mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Popups
synced 2024-12-02 19:26:24 +00:00
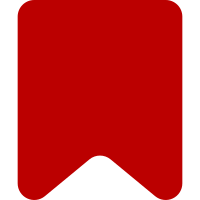
Use schema revision 16163887. Add the 'checkin' action, which is accompanied by the 'checkin' property. The action is logged at the following seconds (Fibonacci numbers) after the page loads: 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, 144, 233, 377, 610, 987, 1597, 2584, 4181, 6765. The `checkin` property contains the values listed above. Bug: T147314 Change-Id: Ib9ec7bd0e60aa34a04e32222b025347f6ee31794
112 lines
3.2 KiB
JavaScript
112 lines
3.2 KiB
JavaScript
( function ( mw ) {
|
|
var pageVisibility = {
|
|
/**
|
|
* Cached value of the browser specific name of the `document.hidden` property
|
|
*
|
|
* Exposed for testing only.
|
|
*
|
|
* @type {null|undefined|string}
|
|
* @private
|
|
*/
|
|
documentHiddenPropertyName: null,
|
|
/**
|
|
* Cached value of the browser specific name of the `document.visibilitychange` event
|
|
*
|
|
* Exposed for testing only.
|
|
*
|
|
* @type {null|undefined|string}
|
|
* @private
|
|
*/
|
|
documentVisibilityChangeEventName: null
|
|
};
|
|
|
|
/**
|
|
* Return the browser specific name of the `document.hidden` property
|
|
*
|
|
* Exposed for testing only.
|
|
*
|
|
* @see https://www.w3.org/TR/page-visibility/#dom-document-hidden
|
|
* @see https://developer.mozilla.org/en-US/docs/Web/API/Page_Visibility_API
|
|
* @private
|
|
* @param {Object} doc window.document object
|
|
* @return {string|undefined}
|
|
*/
|
|
pageVisibility.getDocumentHiddenPropertyName = function ( doc ) {
|
|
var property;
|
|
|
|
if ( pageVisibility.documentHiddenPropertyName === null ) {
|
|
if ( doc.hidden !== undefined ) {
|
|
property = 'hidden';
|
|
} else if ( doc.mozHidden !== undefined ) {
|
|
property = 'mozHidden';
|
|
} else if ( doc.msHidden !== undefined ) {
|
|
property = 'msHidden';
|
|
} else if ( doc.webkitHidden !== undefined ) {
|
|
property = 'webkitHidden';
|
|
} else {
|
|
// let's be explicit about returning `undefined`
|
|
property = undefined;
|
|
}
|
|
// cache
|
|
pageVisibility.documentHiddenPropertyName = property;
|
|
}
|
|
|
|
return pageVisibility.documentHiddenPropertyName;
|
|
};
|
|
|
|
/**
|
|
* Return the browser specific name of the `document.visibilitychange` event
|
|
*
|
|
* Exposed for testing only.
|
|
*
|
|
* @see https://www.w3.org/TR/page-visibility/#sec-visibilitychange-event
|
|
* @see https://developer.mozilla.org/en-US/docs/Web/API/Page_Visibility_API
|
|
* @private
|
|
* @param {Object} doc window.document object
|
|
* @return {string|undefined}
|
|
*/
|
|
pageVisibility.getDocumentVisibilitychangeEventName = function ( doc ) {
|
|
var eventName;
|
|
|
|
if ( pageVisibility.documentVisibilityChangeEventName === null ) {
|
|
if ( doc.hidden !== undefined ) {
|
|
eventName = 'visibilitychange';
|
|
} else if ( doc.mozHidden !== undefined ) {
|
|
eventName = 'mozvisibilitychange';
|
|
} else if ( doc.msHidden !== undefined ) {
|
|
eventName = 'msvisibilitychange';
|
|
} else if ( doc.webkitHidden !== undefined ) {
|
|
eventName = 'webkitvisibilitychange';
|
|
} else {
|
|
// let's be explicit about returning `undefined`
|
|
eventName = undefined;
|
|
}
|
|
// cache
|
|
pageVisibility.documentVisibilityChangeEventName = eventName;
|
|
}
|
|
|
|
return pageVisibility.documentVisibilityChangeEventName;
|
|
};
|
|
|
|
/**
|
|
* Whether `window.document` is visible
|
|
*
|
|
* `undefined` is returned if the browser does not support the Visibility API.
|
|
*
|
|
* Exposed for testing only.
|
|
*
|
|
* @see https://www.w3.org/TR/page-visibility/#dom-document-hidden
|
|
* @see https://developer.mozilla.org/en-US/docs/Web/API/Page_Visibility_API
|
|
* @private
|
|
* @param {Object} doc window.document object
|
|
* @returns {boolean|undefined}
|
|
*/
|
|
pageVisibility.isDocumentHidden = function ( doc ) {
|
|
var property = pageVisibility.getDocumentHiddenPropertyName( doc );
|
|
|
|
return property !== undefined ? doc[ property ] : undefined;
|
|
};
|
|
|
|
mw.popups.pageVisibility = pageVisibility;
|
|
}( mediaWiki ) );
|