mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Popups
synced 2024-11-28 01:10:04 +00:00
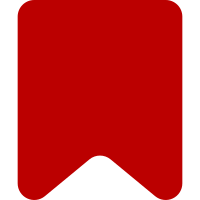
The following upgrade was made: eslint-config-wikimedia | 0.8.1 | 0.9.0 The upgrade of eslint-config-wikimedia removes the need of eslint-plugin-qunit as a peerDependency because it is now a hard dependency [1] so it was removed in our package.json. It appears the biggest change in the upgrade was the use of separate profiles [2]. Given this, our root .eslintrc.json was updated to extend from 'wikimedia/client'. Several test files were flagged by the upgraded linter and were fixed in this patch. Additionally, our build file was flagged for having too many statements on one line. This rule was turned off in .eslintrc.es5.json [1] https://github.com/wikimedia/eslint-config-wikimedia/blob/master/package.json#L48 [2] https://github.com/wikimedia/eslint-config-wikimedia/blob/master/CHANGELOG.md#090--2018-11-19 Bug: T209314 Change-Id: I29db72e77f04a327bc9c2b558c6d53849287bb80
111 lines
2.6 KiB
JavaScript
111 lines
2.6 KiB
JavaScript
import createPreviewBehavior from '../../src/previewBehavior';
|
|
import { createStubUser } from './stubs';
|
|
|
|
QUnit.module( 'ext.popups.preview.settingsBehavior', {
|
|
beforeEach() {
|
|
function newFromText( title ) {
|
|
return {
|
|
getUrl() { return `url/${ title }`; }
|
|
};
|
|
}
|
|
|
|
mediaWiki.Title = { newFromText };
|
|
/* global Map */ this.config = new Map();
|
|
},
|
|
afterEach() {
|
|
mediaWiki.Title = null;
|
|
}
|
|
} );
|
|
|
|
QUnit.test( 'it should set the settingsUrl', function ( assert ) {
|
|
const user = createStubUser( /* isAnon = */ false ),
|
|
actions = {};
|
|
|
|
const behavior = createPreviewBehavior( user, actions );
|
|
|
|
assert.deepEqual(
|
|
behavior.settingsUrl,
|
|
'url/Special:Preferences#mw-prefsection-rendering'
|
|
);
|
|
} );
|
|
|
|
QUnit.test( 'it shouldn\'t set the settingsUrl if the user is logged out', function ( assert ) {
|
|
const user = createStubUser( /* isAnon = */ true ),
|
|
actions = {},
|
|
behavior = createPreviewBehavior( user, actions );
|
|
|
|
assert.strictEqual(
|
|
behavior.settingsUrl,
|
|
undefined,
|
|
'No settings URL is set.'
|
|
);
|
|
} );
|
|
|
|
QUnit.test( 'it shouldn\'t set a showSettings handler if the user is logged in', function ( assert ) {
|
|
const user = createStubUser( /* isAnon = */ false ),
|
|
actions = {},
|
|
behavior = createPreviewBehavior( user, actions );
|
|
|
|
assert.strictEqual(
|
|
behavior.showSettings,
|
|
$.noop,
|
|
'No show settings handler is set.'
|
|
);
|
|
} );
|
|
|
|
QUnit.test( 'it should set a showSettings handler if the user is logged out', function ( assert ) {
|
|
const user = createStubUser( /* isAnon = */ true ),
|
|
event = {
|
|
preventDefault: this.sandbox.spy()
|
|
},
|
|
actions = {
|
|
showSettings: this.sandbox.spy()
|
|
},
|
|
behavior = createPreviewBehavior( user, actions );
|
|
|
|
behavior.showSettings( event );
|
|
|
|
assert.ok(
|
|
event.preventDefault.called,
|
|
'It should prevent the default action of the event.'
|
|
);
|
|
|
|
assert.ok(
|
|
actions.showSettings.called,
|
|
'It should dispatch the SETTINGS_SHOW action.'
|
|
);
|
|
} );
|
|
|
|
QUnit.test( 'it should mix in default actions', function ( assert ) {
|
|
const user = createStubUser( /* isAnon = */ true ),
|
|
actions = {};
|
|
|
|
actions.previewDwell = () => {};
|
|
actions.abandon = () => {};
|
|
actions.previewShow = () => {};
|
|
actions.linkClick = () => {};
|
|
|
|
const behavior = createPreviewBehavior( user, actions );
|
|
|
|
assert.strictEqual(
|
|
behavior.previewDwell,
|
|
actions.previewDwell,
|
|
'Preview dwelled action is mixed.'
|
|
);
|
|
assert.strictEqual(
|
|
behavior.previewAbandon,
|
|
actions.abandon,
|
|
'Preview action is mixed.'
|
|
);
|
|
assert.strictEqual(
|
|
behavior.previewShow,
|
|
actions.previewShow,
|
|
'Preview shown action is mixed.'
|
|
);
|
|
assert.strictEqual(
|
|
behavior.click,
|
|
actions.linkClick,
|
|
'Link click action is mixed.'
|
|
);
|
|
} );
|