mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/RevisionSlider
synced 2024-11-16 03:53:18 +00:00
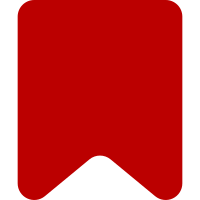
It's never used in another context but together with the ….Slider module. Motivated by the confusion about the two types of require() introduced in Idf1cc79. Bug: T233279 Change-Id: I922d7ab56fd3ce80bc901f1a5d7174df6fe6756d
139 lines
3.3 KiB
JavaScript
139 lines
3.3 KiB
JavaScript
var SliderView = require( './ext.RevisionSlider.SliderView.js' );
|
|
|
|
/**
|
|
* Module handling the slider logic of the RevisionSlider
|
|
*
|
|
* @class Slider
|
|
* @param {RevisionList} revisions
|
|
* @constructor
|
|
*/
|
|
function Slider( revisions ) {
|
|
this.revisions = revisions;
|
|
this.view = new SliderView( this );
|
|
}
|
|
|
|
$.extend( Slider.prototype, {
|
|
/**
|
|
* @type {RevisionList}
|
|
*/
|
|
revisions: null,
|
|
|
|
/**
|
|
* @type {number}
|
|
*/
|
|
oldestVisibleRevisionIndex: 0,
|
|
|
|
/**
|
|
* @type {number}
|
|
*/
|
|
revisionsPerWindow: 0,
|
|
|
|
/**
|
|
* @type {SliderView}
|
|
*/
|
|
view: null,
|
|
|
|
/**
|
|
* @return {RevisionList}
|
|
*/
|
|
getRevisionList: function () {
|
|
return this.revisions;
|
|
},
|
|
|
|
/**
|
|
* @return {SliderView}
|
|
*/
|
|
getView: function () {
|
|
return this.view;
|
|
},
|
|
|
|
/**
|
|
* Sets the number of revisions that are visible at once (depending on browser window size)
|
|
*
|
|
* @param {number} n
|
|
*/
|
|
setRevisionsPerWindow: function ( n ) {
|
|
this.revisionsPerWindow = n;
|
|
},
|
|
|
|
/**
|
|
* @return {number}
|
|
*/
|
|
getRevisionsPerWindow: function () {
|
|
return this.revisionsPerWindow;
|
|
},
|
|
|
|
/**
|
|
* Returns the index of the oldest revision that is visible in the current window
|
|
*
|
|
* @return {number}
|
|
*/
|
|
getOldestVisibleRevisionIndex: function () {
|
|
return this.oldestVisibleRevisionIndex;
|
|
},
|
|
|
|
/**
|
|
* Returns the index of the newest revision that is visible in the current window
|
|
*
|
|
* @return {number}
|
|
*/
|
|
getNewestVisibleRevisionIndex: function () {
|
|
return this.oldestVisibleRevisionIndex + this.revisionsPerWindow - 1;
|
|
},
|
|
|
|
/**
|
|
* @return {boolean}
|
|
*/
|
|
isAtStart: function () {
|
|
return this.getOldestVisibleRevisionIndex() === 0 || this.revisions.getLength() <= this.revisionsPerWindow;
|
|
},
|
|
|
|
/**
|
|
* @return {boolean}
|
|
*/
|
|
isAtEnd: function () {
|
|
return this.getNewestVisibleRevisionIndex() === this.revisions.getLength() - 1 || this.revisions.getLength() <= this.revisionsPerWindow;
|
|
},
|
|
|
|
/**
|
|
* Sets the index of the first revision that is visible in the current window
|
|
*
|
|
* @param {number} value
|
|
*/
|
|
setFirstVisibleRevisionIndex: function ( value ) {
|
|
this.oldestVisibleRevisionIndex = value;
|
|
},
|
|
|
|
/**
|
|
* Sets the new oldestVisibleRevisionIndex after sliding in a direction
|
|
*
|
|
* @param {number} direction - Either -1, 0 or 1
|
|
*/
|
|
slide: function ( direction ) {
|
|
var highestPossibleFirstRev = this.revisions.getLength() - this.revisionsPerWindow;
|
|
|
|
this.oldestVisibleRevisionIndex += direction * this.revisionsPerWindow;
|
|
this.oldestVisibleRevisionIndex = Math.min( this.oldestVisibleRevisionIndex, highestPossibleFirstRev );
|
|
this.oldestVisibleRevisionIndex = Math.max( 0, this.oldestVisibleRevisionIndex );
|
|
}
|
|
} );
|
|
|
|
module.exports = {
|
|
Api: require( './ext.RevisionSlider.Api.js' ),
|
|
DiffPage: require( './ext.RevisionSlider.DiffPage.js' ),
|
|
HelpDialog: require( './ext.RevisionSlider.HelpDialog.js' ),
|
|
makeRevisions: require( './ext.RevisionSlider.RevisionList.js' ).makeRevisions,
|
|
Revision: require( './ext.RevisionSlider.Revision.js' ).Revision,
|
|
RevisionList: require( './ext.RevisionSlider.RevisionList.js' ).RevisionList,
|
|
RevisionListView: require( './ext.RevisionSlider.RevisionListView.js' ),
|
|
setUserOffset: require( './ext.RevisionSlider.Revision.js' ).setUserOffset,
|
|
Slider: Slider,
|
|
SliderView: SliderView,
|
|
utils: require( './ext.RevisionSlider.util.js' ),
|
|
|
|
private: {
|
|
Pointer: require( './ext.RevisionSlider.Pointer.js' ),
|
|
PointerView: require( './ext.RevisionSlider.PointerView.js' )
|
|
}
|
|
};
|