mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Scribunto
synced 2024-11-24 08:14:09 +00:00
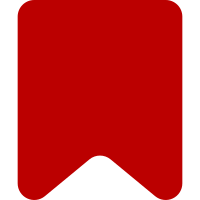
mw.text.unstrip is too broad, it's allowing for unstripping things that cause problems when unstripped (e.g. bug 61268). Since the original request was only for unstripping <nowiki>, let's add a function that does only that. We should also add an interface to StripState::killMarkers(), instead of requiring everyone to roll their own work-alike. Then, to fix the bug, we can make mw.text.unstrip be the combination of the two. This is the most like the original behavior of mw.text.unstrip (removes all strip markers, replacing them with text where applicable) without causing issues. Bug: 61268 Change-Id: I3a151fd678b365d629b71b4f1cb0d5d284b98555
66 lines
2.1 KiB
PHP
66 lines
2.1 KiB
PHP
<?php
|
|
|
|
class Scribunto_LuaTextLibrary extends Scribunto_LuaLibraryBase {
|
|
function register() {
|
|
global $wgUrlProtocols;
|
|
|
|
$lib = array(
|
|
'unstrip' => array( $this, 'textUnstrip' ),
|
|
'unstripNoWiki' => array( $this, 'textUnstripNoWiki' ),
|
|
'killMarkers' => array( $this, 'killMarkers' ),
|
|
'getEntityTable' => array( $this, 'getEntityTable' ),
|
|
);
|
|
$opts = array(
|
|
'comma' => wfMessage( 'comma-separator' )->inContentLanguage()->text(),
|
|
'and' => wfMessage( 'and' )->inContentLanguage()->text() .
|
|
wfMessage( 'word-separator' )->inContentLanguage()->text(),
|
|
'ellipsis' => wfMessage( 'ellipsis' )->inContentLanguage()->text(),
|
|
'nowiki_protocols' => array(),
|
|
);
|
|
|
|
foreach ( $wgUrlProtocols as $prot ) {
|
|
if ( substr( $prot, -1 ) === ':' ) {
|
|
// To convert the protocol into a case-insensitive Lua pattern,
|
|
// we need to replace letters with a character class like [Xx]
|
|
// and insert a '%' before various punctuation.
|
|
$prot = preg_replace_callback( '/([a-zA-Z])|([()^$%.\[\]*+?-])/', function ( $m ) {
|
|
if ( $m[1] ) {
|
|
return '[' . strtoupper( $m[1] ) . strtolower( $m[1] ) . ']';
|
|
} else {
|
|
return '%' . $m[2];
|
|
}
|
|
}, substr( $prot, 0, -1 ) );
|
|
$opts['nowiki_protocols']["($prot):"] = '%1:';
|
|
}
|
|
}
|
|
|
|
return $this->getEngine()->registerInterface( 'mw.text.lua', $lib, $opts );
|
|
}
|
|
|
|
function textUnstrip( $s ) {
|
|
$this->checkType( 'unstrip', 1, $s, 'string' );
|
|
$stripState = $this->getParser()->mStripState;
|
|
return array( $stripState->killMarkers( $stripState->unstripNoWiki( $s ) ) );
|
|
}
|
|
|
|
function textUnstripNoWiki( $s ) {
|
|
$this->checkType( 'unstripNoWiki', 1, $s, 'string' );
|
|
return array( $this->getParser()->mStripState->unstripNoWiki( $s ) );
|
|
}
|
|
|
|
function killMarkers( $s ) {
|
|
$this->checkType( 'killMarkers', 1, $s, 'string' );
|
|
return array( $this->getParser()->mStripState->killMarkers( $s ) );
|
|
}
|
|
|
|
function getEntityTable() {
|
|
$flags = ENT_QUOTES;
|
|
// PHP 5.3 compat
|
|
if ( defined( "ENT_HTML5" ) ) {
|
|
$flags |= constant( "ENT_HTML5" );
|
|
}
|
|
$table = array_flip( get_html_translation_table( HTML_ENTITIES, $flags, "UTF-8" ) );
|
|
return array( $table );
|
|
}
|
|
}
|