mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Popups
synced 2024-11-15 11:46:55 +00:00
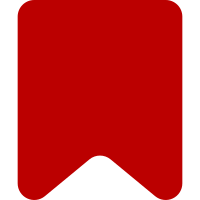
Functional changes - Show the default / error preview for all extract request failures except those due to network circumstances (such as CORS) or no connectivity (offline). Previously, the error preview was displayed only for missing pages. - FETCH_COMPLETE was previously only dispatched after FETCH_END. Now it's also dispatched after FETCH_FAILED. The additional "fetch complete" is not expected to impact logging. The states of success are: START, END, COMPLETE. The new failure states are consistent with success: START, FAILED, COMPLETE. Testing Errors may be stimulated in a number of ways including: - Timeout: add a timeout field to RESTBaseGateway / MediaWikiGateway.fetch(). http://api.jquery.com/jquery.ajax/ - Bad request: change MediaWikiGateway.fetch's action field to `Math.random() > 0.5 ? 'query' : 'fail'` and RESTBaseGateway.fetch's url field to `RESTBASE_ENDPOINT + ( Math.random() > 0.5 ? encodeURIComponent( title ) : '%%%' )`. - Desired Gateway can be configured in Gateway#createGateway(). - Note: T184534 describes a circumstance where cached previews may not appear when offline. Disable browser caching to avoid confusion. Bug: T183151 Bug: T184534 Change-Id: I7332284da0e0fb1ecd234a6f1e146ebd9ad8d81f
290 lines
5.3 KiB
JavaScript
290 lines
5.3 KiB
JavaScript
import preview from '../../../src/reducers/preview';
|
|
import actionTypes from '../../../src/actionTypes';
|
|
import { createNullModel } from '../../../src/preview/model';
|
|
|
|
QUnit.module( 'ext.popups/reducers#preview', {
|
|
beforeEach: function () {
|
|
this.el = 'active link';
|
|
}
|
|
} );
|
|
|
|
QUnit.test( '@@INIT', function ( assert ) {
|
|
var state = preview( undefined, { type: '@@INIT' } );
|
|
|
|
assert.expect( 1 );
|
|
|
|
assert.deepEqual(
|
|
state,
|
|
{
|
|
enabled: undefined,
|
|
activeLink: undefined,
|
|
activeEvent: undefined,
|
|
activeToken: '',
|
|
shouldShow: false,
|
|
isUserDwelling: false
|
|
}
|
|
);
|
|
} );
|
|
|
|
QUnit.test( 'BOOT', function ( assert ) {
|
|
var action = {
|
|
type: 'BOOT',
|
|
isEnabled: true
|
|
};
|
|
|
|
assert.expect( 1 );
|
|
|
|
assert.deepEqual(
|
|
preview( {}, action ),
|
|
{
|
|
enabled: true
|
|
},
|
|
'It should set whether or not previews are enabled.'
|
|
);
|
|
} );
|
|
|
|
QUnit.test( 'SETTINGS_CHANGE', function ( assert ) {
|
|
var action = {
|
|
type: 'SETTINGS_CHANGE',
|
|
enabled: true
|
|
};
|
|
|
|
assert.expect( 1 );
|
|
|
|
assert.deepEqual(
|
|
preview( {}, action ),
|
|
{
|
|
enabled: true
|
|
},
|
|
'It should set whether or not previews are enabled when settings change.'
|
|
);
|
|
} );
|
|
|
|
QUnit.test( 'LINK_DWELL initializes the state for a new link', function ( assert ) {
|
|
var action = {
|
|
type: 'LINK_DWELL',
|
|
el: this.el,
|
|
event: {},
|
|
token: '1234567890'
|
|
};
|
|
|
|
assert.deepEqual(
|
|
preview( {}, action ),
|
|
{
|
|
activeLink: action.el,
|
|
activeEvent: action.event,
|
|
activeToken: action.token,
|
|
shouldShow: false,
|
|
isUserDwelling: true
|
|
},
|
|
'It should set active link and event as well as interaction info and hide the preview.'
|
|
);
|
|
} );
|
|
|
|
QUnit.test( 'LINK_DWELL on an active link only updates dwell state', function ( assert ) {
|
|
var action = {
|
|
type: 'LINK_DWELL',
|
|
el: this.el,
|
|
event: {},
|
|
token: '1234567890'
|
|
},
|
|
state = {
|
|
activeLink: this.el,
|
|
isUserDwelling: false
|
|
};
|
|
|
|
assert.deepEqual(
|
|
preview( state, action ),
|
|
{
|
|
activeLink: this.el,
|
|
isUserDwelling: true
|
|
},
|
|
'It should only set isUserDwelling to true'
|
|
);
|
|
} );
|
|
|
|
QUnit.test( 'ABANDON_END', function ( assert ) {
|
|
var action = {
|
|
type: 'ABANDON_END',
|
|
token: 'bananas'
|
|
},
|
|
state = {
|
|
activeToken: 'bananas',
|
|
isUserDwelling: false
|
|
};
|
|
|
|
assert.deepEqual(
|
|
preview( state, action ),
|
|
{
|
|
activeLink: undefined,
|
|
activeToken: undefined,
|
|
activeEvent: undefined,
|
|
fetchResponse: undefined,
|
|
isUserDwelling: false,
|
|
shouldShow: false
|
|
},
|
|
'ABANDON_END should hide the preview and reset the interaction info.'
