mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Scribunto
synced 2024-09-23 18:30:32 +00:00
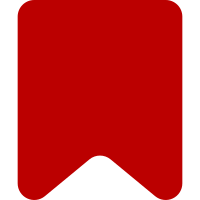
Currently, the time taken to parse the arguments passed to a Lua function from #invoke will be counted against Lua's 10-second limit. This is counterintuitive, and can remove incentive for users to convert templates to Lua since they may have to convert a whole stack at once. Note this requires change I11881232 to mediawiki/php/luasandbox to actually have any effect. Bug: 45684 Change-Id: I773950e4c399b8a1cfa6d1cde781a069d286b3bd
63 lines
1.7 KiB
PHP
63 lines
1.7 KiB
PHP
<?php
|
|
|
|
abstract class Scribunto_LuaInterpreter {
|
|
/**
|
|
* Load a string. Return an object which can later be passed to callFunction.
|
|
* If there is a pass error, a Scribunto_LuaError will be thrown.
|
|
*
|
|
* @param $text The Lua source code
|
|
* @param $chunkName The chunk name
|
|
*/
|
|
abstract public function loadString( $text, $chunkName );
|
|
|
|
/**
|
|
* Call a Lua function. Return an array of results, with indices starting
|
|
* at zero. If an error occurs, a Scribunto_LuaError will be thrown.
|
|
*
|
|
* @param $func The function object
|
|
*/
|
|
abstract public function callFunction( $func /*...*/ );
|
|
|
|
/**
|
|
* Wrap a PHP callable as a Lua function, which can be passed back into
|
|
* Lua. If an error occurs, a Scribunto_LuaError will be thrown.
|
|
*
|
|
* @param $callable The PHP callable
|
|
* @return a Lua function
|
|
*/
|
|
abstract public function wrapPhpFunction( $callable );
|
|
|
|
/**
|
|
* Test whether an object is a Lua function.
|
|
*
|
|
* @param $object
|
|
* @return boolean
|
|
*/
|
|
abstract public function isLuaFunction( $object );
|
|
|
|
/**
|
|
* Register a library of functions.
|
|
*
|
|
* @param $name string The global variable name to be created or added to.
|
|
* @param $functions array An associative array mapping the function name to the
|
|
* callback. The callback may throw a Scribunto_LuaError, which will be
|
|
* caught and raised in the Lua code as a Lua error, catchable with
|
|
* pcall().
|
|
*/
|
|
abstract public function registerLibrary( $name, $functions );
|
|
|
|
/**
|
|
* Pause CPU usage and limits
|
|
* @return void
|
|
*/
|
|
abstract public function pauseUsageTimer();
|
|
|
|
/**
|
|
* Unpause CPU usage and limits
|
|
* @return void
|
|
*/
|
|
abstract public function unpauseUsageTimer();
|
|
}
|
|
|
|
class Scribunto_LuaInterpreterNotFoundError extends MWException {}
|