mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/RevisionSlider
synced 2024-11-13 18:27:03 +00:00
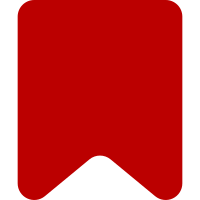
* eslint-config-wikimedia: 0.28.0 → 0.28.2 * grunt-stylelint: 0.20.0 → 0.20.1 * stylelint-config-wikimedia: 0.17.1 → 0.17.2 Change-Id: I798f739b5b2f12e5c6eedc88ecac557de28be129
109 lines
2.2 KiB
JavaScript
109 lines
2.2 KiB
JavaScript
/**
|
|
* @class Settings
|
|
* @constructor
|
|
*/
|
|
function Settings() {
|
|
this.hideHelpDialogue = this.loadBoolean( 'hide-help-dialogue' );
|
|
this.autoExpand = this.loadBoolean( 'autoexpand' );
|
|
}
|
|
|
|
Object.assign( Settings.prototype, {
|
|
/**
|
|
* @type {boolean}
|
|
*/
|
|
hideHelpDialogue: null,
|
|
|
|
/**
|
|
* @type {boolean}
|
|
*/
|
|
autoExpand: null,
|
|
|
|
/**
|
|
* @return {boolean}
|
|
*/
|
|
shouldHideHelpDialogue: function () {
|
|
return this.hideHelpDialogue;
|
|
},
|
|
|
|
/**
|
|
* @return {boolean}
|
|
*/
|
|
shouldAutoExpand: function () {
|
|
return this.autoExpand;
|
|
},
|
|
|
|
/**
|
|
* @param {boolean} newSetting
|
|
*/
|
|
setHideHelpDialogue: function ( newSetting ) {
|
|
if ( newSetting !== this.hideHelpDialogue ) {
|
|
this.saveBoolean( 'hide-help-dialogue', newSetting );
|
|
this.hideHelpDialogue = newSetting;
|
|
}
|
|
},
|
|
|
|
/**
|
|
* @param {boolean} newSetting
|
|
*/
|
|
setAutoExpand: function ( newSetting ) {
|
|
if ( newSetting !== this.autoExpand ) {
|
|
this.saveBoolean( 'autoexpand', newSetting );
|
|
this.autoExpand = newSetting;
|
|
}
|
|
},
|
|
|
|
/**
|
|
* @private
|
|
* @param {string} name
|
|
* @param {string} defaultValue
|
|
* @return {string|boolean}
|
|
*/
|
|
loadSetting: function ( name, defaultValue ) {
|
|
let setting;
|
|
if ( mw.user.isNamed() ) {
|
|
setting = mw.user.options.get( 'userjs-revslider-' + name );
|
|
} else {
|
|
setting = mw.storage.get( 'mw-revslider-' + name ) ||
|
|
mw.cookie.get( '-revslider-' + name );
|
|
}
|
|
|
|
return setting !== null && setting !== false ? setting : defaultValue;
|
|
},
|
|
|
|
/**
|
|
* @private
|
|
* @param {string} name
|
|
* @param {boolean} [defaultValue]
|
|
* @return {boolean}
|
|
*/
|
|
loadBoolean: function ( name, defaultValue ) {
|
|
return this.loadSetting( name, defaultValue ? '1' : '0' ) === '1';
|
|
},
|
|
|
|
/**
|
|
* @private
|
|
* @param {string} name
|
|
* @param {string} value
|
|
*/
|
|
saveSetting: function ( name, value ) {
|
|
if ( mw.user.isNamed() ) {
|
|
( new mw.Api() ).saveOption( 'userjs-revslider-' + name, value );
|
|
} else {
|
|
if ( !mw.storage.set( 'mw-revslider-' + name, value ) ) {
|
|
mw.cookie.set( '-revslider-' + name, value ); // use cookie when localStorage is not available
|
|
}
|
|
}
|
|
},
|
|
|
|
/**
|
|
* @private
|
|
* @param {string} name
|
|
* @param {boolean} value
|
|
*/
|
|
saveBoolean: function ( name, value ) {
|
|
this.saveSetting( name, value ? '1' : '0' );
|
|
}
|
|
} );
|
|
|
|
module.exports = Settings;
|