mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Popups
synced 2024-11-15 19:50:04 +00:00
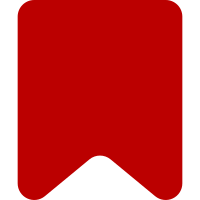
This patch also removes misplaced empty lines at the beginning of a scope. In PHP code we even have a sniff for these. In JavaScript we don't, but I suggest to be consistent about this. Change-Id: Ic104ae8fe176da1dafa9bc783402adecb71de1f0
93 lines
1.8 KiB
JavaScript
93 lines
1.8 KiB
JavaScript
/**
|
|
* @module container
|
|
*/
|
|
|
|
/**
|
|
* Creates an empty service container.
|
|
*
|
|
* @return {Container}
|
|
*/
|
|
export default function createContainer() {
|
|
const factories = {},
|
|
cache = {};
|
|
|
|
/**
|
|
* The interface implemented by all service containers.
|
|
*
|
|
* @interface Container
|
|
* @global
|
|
*/
|
|
return {
|
|
/**
|
|
* Defines a service.
|
|
*
|
|
* @function
|
|
* @name Container#set
|
|
* @param {string} name
|
|
* @param {*} factory
|
|
* @return {void}
|
|
*/
|
|
set( name, factory ) {
|
|
factories[ name ] = factory;
|
|
},
|
|
|
|
/**
|
|
* Gets whether a service has been defined.
|
|
*
|
|
* @function
|
|
* @name Container#has
|
|
* @param {string} name
|
|
* @return {boolean} `true` if the service has been defined; otherwise,
|
|
* `false`
|
|
*/
|
|
has( name ) {
|
|
return factories.hasOwnProperty( name );
|
|
},
|
|
|
|
/**
|
|
* Gets a service.
|
|
*
|
|
* If the service was defined with a factory function, then the factory
|
|
* function is called and the result is returned. For performance reasons,
|
|
* the result of calling the factory function is cached.
|
|
*
|
|
* If the service was defined with a value, then the value is returned.
|
|
*
|
|
* @example
|
|
* var container = createContainer();
|
|
*
|
|
* container.set( 'foo', true );
|
|
* container.set( 'baz', ( c ) => {
|
|
* if ( c.get( 'foo' ) ) {
|
|
* return 'qux';
|
|
* }
|
|
*
|
|
* return 'quux';
|
|
* } );
|
|
*
|
|
* @function
|
|
* @name Container#get
|
|
* @param {string} name
|
|
* @return {*}
|
|
* @throws Error If the service hasn't been defined
|
|
*/
|
|
get( name ) {
|
|
if ( !this.has( name ) ) {
|
|
throw new Error( `The service "${ name }" hasn't been defined.` );
|
|
}
|
|
|
|
const factory = factories[ name ];
|
|
|
|
if ( typeof factory !== 'function' ) {
|
|
return factory;
|
|
}
|
|
|
|
if ( !cache.hasOwnProperty( name ) ) {
|
|
cache[ name ] = factories[ name ]( this );
|
|
}
|
|
|
|
return cache[ name ];
|
|
}
|
|
};
|
|
}
|