mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Scribunto
synced 2024-11-24 00:05:00 +00:00
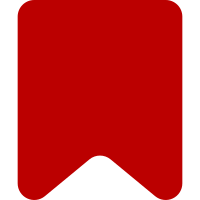
Coding style: * Avoid meaningless '_' in variable names, especially when used inconsistently. * Avoid trailing line comments. * Consistent if/else curly brace position. * Consistently use single quotes (there are no magic quotes in js). * Consistently use $ in variable names of jQuery-wrapped elements (as opposed to plain node references). * Avoid using variable names like '_this' or 'that', instead name them after the object. * Too many var statements. * Hoist var statement. * Fix alignment of closing parentheses in initEditPage. Code quality: * Remove commented out code. * Add missing radix parameter for parseInt. * Remove unused private function "printWithRunin". * Remove unused parameters. * Don't call "console.log" in production client-side code because the console doesn't always exist in normal browser modes (and would result in an Uncaught ReferenceError, aborting the script unexpectedly and leaving the user interface in a likely unresponsive state). * Use the Promise.done and Promise.fail handlers of mw.Api, instead of the deprecated 'ok' and 'err' parameters. * Use jQuery#on instead of the deprecated jQuery#bind. * Use a local shared reference to the singleton instead of relying on 'this' context, this way the methods can be called regardless of context. Such as in the $(document).ready(), or when passing around setErrors callback. * Avoid using invalid html shortcuts like <div/>, use <tag> for creation, and <tag>..</tag> for parsing (per style guide). * Document inputKeydown parameter being jQuery.Event (as oppposed to native Event). Misc: * Renamed '_in' to 'in', and renamed again to 'input' ('in' is an illegal variable name and would've crashed). Change-Id: I283fda1409b1e76db56a939183bdaefc95e60961
48 lines
1.1 KiB
JavaScript
48 lines
1.1 KiB
JavaScript
( function ( $, mw ) {
|
|
|
|
var scribunto = mw.scribunto = {
|
|
errors: null,
|
|
|
|
setErrors: function ( errors ) {
|
|
scribunto.errors = errors;
|
|
},
|
|
|
|
init: function () {
|
|
var regex = /^mw-scribunto-error-(\d+)/,
|
|
$dialog = $( '<div>' );
|
|
|
|
$dialog.dialog( {
|
|
title: mw.msg( 'scribunto-parser-dialog-title' ),
|
|
autoOpen: false
|
|
} );
|
|
|
|
$( '.scribunto-error' ).each( function ( index, span ) {
|
|
var errorId,
|
|
matches = regex.exec( span.id );
|
|
if ( matches === null ) {
|
|
mw.log( 'mw.scribunto.init: regex mismatch!' );
|
|
return;
|
|
}
|
|
errorId = parseInt( matches[1], 10 );
|
|
$( span ).on( 'click', function ( e ) {
|
|
if ( typeof scribunto.errors[ errorId ] !== 'string' ) {
|
|
mw.log( 'mw.scribunto.init: error ' + matches[1] + ' not found, ' +
|
|
'mw.loader.using() callback may not have been called yet.' );
|
|
return;
|
|
}
|
|
var error = scribunto.errors[ errorId ];
|
|
$dialog
|
|
.dialog( 'close' )
|
|
.html( error )
|
|
.dialog( 'option', 'position', [ e.clientX + 5, e.clientY + 5 ] )
|
|
.dialog( 'open' );
|
|
} );
|
|
} );
|
|
}
|
|
};
|
|
|
|
$( mw.scribunto.init );
|
|
|
|
} ) ( jQuery, mediaWiki );
|
|
|