mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Popups
synced 2024-11-15 03:34:03 +00:00
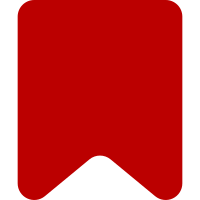
Changes: * Make the gateway handle missing pages, which are characterised by the MediaWiki API response having both the missing property set to truthy and the page not having any revisions. * Add the preview-empty template and associated "mwe-popups-is-empty" CSS class, which describe what an empty preview contains and how it should look. Supporting changes: * Move the original preview template into the ext.popups module. Bug: T151054 Change-Id: Ife75bf9c6bafdfe0a6cc3e20eea853b4ac8f951b
219 lines
5.8 KiB
JavaScript
219 lines
5.8 KiB
JavaScript
( function ( mw, $ ) {
|
|
|
|
/**
|
|
* @private
|
|
*
|
|
* @param {Object} data
|
|
*/
|
|
function createGateway( data ) {
|
|
var api = {
|
|
get: function () {
|
|
return $.Deferred()
|
|
.resolve( data )
|
|
.promise();
|
|
}
|
|
};
|
|
|
|
return mw.popups.createGateway( api );
|
|
}
|
|
|
|
/**
|
|
* @private
|
|
*
|
|
* @param {Object} page
|
|
*/
|
|
function createGatewayWithPage( page ) {
|
|
return createGateway( {
|
|
query: {
|
|
pages: [ page ]
|
|
}
|
|
} );
|
|
}
|
|
|
|
QUnit.module( 'ext.popups/gateway' );
|
|
|
|
QUnit.test( 'it should handle the API request failing', function ( assert ) {
|
|
var getDeferred = this.getDeferred = $.Deferred(),
|
|
api = {
|
|
get: function () {
|
|
return getDeferred.promise();
|
|
}
|
|
},
|
|
fetchPreview = mw.popups.createGateway( api ),
|
|
done = assert.async( 1 );
|
|
|
|
fetchPreview( 'Foo' ).fail( function () {
|
|
assert.ok( true );
|
|
|
|
done();
|
|
} );
|
|
|
|
getDeferred.reject();
|
|
} );
|
|
|
|
QUnit.test( 'it should fail if the response is empty', function ( assert ) {
|
|
var cases,
|
|
done;
|
|
|
|
cases = [
|
|
{},
|
|
{
|
|
query: {}
|
|
},
|
|
{
|
|
query: {
|
|
pages: []
|
|
}
|
|
}
|
|
];
|
|
|
|
done = assert.async( cases.length );
|
|
|
|
$.each( cases, function ( _, data ) {
|
|
var fetchPreview = createGateway( data );
|
|
|
|
fetchPreview( 'Foo' ).fail( function () {
|
|
assert.ok( true );
|
|
|
|
done();
|
|
} );
|
|
} );
|
|
} );
|
|
|
|
QUnit.test( 'it should handle an API response', function ( assert ) {
|
|
var done = assert.async( 1 ),
|
|
fetchPreview;
|
|
|
|
fetchPreview = createGateway( {
|
|
batchcomplete: true,
|
|
query: {
|
|
pages: [ {
|
|
contentmodel: 'wikitext',
|
|
extract: 'Richard Paul "Rick" Astley (/\u02c8r\u026ak \u02c8\u00e6stli/; born 6 February 1966) is an English singer, songwriter, musician, and radio personality. His 1987 song, "Never Gonna Give You Up" was a No. 1 hit single in 25 countries. By the time of his retirement in 1993, Astley had sold approximately 40 million records worldwide.\nAstley made a comeback in 2007, becoming an Internet phenomenon when his video "Never Gonna Give You Up" became integral to the meme known as "rickrolling". Astley was voted "Best Act Ever" by Internet users at the...',
|
|
lastrevid: 748725726,
|
|
length: 32132,
|
|
fullurl: 'https://en.wikipedia.org/wiki/Rick_Astley',
|
|
editurl: 'https://en.wikipedia.org/w/index.php?title=Rick_Astley&action=edit',
|
|
canonicalurl: 'https://en.wikipedia.org/wiki/Rick_Astley',
|
|
ns: 0,
|
|
pageid: 447541,
|
|
pagelanguage: 'en',
|
|
pagelanguagedir: 'ltr',
|
|
pagelanguagehtmlcode: 'en',
|
|
revisions: [ {
|
|
timestamp: '2016-11-10T00:14:14Z'
|
|
} ],
|
|
thumbnail: {
|
|
height: 300,
|
|
