mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/RelatedArticles
synced 2024-12-23 21:33:11 +00:00
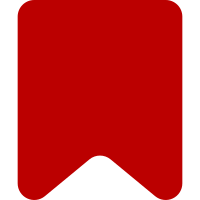
1) Merge ext.relatedArticles.readMore and ext.relatedArticles.cards modules These are always loaded together. No need to have them in separate modules. In process switch to packagefiles 2) Merge ext.relatedArticles.readMore.bootstrap and ext.relatedArticles.readMore.gateway Always loaded together. Bug: T306228 Change-Id: I9442b0336e22ca795cc06f76068215266fe81271
55 lines
1.4 KiB
JavaScript
55 lines
1.4 KiB
JavaScript
'use strict';
|
|
|
|
/**
|
|
* Model for an article
|
|
* It is the single source of truth about a Card, which is a representation
|
|
* of a wiki article. It emits a 'change' event when its attribute changes.
|
|
* A View can listen to this event and update the UI accordingly.
|
|
*
|
|
* @class mw.cards.CardModel
|
|
* @extends OO.EventEmitter
|
|
* @param {Object} attributes article data, such as title, url, etc. about
|
|
* an article
|
|
*/
|
|
function CardModel( attributes ) {
|
|
CardModel.super.apply( this, arguments );
|
|
/**
|
|
* @property {Object} attributes of the model
|
|
*/
|
|
this.attributes = attributes;
|
|
}
|
|
OO.inheritClass( CardModel, OO.EventEmitter );
|
|
|
|
/**
|
|
* Set a model attribute.
|
|
* Emits a 'change' event with the object whose key is the attribute
|
|
* that's being updated and value is the value that's being set. The event
|
|
* can also be silenced.
|
|
*
|
|
* @param {string} key attribute that's being set
|
|
* @param {Mixed} value the value of the key param
|
|
* @param {boolean} [silent] whether to emit the 'change' event. By default
|
|
* the 'change' event will be emitted.
|
|
*/
|
|
CardModel.prototype.set = function ( key, value, silent ) {
|
|
var event = {};
|
|
|
|
this.attributes[ key ] = value;
|
|
if ( !silent ) {
|
|
event[ key ] = value;
|
|
this.emit( 'change', event );
|
|
}
|
|
};
|
|
|
|
/**
|
|
* Get the model attribute's value.
|
|
*
|
|
* @param {string} key attribute that's being looked up
|
|
* @return {Mixed}
|
|
*/
|
|
CardModel.prototype.get = function ( key ) {
|
|
return this.attributes[ key ];
|
|
};
|
|
|
|
module.exports = CardModel;
|