mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Popups
synced 2024-11-12 01:08:39 +00:00
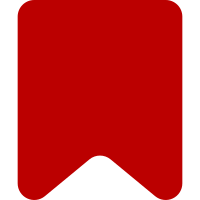
The schema introduces a new field called `hovercardsSuppressedByGadget`, which is `true` when the Navigation Popups gadget, rather than the Popups extensions, is being used to fetch and display article summary. Note that it may take some time before the Navigation Popups gadget is loaded onto the page, thus actions taking place before this happens will record the value of `hovercardsSuppressedByGadget` as `false`. This is mostly the case with the `pageLoaded` action which almost always happens before the gadget code is loaded. Bug: T137203 Change-Id: Ie31deea7ae2323d6a346c67ed84fdf587ad55bd1
112 lines
2.9 KiB
JavaScript
112 lines
2.9 KiB
JavaScript
( function ( $, mw ) {
|
|
/**
|
|
* Return data that will be logged with each EL request
|
|
*
|
|
* @return {Object}
|
|
*/
|
|
function getDefaultValues() {
|
|
var defaults = {
|
|
pageTitleSource: mw.config.get( 'wgTitle' ),
|
|
namespaceIdSource: mw.config.get( 'wgNamespaceNumber' ),
|
|
pageIdSource: mw.config.get( 'wgArticleId' ),
|
|
isAnon: mw.user.isAnon(),
|
|
hovercardsSuppressedByGadget: false,
|
|
popupEnabled: mw.popups.getEnabledState(),
|
|
popupDelay: mw.popups.render.POPUP_DELAY,
|
|
pageToken: mw.user.generateRandomSessionId() +
|
|
Math.floor( mw.now() ).toString() +
|
|
mw.user.generateRandomSessionId(),
|
|
sessionToken: mw.user.sessionId(),
|
|
// arbitrary name that represents the current UI of the popups
|
|
version: 'legacy',
|
|
// current API version
|
|
api: 'mwapi'
|
|
};
|
|
|
|
// Include edit count bucket to the list of default values if the user is logged in.
|
|
if ( !mw.user.isAnon() ) {
|
|
defaults.editCountBucket = mw.popups.schemaPopups.getEditCountBucket(
|
|
mw.config.get( 'wgUserEditCount' ) );
|
|
}
|
|
|
|
return defaults;
|
|
}
|
|
|
|
/**
|
|
* Return the sampling rate for the Schema:Popups
|
|
*
|
|
* User's session ID is used to determine the eligibility for logging,
|
|
* thus the function will result the same outcome as long as the browser
|
|
* hasn't been restarted or the cookie hasn't been cleared.
|
|
*
|
|
* @return {number}
|
|
*/
|
|
function getSamplingRate() {
|
|
var bucket,
|
|
samplingRate = mw.config.get( 'wgPopupsSchemaPopupsSamplingRate', 0 );
|
|
|
|
if ( !$.isFunction( navigator.sendBeacon ) ) {
|
|
return 0;
|
|
}
|
|
|
|
bucket = mw.experiments.getBucket( {
|
|
name: 'ext.popups.schemaPopus',
|
|
enabled: true,
|
|
buckets: {
|
|
control: 1 - samplingRate,
|
|
A: samplingRate
|
|
}
|
|
}, mw.user.sessionId() );
|
|
|
|
return bucket === 'A' ? 1 : 0;
|
|
}
|
|
|
|
/**
|
|
* Return edit count bucket based on the number of edits
|
|
*
|
|
* @param {number} editCount
|
|
* @return {string}
|
|
*/
|
|
function getEditCountBucket( editCount ) {
|
|
var bucket;
|
|
|
|
if ( editCount === 0 ) {
|
|
bucket = '0';
|
|
} else if ( editCount >= 1 && editCount <= 4 ) {
|
|
bucket = '1-4';
|
|
} else if ( editCount >= 5 && editCount <= 99 ) {
|
|
bucket = '5-99';
|
|
} else if ( editCount >= 100 && editCount <= 999 ) {
|
|
bucket = '100-999';
|
|
} else if ( editCount >= 1000 ) {
|
|
bucket = '1000+';
|
|
}
|
|
|
|
return bucket + ' edits';
|
|
}
|
|
|
|
/**
|
|
* Return data after making some adjustments so that it's ready to be logged
|
|
*
|
|
* @param {Object}data
|
|
* @return {Object}
|
|
*/
|
|
function getMassagedData( data ) {
|
|
data.previewCountBucket = mw.popups.getPreviewCountBucket();
|
|
delete data.dwellStartTime;
|
|
// Figure out `namespaceIdHover` from `pageTitleHover`.
|
|
if ( data.pageTitleHover && data.namespaceIdHover === undefined ) {
|
|
data.namespaceIdHover = new mw.Title( data.pageTitleHover ).getNamespaceId();
|
|
}
|
|
return data;
|
|
}
|
|
|
|
mw.popups.schemaPopups = {
|
|
getDefaultValues: getDefaultValues,
|
|
getSamplingRate: getSamplingRate,
|
|
getEditCountBucket: getEditCountBucket,
|
|
getMassagedData: getMassagedData
|
|
};
|
|
|
|
} )( jQuery, mediaWiki );
|