mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Popups
synced 2024-11-15 11:46:55 +00:00
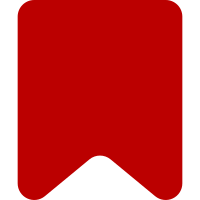
Creating a different page preview for disambiguation pages. This patch: - modifies the Preview model to accept a new 'type' property - modifies the Restbase Gateway to pass the 'type' prop to the Preview model - creates a new template to accept both generic/disambig previews - modifies the renderer to render the new template - generates icons for new template through resource loader - adds new i18n strings - modifies event-logging "preview seen" event to send new "disambiguation" previewType - updates event logging schema version - adds tests for Preview model and renderer for new preview type - does way too much? yes, yes it does. Bug: T168392 Change-Id: Idc936cc3eabbdd99a3d98f43c66b4cdbb7d24917
69 lines
1.9 KiB
JavaScript
69 lines
1.9 KiB
JavaScript
import { createModel, previewTypes }
|
|
from '../../../src/preview/model';
|
|
|
|
QUnit.module( 'ext.popups.preview#createModel' );
|
|
|
|
QUnit.test( 'it should copy the basic properties', function ( assert ) {
|
|
var thumbnail = {},
|
|
model = createModel(
|
|
'Foo',
|
|
'https://en.wikipedia.org/wiki/Foo',
|
|
'en',
|
|
'ltr',
|
|
'Foo bar baz.',
|
|
'standard',
|
|
thumbnail
|
|
);
|
|
|
|
assert.strictEqual( model.title, 'Foo' );
|
|
assert.strictEqual( model.url, 'https://en.wikipedia.org/wiki/Foo' );
|
|
assert.strictEqual( model.languageCode, 'en' );
|
|
assert.strictEqual( model.languageDirection, 'ltr' );
|
|
assert.strictEqual( model.type, previewTypes.TYPE_PAGE );
|
|
assert.strictEqual( model.thumbnail, thumbnail );
|
|
} );
|
|
|
|
QUnit.test( 'it computes the type property', function ( assert ) {
|
|
|
|
function createModelWith( { extract, type } ) {
|
|
return createModel(
|
|
'Foo',
|
|
'https://en.wikipedia.org/wiki/Foo',
|
|
'en',
|
|
'ltr',
|
|
extract,
|
|
type
|
|
);
|
|
}
|
|
|
|
assert.strictEqual(
|
|
createModelWith( { extract: 'Foo', type: 'standard' } ).type,
|
|
previewTypes.TYPE_PAGE,
|
|
'A non-generic ("page") preview has an extract and type "standard" property.'
|
|
);
|
|
|
|
assert.strictEqual(
|
|
createModelWith( { extract: 'Foo', type: undefined } ).type,
|
|
previewTypes.TYPE_PAGE,
|
|
'A non-generic ("page") preview has an extract with an undefined "type" property.'
|
|
);
|
|
|
|
assert.strictEqual(
|
|
createModelWith( { extract: undefined, type: undefined } ).type,
|
|
previewTypes.TYPE_GENERIC,
|
|
'A generic ("empty") preview has an undefined extract and an undefined "type" property.'
|
|
);
|
|
|
|
assert.strictEqual(
|
|
createModelWith( { extract: undefined, type: 'standard' } ).type,
|
|
previewTypes.TYPE_GENERIC,
|
|
'A generic ("empty") preview has an undefined extract regardless of "type".'
|
|
);
|
|
|
|
assert.strictEqual(
|
|
createModelWith( { extract: 'Foo', type: 'disambiguation' } ).type,
|
|
previewTypes.TYPE_DISAMBIGUATION,
|
|
'A disambiguation preview has an extract and type ("disambiguation") property.'
|
|
);
|
|
} );
|