mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Popups
synced 2024-11-13 17:56:55 +00:00
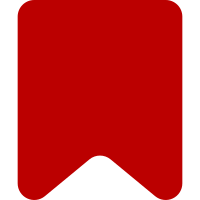
The invocation of `rm -rf resources/dist` in package.json (`check-built-assets`) is replaced with clean-webpack-plugin. The benefit of this change is that calling `npm run build` now works the same as the `check-built-assets` script. Bug: T193522 Change-Id: I64f048855ddceb7159279671b2174a7937e169ff
94 lines
2.5 KiB
JavaScript
94 lines
2.5 KiB
JavaScript
/* global __dirname, process */
|
|
const path = require( 'path' ),
|
|
webpack = require( 'webpack' ),
|
|
CleanPlugin = require( 'clean-webpack-plugin' ),
|
|
PUBLIC_PATH = '/w/extensions/Popups',
|
|
isProduction = process.env.NODE_ENV === 'production';
|
|
|
|
const reduxPath = isProduction ?
|
|
'node_modules/redux/dist/redux.min.js' :
|
|
'node_modules/redux/dist/redux.js';
|
|
|
|
const reduxThunkPath = isProduction ?
|
|
'node_modules/redux-thunk/dist/redux-thunk.min.js' :
|
|
'node_modules/redux-thunk/dist/redux-thunk.js';
|
|
|
|
const conf = {
|
|
output: {
|
|
// The absolute path to the output directory.
|
|
path: path.resolve( __dirname, 'resources/dist' ),
|
|
devtoolModuleFilenameTemplate: `${PUBLIC_PATH}/[resource-path]`,
|
|
|
|
// Write each chunk (entries, here) to a file named after the entry, e.g.
|
|
// the "index" entry gets written to index.js.
|
|
filename: '[name].js',
|
|
// as we cannot serve .map files from production servers store map files
|
|
// with .json extension
|
|
sourceMapFilename: '[file].json'
|
|
},
|
|
entry: { index: './src' },
|
|
performance: {
|
|
hints: isProduction ? 'error' : false,
|
|
maxAssetSize: 40 * 1024,
|
|
maxEntrypointSize: 40 * 1024,
|
|
assetFilter: function ( filename ) {
|
|
// The default filter excludes map files but we rename ours to .filename.
|
|
return filename.endsWith( '.js' );
|
|
}
|
|
},
|
|
devtool: 'source-map',
|
|
resolve: {
|
|
alias: {
|
|
redux: path.resolve( __dirname, reduxPath ),
|
|
'redux-thunk': path.resolve( __dirname, reduxThunkPath )
|
|
}
|
|
},
|
|
module: {
|
|
rules: [
|
|
{
|
|
test: /\.js$/,
|
|
exclude: /node_modules/,
|
|
use: {
|
|
loader: 'babel-loader',
|
|
options: { cacheDirectory: true }
|
|
}
|
|
},
|
|
{
|
|
test: /\.svg$/,
|
|
loader: 'svg-inline-loader',
|
|
options: {
|
|
removeSVGTagAttrs: false // Keep width and height attributes.
|
|
}
|
|
}
|
|
]
|
|
},
|
|
plugins: [
|
|
new CleanPlugin( 'resources/dist', { verbose: false } ),
|
|
|
|
// To generate identifiers that are preserved over builds, webpack supplies
|
|
// the NamedModulesPlugin (generates comments with file names on bundle)
|
|
// https://webpack.js.org/guides/caching/#deterministic-hashes
|
|
new webpack.NamedModulesPlugin()
|
|
]
|
|
};
|
|
|
|
// Production settings.
|
|
// Define the global process.env.NODE_ENV so that libraries like redux and
|
|
// redux-thunk get development code trimmed.
|
|
// Enable minimize flags for webpack loaders and disable debug.
|
|
if ( isProduction ) {
|
|
conf.plugins = conf.plugins.concat( [
|
|
new webpack.LoaderOptionsPlugin( {
|
|
minimize: true,
|
|
debug: false
|
|
} ),
|
|
new webpack.DefinePlugin( {
|
|
'process.env': {
|
|
NODE_ENV: JSON.stringify( 'production' )
|
|
}
|
|
} )
|
|
] );
|
|
}
|
|
|
|
module.exports = conf;
|