mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Popups
synced 2024-11-13 17:56:55 +00:00
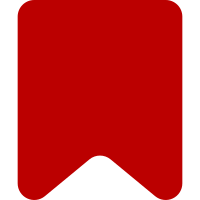
Why: Because they are the approved standard by TC39 and Ecma for JavaScript modules. Changes: * Wrap mw-node-qunit in run.js to register babel to transpile modules for node v6 * Change all sources in src/ to use ES modules * Change constants.js to be able to run without jQuery.bracketedDevicePixelRatio given ES modules are hoisted to the top by spec so we can't patch globals before importing it * Change all tests in tests/node-qunit/ to use ES modules * Drop usage of mock-require given ES modules are easy to stub with sinon Additional changes: * Rename tests/node-qunit/renderer.js to renderer.test.js to follow the convention of all the other files * Make npm run test:node run only .test.js test files so that it doesn't run the stubs.js or run.js file. Bug: T171951 Change-Id: I17a0b76041d5e2fd18e2d54950d9d7c0db99a941
54 lines
1.1 KiB
JavaScript
54 lines
1.1 KiB
JavaScript
import createContainer from '../../src/container';
|
|
|
|
QUnit.module( 'container', {
|
|
beforeEach: function () {
|
|
this.container = createContainer();
|
|
this.factory = this.sandbox.stub();
|
|
}
|
|
} );
|
|
|
|
QUnit.test( '#has', function ( assert ) {
|
|
this.container.set( 'foo', this.factory );
|
|
|
|
assert.ok( this.container.has( 'foo' ) );
|
|
} );
|
|
|
|
QUnit.test( '#get', function ( assert ) {
|
|
var service = {},
|
|
that = this;
|
|
|
|
this.factory.returns( service );
|
|
|
|
this.container.set( 'foo', this.factory );
|
|
|
|
assert.strictEqual( service, this.container.get( 'foo' ) );
|
|
assert.strictEqual(
|
|
this.container,
|
|
this.factory.getCall( 0 ).args[ 0 ]
|
|
);
|
|
|
|
// ---
|
|
|
|
this.container.get( 'foo' );
|
|
|
|
assert.ok(
|
|
this.factory.calledOnce,
|
|
'It should memoize the result of the factory.'
|
|
);
|
|
|
|
// ---
|
|
|
|
assert.throws(
|
|
function () {
|
|
that.container.get( 'bar' );
|
|
},
|
|
/The service "bar" hasn't been defined./
|
|
);
|
|
} );
|
|
|
|
QUnit.test( '#get should handle values, not just functions', function ( assert ) {
|
|
this.container.set( 'foo', 'bar' );
|
|
|
|
assert.strictEqual( 'bar', this.container.get( 'foo' ) );
|
|
} );
|