mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Popups
synced 2024-11-13 17:56:55 +00:00
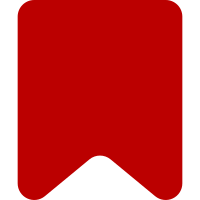
Why: Because they are the approved standard by TC39 and Ecma for JavaScript modules. Changes: * Wrap mw-node-qunit in run.js to register babel to transpile modules for node v6 * Change all sources in src/ to use ES modules * Change constants.js to be able to run without jQuery.bracketedDevicePixelRatio given ES modules are hoisted to the top by spec so we can't patch globals before importing it * Change all tests in tests/node-qunit/ to use ES modules * Drop usage of mock-require given ES modules are easy to stub with sinon Additional changes: * Rename tests/node-qunit/renderer.js to renderer.test.js to follow the convention of all the other files * Make npm run test:node run only .test.js test files so that it doesn't run the stubs.js or run.js file. Bug: T171951 Change-Id: I17a0b76041d5e2fd18e2d54950d9d7c0db99a941
68 lines
1.5 KiB
JavaScript
68 lines
1.5 KiB
JavaScript
/**
|
|
* Creates a **minimal** stub that can be used in place of an `mw.User`
|
|
* instance.
|
|
*
|
|
* @param {boolean} isAnon The return value of the `#isAnon`.
|
|
* @return {Object}
|
|
*/
|
|
export function createStubUser( isAnon ) {
|
|
return {
|
|
isAnon: function () {
|
|
return isAnon;
|
|
},
|
|
sessionId: function () {
|
|
return '0123456789';
|
|
}
|
|
};
|
|
}
|
|
|
|
/**
|
|
* Creates a **minimal** stub that can be used in place of an `mw.Map`
|
|
* instance.
|
|
*
|
|
* @return {mw.Map}
|
|
*/
|
|
export function createStubMap() {
|
|
var m = new Map(); /* global Map */
|
|
m.get = function ( key, fallback ) {
|
|
fallback = arguments.length > 1 ? fallback : null;
|
|
if ( typeof key === 'string' ) {
|
|
return m.has( key ) ? Map.prototype.get.call( m, key ) : fallback;
|
|
}
|
|
// Invalid selection key
|
|
return null;
|
|
};
|
|
return m;
|
|
}
|
|
|
|
/**
|
|
* Creates a stub that can be used as a replacement to mw.experiements
|
|
* @param {bool} isSampled If `true` then the `getBucket` method will
|
|
* return 'A' otherwise 'control'.
|
|
* @return {object}
|
|
*/
|
|
export function createStubExperiments( isSampled ) {
|
|
return {
|
|
getBucket: function () {
|
|
return isSampled ? 'A' : 'control';
|
|
}
|
|
};
|
|
}
|
|
|
|
/**
|
|
* Creates a **minimal** stub that can be used in place of an instance of
|
|
* `mw.Title`.
|
|
*
|
|
* @param {Number} namespace
|
|
* @param {String} prefixedDb, e.g. Foo, or File:Bar.jpg
|
|
* @return {Object}
|
|
*/
|
|
export function createStubTitle( namespace, prefixedDb ) {
|
|
return {
|
|
namespace: namespace,
|
|
getPrefixedDb: function () {
|
|
return prefixedDb;
|
|
}
|
|
};
|
|
}
|