source: 'https://upload.wikimedia.org/wikipedia/commons/thumb/6/6d/Rick_Astley_-_Pepsifest_2009.jpg/200px-Rick_Astley_-_Pepsifest_2009.jpg',
|
|
width: 200
|
|
},
|
|
title: 'Rick Astley',
|
|
touched: '2016-11-10T00:14:14Z'
|
|
} ]
|
|
}
|
|
} );
|
|
|
|
fetchPreview( 'Rick Astley' ).done( function ( result ) {
|
|
assert.deepEqual( result, {
|
|
title: 'Rick Astley',
|
|
languageCode: 'en',
|
|
languageDirection: 'ltr',
|
|
url: 'https://en.wikipedia.org/wiki/Rick_Astley',
|
|
thumbnail: {
|
|
height: 300,
|
|
url: 'https://upload.wikimedia.org/wikipedia/commons/thumb/6/6d/Rick_Astley_-_Pepsifest_2009.jpg/200px-Rick_Astley_-_Pepsifest_2009.jpg',
|
|
width: 200
|
|
},
|
|
lastModified: new Date( '2016-11-10T00:14:14Z' ),
|
|
extract: 'Richard Paul "Rick" Astley is an English singer, songwriter, musician, and radio personality. His 1987 song, "Never Gonna Give You Up" was a No. 1 hit single in 25 countries. By the time of his retirement in 1993, Astley had sold approximately 40 million records worldwide.\nAstley made a comeback in 2007, becoming an Internet phenomenon when his video "Never Gonna Give You Up" became integral to the meme known as "rickrolling". Astley was voted "Best Act Ever" by Internet users at the',
|
|
isRecent: false
|
|
} );
|
|
|
|
done();
|
|
} );
|
|
} );
|
|
|
|
QUnit.test( 'it handles extracts', function ( assert ) {
|
|
var cases = [
|
|
// removeEllipsis
|
|
[ '', undefined ],
|
|
[ 'Extract...', 'Extract' ],
|
|
[ 'Extract.', 'Extract.' ],
|
|
[ '...', undefined ],
|
|
|
|
// removeParentheticals
|
|
[ 'Foo', 'Foo' ],
|
|
[ 'Foo (', 'Foo (' ],
|
|
[ 'Foo (Bar)', 'Foo' ],
|
|
[ 'Foo (Bar))', 'Foo (Bar))' ],
|
|
[ 'Foo )(Bar)', 'Foo )(Bar)' ],
|
|
[ '(Bar)', undefined ]
|
|
],
|
|
done = assert.async( cases.length );
|
|
|
|
$.each( cases, function ( _, testCase ) {
|
|
var fetchPreview = createGatewayWithPage( {
|
|
revisions: [ {
|
|
timestamp: '2016-11-11T18:28:43Z' // As data.revisions[0] is accessed.
|
|
} ],
|
|
extract: testCase[0]
|
|
} );
|
|
|
|
fetchPreview( 'Extract' ).done( function ( result ) {
|
|
assert.strictEqual( result.extract, testCase[1] );
|
|
|
|
done();
|
|
} );
|
|
} );
|
|
} );
|
|
|
|
QUnit.test( 'it handles new-ish pages', function ( assert ) {
|
|
var done = assert.async( 1 ),
|
|
fetchPreview;
|
|
|
|
fetchPreview = createGatewayWithPage( {
|
|
revisions: [ {
|
|
timestamp: new Date().toString()
|
|
} ]
|
|
} );
|
|
|
|
fetchPreview( 'Is Recent?' ).done( function ( result ) {
|
|
assert.strictEqual( result.isRecent, true );
|
|
|
|
done();
|
|
} );
|
|
} );
|
|
|
|
QUnit.test( 'it handles missing pages', function ( assert ) {
|
|
var done = assert.async( 1 ),
|
|
fetchPreview;
|
|
|
|
fetchPreview = createGatewayWithPage( {
|
|
canonicalurl: 'http://dev.wiki.local.wmftest.net:8080/wiki/Missing_page',
|
|
contentmodel: 'wikitext',
|
|
editurl: 'http://dev.wiki.local.wmftest.net:8080/w/index.php?title=Missing_page&action=edit',
|
|
fullurl: 'http://dev.wiki.local.wmftest.net:8080/wiki/Missing_page',
|
|
missing: true,
|
|
ns: 0,
|
|
pagelanguage: 'en',
|
|
pagelanguagedir: 'ltr',
|
|
pagelanguagehtmlcode: 'en',
|
|
title: 'Missing page'
|
|
} );
|
|
|
|
fetchPreview( 'Missing page' ).done( function ( result ) {
|
|
assert.deepEqual( result, {
|
|
title: 'Missing page',
|
|
languageCode: 'en',
|
|
languageDirection: 'ltr',
|
|
url: 'http://dev.wiki.local.wmftest.net:8080/wiki/Missing_page',
|
|
extract: undefined
|
|
} );
|
|
|
|
done();
|
|
} );
|
|
} );
|
|
|
|
}( mediaWiki, jQuery ) );
|