mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/RelatedArticles
synced 2024-11-14 19:31:34 +00:00
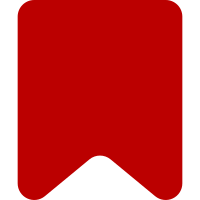
The ParserOutput object is already reset when state is reset, so there's no point in setting or unsetting properties on it. And since the only unit test was deleted, remove the hook for that too. Change-Id: Idf12365e8c4b14e527d923edc1086bdaf349df32
105 lines
2.7 KiB
PHP
105 lines
2.7 KiB
PHP
<?php
|
|
|
|
namespace RelatedArticles;
|
|
|
|
use Parser;
|
|
use OutputPage;
|
|
use ParserOutput;
|
|
|
|
class Hooks {
|
|
|
|
/**
|
|
* Handler for the <code>ParserFirstCallInit</code> hook.
|
|
*
|
|
* Registers the <code>related</code> parser function (see
|
|
* {@see Hooks::onFuncRelated}).
|
|
*
|
|
* @param Parser $parser
|
|
* @return boolean Always <code>true</code>
|
|
*/
|
|
public static function onParserFirstCallInit( Parser &$parser ) {
|
|
$parser->setFunctionHook( 'related', 'RelatedArticles\\Hooks::onFuncRelated' );
|
|
|
|
return true;
|
|
}
|
|
|
|
/**
|
|
* The <code>related</code> parser function.
|
|
*
|
|
* Appends the arguments to the internal list so that it can be used
|
|
* more that once per page.
|
|
* We don't use setProperty here is there is no need
|
|
* to store it as a page prop in the database, only in the cache.
|
|
*
|
|
* @todo Test for uniqueness
|
|
* @param Parser $parser
|
|
*
|
|
* @return string Always <code>''</code>
|
|
*/
|
|
public static function onFuncRelated( Parser $parser ) {
|
|
$parserOutput = $parser->getOutput();
|
|
$relatedPages = $parserOutput->getExtensionData( 'RelatedArticles' );
|
|
if ( !$relatedPages ) {
|
|
$relatedPages = [];
|
|
}
|
|
$args = func_get_args();
|
|
array_shift( $args );
|
|
|
|
// Add all the related pages passed by the parser function
|
|
// {{#related:Test with read more|Foo|Bar}}
|
|
foreach ( $args as $relatedPage ) {
|
|
$relatedPages[] = $relatedPage;
|
|
}
|
|
$parserOutput->setExtensionData( 'RelatedArticles', $relatedPages );
|
|
|
|
return '';
|
|
}
|
|
|
|
/**
|
|
* Passes the related pages list from the cached parser output
|
|
* object to the output page for rendering.
|
|
*
|
|
* The list of related pages will be retrieved using
|
|
* <code>ParserOutput#getExtensionData</code>.
|
|
*
|
|
* @param OutputPage $out
|
|
* @param ParserOutput $parserOutput
|
|
* @return boolean Always <code>true</code>
|
|
*/
|
|
public static function onOutputPageParserOutput( OutputPage &$out, ParserOutput $parserOutput ) {
|
|
$related = $parserOutput->getExtensionData( 'RelatedArticles' );
|
|
|
|
if ( $related ) {
|
|
$out->setProperty( 'RelatedArticles', $related );
|
|
}
|
|
|
|
return true;
|
|
}
|
|
|
|
/**
|
|
* Register QUnit tests.
|
|
* @see https://www.mediawiki.org/wiki/Manual:Hooks/ResourceLoaderTestModules
|
|
*
|
|
* @param array $modules
|
|
* @param ResourceLoader $rl
|
|
* @return bool
|
|
*/
|
|
public static function onResourceLoaderTestModules( &$modules, &$rl ) {
|
|
$boilerplate = [
|
|
'localBasePath' => __DIR__ . '/../tests/qunit/',
|
|
'remoteExtPath' => 'RelatedArticles/tests/qunit',
|
|
'targets' => [ 'desktop', 'mobile' ],
|
|
];
|
|
|
|
$modules['qunit']['ext.relatedArticles.readMore.gateway.tests'] = $boilerplate + [
|
|
'scripts' => [
|
|
'ext.relatedArticles.readMore.gateway/test_RelatedPagesGateway.js',
|
|
],
|
|
'dependencies' => [
|
|
'ext.relatedArticles.readMore.gateway',
|
|
],
|
|
];
|
|
return true;
|
|
}
|
|
}
|