mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Popups
synced 2024-11-15 11:46:55 +00:00
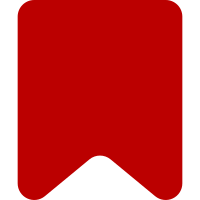
Mixing in the delay was introduced in If3f1a06f so that the total RTT for an API request could be calculated. Now that the FETCH_END action is dispatched when the gateway request ends and not when the preview model is resolved, this additional information (state) is redundant. Change-Id: I7e6ffe0945ffedd9425525fa7da855e729d50b77
140 lines
2.2 KiB
JavaScript
140 lines
2.2 KiB
JavaScript
var statsv = require( '../../../src/reducers/statsv' );
|
|
|
|
QUnit.module( 'ext.popups/reducers#eventLogging', {
|
|
setup: function () {
|
|
this.initialState = statsv( undefined, {
|
|
type: '@@INIT'
|
|
} );
|
|
}
|
|
} );
|
|
|
|
QUnit.test( '@@INIT', function ( assert ) {
|
|
assert.expect( 1 );
|
|
|
|
assert.deepEqual( this.initialState, {} );
|
|
} );
|
|
|
|
QUnit.test( 'FETCH_START', function ( assert ) {
|
|
var action, state;
|
|
|
|
assert.expect( 1 );
|
|
|
|
action = {
|
|
type: 'FETCH_START',
|
|
timestamp: 123
|
|
};
|
|
state = statsv( this.initialState, action );
|
|
|
|
assert.deepEqual(
|
|
state,
|
|
{
|
|
fetchStartedAt: 123
|
|
}
|
|
);
|
|
} );
|
|
|
|
QUnit.test( 'FETCH_END', function ( assert ) {
|
|
var startedAt, endedAt, delay, action, state;
|
|
|
|
assert.expect( 1 );
|
|
|
|
startedAt = 200;
|
|
endedAt = 500;
|
|
action = {
|
|
type: 'FETCH_END',
|
|
timestamp: endedAt,
|
|
};
|
|
state = statsv( { fetchStartedAt: startedAt }, action );
|
|
|
|
assert.deepEqual(
|
|
state,
|
|
{
|
|
fetchStartedAt: startedAt,
|
|
action: 'timing.PagePreviewsApiResponse',
|
|
data: endedAt - startedAt
|
|
}
|
|
);
|
|
} );
|
|
|
|
QUnit.test( 'FETCH_FAILED', function ( assert ) {
|
|
var action, state;
|
|
|
|
assert.expect( 1 );
|
|
|
|
action = {
|
|
type: 'FETCH_FAILED'
|
|
};
|
|
state = statsv( {}, action );
|
|
|
|
assert.deepEqual(
|
|
state,
|
|
{
|
|
action: 'counter.PagePreviewsApiFailure',
|
|
data: 1
|
|
}
|
|
);
|
|
} );
|
|
|
|
QUnit.test( 'LINK_DWELL', function ( assert ) {
|
|
var timestamp, action, state;
|
|
|
|
assert.expect( 1 );
|
|
|
|
timestamp = 100;
|
|
action = {
|
|
type: 'LINK_DWELL',
|
|
timestamp: timestamp
|
|
};
|
|
state = statsv( {}, action );
|
|
|
|
assert.deepEqual(
|
|
state,
|
|
{
|
|
linkDwellStartedAt: timestamp
|
|
}
|
|
);
|
|
} );
|
|
|
|
QUnit.test( 'PREVIEW_SHOW', function ( assert ) {
|
|
var startedAt, endedAt, action, state;
|
|
|
|
assert.expect( 1 );
|
|
|
|
startedAt = 100;
|
|
endedAt = 300;
|
|
action = {
|
|
type: 'PREVIEW_SHOW',
|
|
timestamp: endedAt
|
|
};
|
|
state = statsv( { linkDwellStartedAt: startedAt }, action );
|
|
|
|
assert.deepEqual(
|
|
state,
|
|
{
|
|
linkDwellStartedAt: startedAt,
|
|
action: 'timing.PagePreviewsPreviewShow',
|
|
data: endedAt - startedAt
|
|
}
|
|
);
|
|
} );
|
|
|
|
QUnit.test( 'STATSV_LOGGED', function ( assert ) {
|
|
var action, state;
|
|
|
|
assert.expect( 1 );
|
|
|
|
action = {
|
|
type: 'STATSV_LOGGED'
|
|
};
|
|
state = statsv( { data: 123 }, action );
|
|
|
|
assert.deepEqual(
|
|
state,
|
|
{
|
|
action: null,
|
|
data: null
|
|
}
|
|
);
|
|
} );
|
|
|