mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Popups
synced 2024-11-15 03:34:03 +00:00
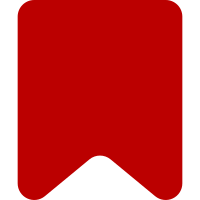
Excluding tests for the renderer which keeps failing. This will be readded in a later patch. Bug: T213415 Bug: T213908 Change-Id: If79fa3d0a7a20f121b1ceda6e0e33ad691b1ad30
77 lines
1.5 KiB
JavaScript
77 lines
1.5 KiB
JavaScript
/**
|
|
* Creates a **minimal** stub that can be used in place of an `mw.User`
|
|
* instance.
|
|
*
|
|
* @param {boolean} isAnon The return value of the `#isAnon`.
|
|
* @return {Object}
|
|
*/
|
|
export function createStubUser( isAnon ) {
|
|
return {
|
|
getPageviewToken() {
|
|
return '9876543210';
|
|
},
|
|
isAnon() {
|
|
return isAnon;
|
|
},
|
|
sessionId() {
|
|
return '0123456789';
|
|
}
|
|
};
|
|
}
|
|
|
|
/**
|
|
* Creates a **minimal** stub that can be used in place of an `mw.Map`
|
|
* instance.
|
|
*
|
|
* @return {mw.Map}
|
|
*/
|
|
export function createStubMap() {
|
|
const m = new Map(); /* global Map */
|
|
m.get = function ( key, fallback ) {
|
|
fallback = arguments.length > 1 ? fallback : null;
|
|
if ( typeof key === 'string' ) {
|
|
return m.has( key ) ? Map.prototype.get.call( m, key ) : fallback;
|
|
}
|
|
// Invalid selection key
|
|
return null;
|
|
};
|
|
return m;
|
|
}
|
|
|
|
/**
|
|
* Creates a stub that can be used as a replacement to mw.experiements
|
|
* @param {string} bucket getBucket will respond with this bucket.
|
|
* @return {Object}
|
|
*/
|
|
export function createStubExperiments( bucket ) {
|
|
return {
|
|
getBucket() {
|
|
return bucket;
|
|
}
|
|
};
|
|
}
|
|
|
|
/**
|
|
* Creates a **minimal** stub that can be used in place of an instance of
|
|
* `mw.Title`.
|
|
*
|
|
* @param {!number} namespace
|
|
* @param {!string} prefixedDb, e.g. Foo, or File:Bar.jpg
|
|
* @param {string|null} [fragment]
|
|
* @return {!Object}
|
|
*/
|
|
export function createStubTitle( namespace, prefixedDb, fragment = null ) {
|
|
return {
|
|
namespace,
|
|
getPrefixedDb() {
|
|
return prefixedDb;
|
|
},
|
|
getUrl() {
|
|
return `/wiki/${ prefixedDb }`;
|
|
},
|
|
getFragment() {
|
|
return fragment;
|
|
}
|
|
};
|
|
}
|