|
|
);
|
|
|
|
// ---
|
|
|
|
state = {
|
|
activeToken: 'apples',
|
|
isUserDwelling: true
|
|
};
|
|
|
|
assert.equal(
|
|
preview( state, action ),
|
|
state,
|
|
'ABANDON_END should NOOP if the current interaction has changed.'
|
|
);
|
|
|
|
// ---
|
|
|
|
state = {
|
|
activeToken: 'bananas',
|
|
isUserDwelling: true
|
|
};
|
|
|
|
assert.equal(
|
|
preview( state, action ),
|
|
state,
|
|
'ABANDON_END should NOOP if the user is dwelling on the preview.'
|
|
);
|
|
} );
|
|
|
|
QUnit.test( 'FETCH_COMPLETE', function ( assert ) {
|
|
var token = '1234567890',
|
|
state = {
|
|
activeToken: token,
|
|
isUserDwelling: true
|
|
},
|
|
action = {
|
|
type: 'FETCH_COMPLETE',
|
|
token: token,
|
|
result: {}
|
|
};
|
|
|
|
assert.expect( 3 );
|
|
|
|
assert.deepEqual(
|
|
preview( state, action ),
|
|
{
|
|
// Previous state.
|
|
activeToken: state.activeToken,
|
|
isUserDwelling: true,
|
|
|
|
fetchResponse: action.result,
|
|
shouldShow: true
|
|
},
|
|
'It should store the result and signal that a preview should be rendered.'
|
|
);
|
|
|
|
// ---
|
|
|
|
state = preview( state, {
|
|
type: 'ABANDON_START',
|
|
token: token
|
|
} );
|
|
|
|
assert.deepEqual(
|
|
preview( state, action ),
|
|
{
|
|
activeToken: token,
|
|
isUserDwelling: false, // Set when ABANDON_START is reduced.
|
|
|
|
fetchResponse: action.result,
|
|
shouldShow: false
|
|
},
|
|
'It shouldn\'t signal that a preview should be rendered if the user has abandoned the link since the gateway request was made.'
|
|
);
|
|
|
|
// ---
|
|
|
|
action = {
|
|
type: 'FETCH_COMPLETE',
|
|
token: 'banana',
|
|
result: {}
|
|
};
|
|
|
|
assert.deepEqual(
|
|
preview( state, action ),
|
|
state,
|
|
'It should NOOP if the user has interacted with another link since the gateway request was made.'
|
|
);
|
|
|
|
} );
|
|
|
|
QUnit.test( actionTypes.FETCH_FAILED, function ( assert ) {
|
|
var token = '1234567890',
|
|
state = {
|
|
activeToken: token,
|
|
isUserDwelling: true
|
|
},
|
|
action = {
|
|
type: actionTypes.FETCH_FAILED,
|
|
token: token
|
|
};
|
|
|
|
assert.expect( 2 );
|
|
|
|
assert.deepEqual(
|
|
preview( state, action ),
|
|
{
|
|
activeToken: state.activeToken,
|
|
isUserDwelling: true
|
|
},
|
|
'It should not transition states.'
|
|
);
|
|
|
|
// ---
|
|
|
|
action = {
|
|
type: actionTypes.FETCH_COMPLETE,
|
|
token: token,
|
|
result: { title: createNullModel( 'Title', '/wiki/Title' ) }
|
|
};
|
|
|
|
assert.deepEqual(
|
|
preview( state, action ),
|
|
{
|
|
activeToken: state.activeToken,
|
|
isUserDwelling: true,
|
|
|
|
fetchResponse: action.result,
|
|
shouldShow: true
|
|
},
|
|
'It should store the result and signal that an error preview should be rendered.'
|
|
);
|
|
} );
|
|
|
|
QUnit.test( 'PREVIEW_DWELL', function ( assert ) {
|
|
var action = {
|
|
type: 'PREVIEW_DWELL'
|
|
};
|
|
|
|
assert.expect( 1 );
|
|
|
|
assert.deepEqual(
|
|
preview( {}, action ),
|
|
{
|
|
isUserDwelling: true
|
|
},
|
|
'It should mark the preview as being dwelled on.'
|
|
);
|
|
} );
|
|
|
|
QUnit.test( 'ABANDON_START', function ( assert ) {
|
|
var action = {
|
|
type: 'ABANDON_START'
|
|
};
|
|
|
|
assert.deepEqual(
|
|
preview( {}, action ),
|
|
{
|
|
isUserDwelling: false
|
|
},
|
|
'ABANDON_START should mark the preview having been abandoned.'
|
|
);
|
|
} );
|