mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Popups
synced 2024-09-23 10:21:11 +00:00
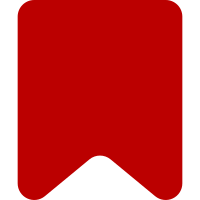
* Create new namespace mw.popups.gateway to contain them * Create folder resources/ext.popups/gateway/ * Split gateway{,.test}.js into gateway/{mediawiki,rest}{.test,}.js * Extract stateless functions out of factory scope now that there are no name collisions between the factories. Bug: T123445 Change-Id: Ib256871c3e4cfe3f13361cb66d4e9a67e9823c7b
68 lines
1.4 KiB
JavaScript
68 lines
1.4 KiB
JavaScript
( function ( mw ) {
|
|
|
|
var RESTBASE_ENDPOINT = '/api/rest_v1/page/summary/',
|
|
RESTBASE_PROFILE = 'https://www.mediawiki.org/wiki/Specs/Summary/1.0.0';
|
|
|
|
/**
|
|
* RESTBase gateway factory
|
|
*
|
|
* @param {Function} ajax function from jQuery for example
|
|
* @returns {ext.popups.Gateway}
|
|
*/
|
|
function createRESTBaseGateway( ajax ) {
|
|
|
|
/**
|
|
* Fetch page data from the API
|
|
*
|
|
* @param {String} title
|
|
* @return {jQuery.Promise}
|
|
*/
|
|
function fetch( title ) {
|
|
return ajax( {
|
|
url: RESTBASE_ENDPOINT + encodeURIComponent( title ),
|
|
headers: {
|
|
Accept: 'application/json; charset=utf-8' +
|
|
'profile="' + RESTBASE_PROFILE + '"'
|
|
}
|
|
} );
|
|
}
|
|
|
|
/**
|
|
* Get the page summary from the api and transform the data
|
|
*
|
|
* @param {String} title
|
|
* @returns {jQuery.Promise<ext.popups.PreviewModel>}
|
|
*/
|
|
function getPageSummary( title ) {
|
|
return fetch( title )
|
|
.then( convertPageToModel );
|
|
}
|
|
|
|
return {
|
|
fetch: fetch,
|
|
convertPageToModel: convertPageToModel,
|
|
getPageSummary: getPageSummary
|
|
};
|
|
}
|
|
|
|
/**
|
|
* Transform the rest API response to a preview model
|
|
*
|
|
* @param {Object} page
|
|
* @returns {ext.popups.PreviewModel}
|
|
*/
|
|
function convertPageToModel( page ) {
|
|
return mw.popups.preview.createModel(
|
|
page.title,
|
|
new mw.Title( page.title ).getUrl(),
|
|
page.lang,
|
|
page.dir,
|
|
page.extract,
|
|
page.thumbnail
|
|
);
|
|
}
|
|
|
|
mw.popups.gateway.createRESTBaseGateway = createRESTBaseGateway;
|
|
|
|
}( mediaWiki ) );
|