mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Popups
synced 2024-11-15 19:50:04 +00:00
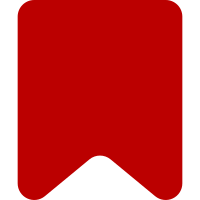
Functional changes: - Require page URL when constructing a PreviewModel null object. These models have valid titles and are used to display a preview when an extract is unobtainable. When presented with an empty URL, their linkage incorrectly pointed to the browser's current URL. Additional tests were added to verify the fix. - Check missing title in addition to falsy response in RESTBase gateway and update the test assertion to check title. It isn't clear if this can happen in the wild. - Forbid state mutation in the conclusion of MediaWikiGateway.getPageSummary() with a call to Deferred.promise(). This is consistent with the rest of repo including RESTBaseGateway. http://api.jquery.com/deferred.promise/ Nonfunctional changes: - Collapse two RESTBase gateway 404 tests into one as the scenarios and expectations were very similar. - Add failure HTTP status to 'MediaWiki API gateway handles API failure' test stub HTTP response for consistency with other cases. - Add nullity expectations to JSDocs touched and fix a couple typos throughout. - Make the gateway tests a little more consistent by collapsing Deferred variable usage where appropriate. This change is necessary to the completion of T183151 which uses the PreviewModel null objects for additional error cases. Bug: T183151 Change-Id: Ib77627fb9c80d8e806208bbafcfc615b130e3278
70 lines
1.5 KiB
JavaScript
70 lines
1.5 KiB
JavaScript
/**
|
|
* Creates a **minimal** stub that can be used in place of an `mw.User`
|
|
* instance.
|
|
*
|
|
* @param {boolean} isAnon The return value of the `#isAnon`.
|
|
* @return {Object}
|
|
*/
|
|
export function createStubUser( isAnon ) {
|
|
return {
|
|
isAnon: function () {
|
|
return isAnon;
|
|
},
|
|
sessionId: function () {
|
|
return '0123456789';
|
|
}
|
|
};
|
|
}
|
|
|
|
/**
|
|
* Creates a **minimal** stub that can be used in place of an `mw.Map`
|
|
* instance.
|
|
*
|
|
* @return {mw.Map}
|
|
*/
|
|
export function createStubMap() {
|
|
var m = new Map(); /* global Map */
|
|
m.get = function ( key, fallback ) {
|
|
fallback = arguments.length > 1 ? fallback : null;
|
|
if ( typeof key === 'string' ) {
|
|
return m.has( key ) ? Map.prototype.get.call( m, key ) : fallback;
|
|
}
|
|
// Invalid selection key
|
|
return null;
|
|
};
|
|
return m;
|
|
}
|
|
|
|
/**
|
|
* Creates a stub that can be used as a replacement to mw.experiements
|
|
* @param {string} bucket getBucket will respond with this bucket.
|
|
* @return {object}
|
|
*/
|
|
export function createStubExperiments( bucket ) {
|
|
return {
|
|
getBucket: function () {
|
|
return bucket;
|
|
}
|
|
};
|
|
}
|
|
|
|
/**
|
|
* Creates a **minimal** stub that can be used in place of an instance of
|
|
* `mw.Title`.
|
|
*
|
|
* @param {!Number} namespace
|
|
* @param {!String} prefixedDb, e.g. Foo, or File:Bar.jpg
|
|
* @return {!Object}
|
|
*/
|
|
export function createStubTitle( namespace, prefixedDb ) {
|
|
return {
|
|
namespace: namespace,
|
|
getPrefixedDb: function () {
|
|
return prefixedDb;
|
|
},
|
|
getUrl: function () {
|
|
return `/wiki/${prefixedDb}`;
|
|
}
|
|
};
|
|
}
|