mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Scribunto
synced 2024-11-13 18:07:05 +00:00
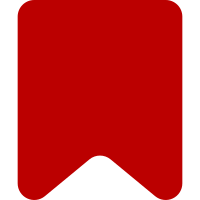
* Introduced a Lua implementation based on shelling out to a standard Lua binary. * Bundled several Lua binaries for common platforms. I haven't added a 32-bit Linux binary yet, but that will come. * Refactored the existing Lua class, bringing out functionality common to all Lua implementations into a set of common base classes. * Moved the bulk of the implementation-specific functionality into a set of "interpreter" classes. * Renamed LuaSandboxEngine to Scribunto_LuaSandboxEngine * Don't create an engine object unconditionally when the ParserLimitReport hook is called. * Implemented isolation of module global variable namespaces. This means that separate {{#invoke}} calls can't pass data to each other -- this was a desired feature in planning since it allows more flexibility in wikitext parser design. Isolation for mw.import() means that modules cannot accidentally create global variables which affect other modules -- exports are solely via the return value. Change-Id: I3fa35651fe5b1fbfd85adeadc220b1ea31cd6f0b
185 lines
4.9 KiB
PHP
185 lines
4.9 KiB
PHP
<?php
|
|
|
|
/**
|
|
* Wikitext scripting infrastructure for MediaWiki: base classes.
|
|
* Copyright (C) 2012 Victor Vasiliev <vasilvv@gmail.com> et al
|
|
* http://www.mediawiki.org/
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License along
|
|
* with this program; if not, write to the Free Software Foundation, Inc.,
|
|
* 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.
|
|
* http://www.gnu.org/copyleft/gpl.html
|
|
*/
|
|
|
|
/**
|
|
* Base class for all script engines. Includes all code
|
|
* not related to particular modules, like tracking links between
|
|
* modules or loading module texts.
|
|
*/
|
|
abstract class ScribuntoEngineBase {
|
|
protected
|
|
$parser,
|
|
$options,
|
|
$modules = array();
|
|
|
|
/**
|
|
* Creates a new module object within this engine
|
|
*/
|
|
abstract protected function newModule( $text, $chunkName );
|
|
|
|
/**
|
|
* Constructor.
|
|
*
|
|
* @param $options Associative array of options:
|
|
* - parser: A Parser object
|
|
*/
|
|
public function __construct( $options ) {
|
|
$this->options = $options;
|
|
if ( isset( $options['parser'] ) ) {
|
|
$this->parser = $options['parser'];
|
|
}
|
|
}
|
|
|
|
/**
|
|
* Load a module from some parser-defined template loading mechanism and
|
|
* register a parser output dependency.
|
|
*
|
|
* Does not initialize the module, i.e. do not expect it to complain if the module
|
|
* text is garbage or has syntax error. Returns a module or throws a
|
|
* ScribuntoException.
|
|
*
|
|
* @param $title The title of the module
|
|
* @return ScribuntoEngineModule
|
|
*/
|
|
function fetchModuleFromParser( Title $title ) {
|
|
list( $text, $finalTitle ) = $this->parser->fetchTemplateAndTitle( $title );
|
|
if ( $text === false ) {
|
|
throw new ScribuntoException( 'scribunto-common-nosuchmodule' );
|
|
}
|
|
|
|
$key = $finalTitle->getPrefixedDBkey();
|
|
if ( !isset( $this->modules[$key] ) ) {
|
|
$this->modules[$key] = $this->newModule( $text, $key );
|
|
}
|
|
return $this->modules[$key];
|
|
}
|
|
|
|
/**
|
|
* Validates the script and returns an array of the syntax errors for the
|
|
* given code.
|
|
*
|
|
* @param $code Code to validate
|
|
* @param $title Title of the code page
|
|
* @return array
|
|
*/
|
|
function validate( $text, $chunkName = false ) {
|
|
$module = $this->newModule( $text, $chunkName );
|
|
|
|
try {
|
|
$module->initialize();
|
|
} catch( ScribuntoException $e ) {
|
|
return array( $e->getMessage() );
|
|
}
|
|
|
|
return array();
|
|
}
|
|
|
|
/**
|
|
* Allows the engine to append their information to the limits
|
|
* report.
|
|
*/
|
|
public function getLimitsReport() {
|
|
/* No-op by default */
|
|
return '';
|
|
}
|
|
|
|
/**
|
|
* Get the language for GeSHi syntax highlighter.
|
|
*/
|
|
function getGeSHiLanguage() {
|
|
return false;
|
|
}
|
|
|
|
/**
|
|
* Get the language for Ace code editor.
|
|
*/
|
|
function getCodeEditorLanguage() {
|
|
return false;
|
|
}
|
|
}
|
|
|
|
/**
|
|
* Class that represents a module. Responsible for initial module parsing
|
|
* and maintaining the contents of the module.
|
|
*/
|
|
abstract class ScribuntoModuleBase {
|
|
var $engine, $code, $chunkName;
|
|
|
|
public function __construct( $engine, $code, $chunkName ) {
|
|
$this->engine = $engine;
|
|
$this->code = $code;
|
|
$this->chunkName = $chunkName;
|
|
}
|
|
|
|
/** Accessors **/
|
|
public function getEngine() { return $this->engine; }
|
|
public function getCode() { return $this->code; }
|
|
public function getChunkName() { return $this->chunkName; }
|
|
|
|
/**
|
|
* Initialize the module. That means parse it and load the
|
|
* functions/constants/whatever into the object.
|
|
*
|
|
* Protection of double-initialization is the responsibility of this method.
|
|
*/
|
|
abstract function initialize();
|
|
|
|
/**
|
|
* Returns the object for a given function. Should return null if it does not exist.
|
|
*
|
|
* @return ScribuntoFunctionBase or null
|
|
*/
|
|
abstract function getFunction( $name );
|
|
|
|
/**
|
|
* Returns the list of the functions in the module.
|
|
*
|
|
* @return array(string)
|
|
*/
|
|
abstract function getFunctions();
|
|
}
|
|
|
|
abstract class ScribuntoFunctionBase {
|
|
protected $mName, $mContents, $mModule, $mEngine;
|
|
|
|
public function __construct( $module, $name, $contents ) {
|
|
$this->name = $name;
|
|
$this->contents = $contents;
|
|
$this->module = $module;
|
|
$this->engine = $module->getEngine();
|
|
}
|
|
|
|
/**
|
|
* Calls the function. Returns its first result or null if no result.
|
|
*
|
|
* @param $args array Arguments to the function
|
|
* @param $frame PPFrame
|
|
*/
|
|
abstract public function call( $args, $frame );
|
|
|
|
/** Accessors **/
|
|
public function getName() { return $this->name; }
|
|
public function getModule() { return $this->module; }
|
|
public function getEngine() { return $this->engine; }
|
|
}
|