(
|
|
'text' => file_get_contents( $fileName ),
|
|
'finalTitle' => $title,
|
|
'deps' => array()
|
|
);
|
|
}
|
|
}
|
|
return Parser::statelessFetchTemplate( $title, $parser );
|
|
}
|
|
|
|
function getTestModuleName() {
|
|
return 'CommonTests';
|
|
}
|
|
|
|
function getTestModule( $engine, $moduleName ) {
|
|
return $engine->fetchModuleFromParser(
|
|
Title::makeTitle( NS_MODULE, $moduleName ) );
|
|
}
|
|
|
|
function testProvider() {
|
|
$tests = $this->provideLua();
|
|
$this->assertGreaterThan( 2, count( $tests ) );
|
|
}
|
|
|
|
function provideLua() {
|
|
$engine = $this->getEngine();
|
|
$allTests = array();
|
|
foreach ( $this->getTestModules() as $moduleName => $fileName ) {
|
|
$module = $this->getTestModule( $engine, $moduleName );
|
|
$moduleTests = $module->invoke( 'getTests', array(), $this->getFrame( $engine ) );
|
|
foreach ( $moduleTests as $test ) {
|
|
array_unshift( $test, $moduleName );
|
|
$allTests[] = $test;
|
|
}
|
|
}
|
|
return $allTests;
|
|
}
|
|
|
|
/** @dataProvider provideLua */
|
|
function testLua( $moduleName, $testName, $expected ) {
|
|
$engine = $this->getEngine();
|
|
$module = $this->getTestModule( $engine, $moduleName );
|
|
if ( isset( $expected['error'] ) ) {
|
|
$caught = false;
|
|
try {
|
|
$ret = $module->invoke( $testName, array(), $this->getFrame( $engine ) );
|
|
} catch ( Scribunto_LuaError $e ) {
|
|
$caught = true;
|
|
$this->assertStringMatchesFormat( $expected['error'], $e->getLuaMessage() );
|
|
}
|
|
$this->assertTrue( $caught, 'expected an exception' );
|
|
} else {
|
|
$ret = $module->invoke( $testName, array(), $this->getFrame( $engine ) );
|
|
$this->assertSame( $expected, $ret );
|
|
}
|
|
}
|
|
}
|