mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/RevisionSlider
synced 2024-11-15 11:40:43 +00:00
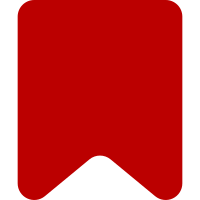
This patch mainly reintroduces the option to click on bars and move the pointers with it. To do this, 'ghost' pointers are introduced to show what would happen when bars are clicked. The pointers moved differ depending on where the user clicks on a bar. Pointers are still not allowed to change positions, so in some cases booth pointers move with one click. See the task description. The patch also includes some renaming and also refactoring of the click handling in general. Furthermore bar hover mechanics are handled by the RevisionListView class now. Moving both pointers is not possible when it would push one of them of limits. Bug: T172092, T173566 Change-Id: I32a8256f7667e03081324d54accdf03a17454faf
122 lines
2.8 KiB
JavaScript
122 lines
2.8 KiB
JavaScript
( function ( mw, $ ) {
|
|
/**
|
|
* Module handling the slider logic of the RevisionSlider
|
|
*
|
|
* @param {RevisionList} revisions
|
|
* @constructor
|
|
*/
|
|
var Slider = function ( revisions ) {
|
|
this.revisions = revisions;
|
|
this.view = new mw.libs.revisionSlider.SliderView( this );
|
|
};
|
|
|
|
$.extend( Slider.prototype, {
|
|
/**
|
|
* @type {RevisionList}
|
|
*/
|
|
revisions: null,
|
|
|
|
/**
|
|
* @type {number}
|
|
*/
|
|
oldestVisibleRevisionIndex: 0,
|
|
|
|
/**
|
|
* @type {number}
|
|
*/
|
|
revisionsPerWindow: 0,
|
|
|
|
/**
|
|
* @type {SliderView}
|
|
*/
|
|
view: null,
|
|
|
|
/**
|
|
* @return {RevisionList}
|
|
*/
|
|
getRevisionList: function () {
|
|
return this.revisions;
|
|
},
|
|
|
|
/**
|
|
* @return {SliderView}
|
|
*/
|
|
getView: function () {
|
|
return this.view;
|
|
},
|
|
|
|
/**
|
|
* Sets the number of revisions that are visible at once (depending on browser window size)
|
|
*
|
|
* @param {number} n
|
|
*/
|
|
setRevisionsPerWindow: function ( n ) {
|
|
this.revisionsPerWindow = n;
|
|
},
|
|
|
|
/**
|
|
* @return {number}
|
|
*/
|
|
getRevisionsPerWindow: function () {
|
|
return this.revisionsPerWindow;
|
|
},
|
|
|
|
/**
|
|
* Returns the index of the oldest revision that is visible in the current window
|
|
*
|
|
* @return {number}
|
|
*/
|
|
getOldestVisibleRevisionIndex: function () {
|
|
return this.oldestVisibleRevisionIndex;
|
|
},
|
|
|
|
/**
|
|
* Returns the index of the newest revision that is visible in the current window
|
|
*
|
|
* @return {number}
|
|
*/
|
|
getNewestVisibleRevisionIndex: function () {
|
|
return this.oldestVisibleRevisionIndex + this.revisionsPerWindow - 1;
|
|
},
|
|
|
|
/**
|
|
* @return {boolean}
|
|
*/
|
|
isAtStart: function () {
|
|
return this.getOldestVisibleRevisionIndex() === 0 || this.revisions.getLength() <= this.revisionsPerWindow;
|
|
},
|
|
|
|
/**
|
|
* @return {boolean}
|
|
*/
|
|
isAtEnd: function () {
|
|
return this.getNewestVisibleRevisionIndex() === this.revisions.getLength() - 1 || this.revisions.getLength() <= this.revisionsPerWindow;
|
|
},
|
|
|
|
/**
|
|
* Sets the index of the first revision that is visible in the current window
|
|
*
|
|
* @param {number} value
|
|
*/
|
|
setFirstVisibleRevisionIndex: function ( value ) {
|
|
this.oldestVisibleRevisionIndex = value;
|
|
},
|
|
|
|
/**
|
|
* Sets the new oldestVisibleRevisionIndex after sliding in a direction
|
|
*
|
|
* @param {number} direction - Either -1, 0 or 1
|
|
*/
|
|
slide: function ( direction ) {
|
|
var highestPossibleFirstRev = this.revisions.getLength() - this.revisionsPerWindow;
|
|
|
|
this.oldestVisibleRevisionIndex += direction * this.revisionsPerWindow;
|
|
this.oldestVisibleRevisionIndex = Math.min( this.oldestVisibleRevisionIndex, highestPossibleFirstRev );
|
|
this.oldestVisibleRevisionIndex = Math.max( 0, this.oldestVisibleRevisionIndex );
|
|
}
|
|
} );
|
|
|
|
mw.libs.revisionSlider = mw.libs.revisionSlider || {};
|
|
mw.libs.revisionSlider.Slider = Slider;
|
|
}( mediaWiki, jQuery ) );
|