mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Popups
synced 2024-11-15 11:46:55 +00:00
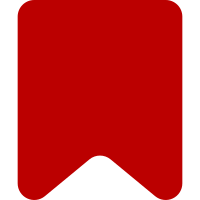
Use the standardised MediaWiki storage system for the simple use case of disabling/enabling Popups. The jStorage library is 12kb uncompressed and cannot be justified for this usage (and if it becomes the standardised library mw.storage will begin using it) This means that browsers with full localStorage or no localStorage support will not be able to disable Popups. Given the current ecosystem of the web - localStorage is widely supported - and the fact that grade A browsers enjoy localStorage support - this is not a problem. See https://github.com/wikimedia/mediawiki/blob/REL1_27/resources/src/startup.js#L59 Changes: * Stop using jStorage * Cleanup and migrate previous values in jStorage with plan for removing this in a month. Bug: T136241 Change-Id: I6dac2911e84d6cb20731be34add01576cf407c46
187 lines
4.6 KiB
JavaScript
187 lines
4.6 KiB
JavaScript
( function ( $, mw ) {
|
|
|
|
var currentLinkLogData,
|
|
/**
|
|
* @class mw.popups.settings
|
|
* @singleton
|
|
*/
|
|
settings = {};
|
|
|
|
/**
|
|
* The settings' dialog's section element.
|
|
* Defined in settings.open
|
|
* @property $element
|
|
*/
|
|
settings.$element = null;
|
|
|
|
/**
|
|
* Renders the relevant form and labels in the settings dialog
|
|
*
|
|
* @method render
|
|
*/
|
|
settings.render = function () {
|
|
var path = mw.config.get( 'wgExtensionAssetsPath' ) + '/Popups/resources/',
|
|
choices = [
|
|
{
|
|
id: 'simple',
|
|
name: mw.message( 'popups-settings-option-simple' ).text(),
|
|
description: mw.message( 'popups-settings-option-simple-description' ).text(),
|
|
image: path + 'images/hovercard.svg',
|
|
isChecked: true
|
|
},
|
|
{
|
|
id: 'advanced',
|
|
name: mw.message( 'popups-settings-option-advanced' ).text(),
|
|
description: mw.message( 'popups-settings-option-advanced-description' ).text(),
|
|
image: path + 'images/navpop.svg'
|
|
},
|
|
{
|
|
id: 'off',
|
|
name: mw.message( 'popups-settings-option-off' ).text(),
|
|
description: mw.message( 'popups-settings-option-off-description' ).text()
|
|
}
|
|
];
|
|
|
|
// Check if NavigationPopups is enabled
|
|
/*global pg: false*/
|
|
if ( typeof pg === 'undefined' || pg.fn.disablePopups === undefined ) {
|
|
// remove the advanced option
|
|
choices.splice( 1, 1 );
|
|
}
|
|
|
|
// render the template
|
|
settings.$element = mw.template.get( 'ext.popups.desktop', 'settings.mustache' ).render( {
|
|
heading: mw.message( 'popups-settings-title' ).text(),
|
|
closeLabel: mw.message( 'popups-settings-cancel' ).text(),
|
|
saveLabel: mw.message( 'popups-settings-save' ).text(),
|
|
helpText: mw.message( 'popups-settings-help' ).text(),
|
|
okLabel: mw.message( 'popups-settings-help-ok' ).text(),
|
|
descriptionText: mw.message( 'popups-settings-description' ).text(),
|
|
choices: choices
|
|
} );
|
|
|
|
// setup event bindings
|
|
settings.$element.find( '.save' ).click( settings.save );
|
|
settings.$element.find( '.close' ).click( settings.close );
|
|
settings.$element.find( '.okay' ).click( function () {
|
|
settings.close();
|
|
settings.reloadPage();
|
|
} );
|
|
|
|
$( 'body' ).append( settings.$element );
|
|
};
|
|
|
|
/**
|
|
* Save the setting to the device and close the dialog
|
|
*
|
|
* @method save
|
|
*/
|
|
settings.save = function () {
|
|
var v = $( 'input[name=mwe-popups-setting]:checked', '#mwe-popups-settings' ).val();
|
|
if ( v === 'simple' ) {
|
|
mw.popups.saveEnabledState( true );
|
|
settings.reloadPage();
|
|
settings.close();
|
|
} else {
|
|
mw.popups.saveEnabledState( false );
|
|
$( '#mwe-popups-settings-form' ).hide();
|
|
$( '#mwe-popups-settings-help' ).show();
|
|
mw.track( 'ext.popups.schemaPopups', $.extend( {}, currentLinkLogData, {
|
|
action: 'disabled'
|
|
} ) );
|
|
}
|
|
};
|
|
|
|
/**
|
|
* Show the settings element and position it correctly
|
|
*
|
|
* @method open
|
|
* @param {Object} logData data to log
|
|
*/
|
|
settings.open = function ( logData ) {
|
|
var
|
|
h = $( window ).height(),
|
|
w = $( window ).width();
|
|
|
|
currentLinkLogData = logData;
|
|
|
|
$( 'body' ).append( $( '<div>' ).addClass( 'mwe-popups-overlay' ) );
|
|
|
|
if ( !settings.$element ) {
|
|
settings.render();
|
|
}
|
|
|
|
settings.$element
|
|
.show()
|
|
.css( 'left', ( w - 600 ) / 2 )
|
|
.css( 'top', ( h - settings.$element.outerHeight( true ) ) / 2 );
|
|
|
|
return false;
|
|
};
|
|
|
|
/**
|
|
* Close the setting dialog and remove the overlay.
|
|
* If the close button is clicked on the help dialog
|
|
* save the setting and reload the page.
|
|
*
|
|
* @method close
|
|
*/
|
|
settings.close = function () {
|
|
if ( $( '#mwe-popups-settings-help' ).is( ':visible' ) ) {
|
|
settings.reloadPage();
|
|
} else {
|
|
$( '.mwe-popups-overlay' ).remove();
|
|
settings.$element.hide();
|
|
}
|
|
};
|
|
|
|
/**
|
|
* Adds a link to the footer to re-enable hovercards
|
|
*
|
|
* @method addFooterLink
|
|
*/
|
|
settings.addFooterLink = function () {
|
|
var $setting, $footer;
|
|
|
|
if ( mw.popups.enabled ) {
|
|
return false;
|
|
}
|
|
|
|
$setting = $( '<li>' ).append(
|
|
$( '<a>' )
|
|
.attr( 'href', '#' )
|
|
.text( mw.message( 'popups-settings-enable' ).text() )
|
|
.click( function ( e ) {
|
|
settings.open();
|
|
e.preventDefault();
|
|
} )
|
|
);
|
|
$footer = $( '#footer-places, #f-list' );
|
|
|
|
// From https://en.wikipedia.org/wiki/MediaWiki:Gadget-ReferenceTooltips.js
|
|
if ( $footer.length === 0 ) {
|
|
$footer = $( '#footer li' ).parent();
|
|
}
|
|
$footer.append( $setting );
|
|
};
|
|
|
|
/**
|
|
* Wrapper around window.location.reload. Exposed for testing purposes only.
|
|
*
|
|
* @private
|
|
* @ignore
|
|
*/
|
|
settings.reloadPage = function () {
|
|
location.reload();
|
|
};
|
|
|
|
$( function () {
|
|
if ( !mw.popups.enabled ) {
|
|
settings.addFooterLink();
|
|
}
|
|
} );
|
|
|
|
mw.popups.settings = settings;
|
|
|
|
} )( jQuery, mediaWiki );
|