mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Popups
synced 2024-11-24 07:34:11 +00:00
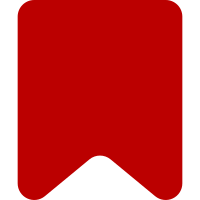
Note how getIsEnabled() is documented: "if the user hasn't previously enabled or disabled Page Previews […] then they are treated as if they have enabled them." In other words: The idea that the default should be true is encoded twice in this code. This is just not necessary. We can remove one without loosing anything. Motivtion: Simplifying the code and reducing the package size. Since the code fundamentally depends on this default value anyway, we can clear the users localStorage when they decide to go back to the default – instead of storing a "1" which does the same as the default. Change-Id: I2814a1e9269979918609162a508eeee6944d9e52
85 lines
1.7 KiB
JavaScript
85 lines
1.7 KiB
JavaScript
/**
|
|
* Creates a **minimal** stub that can be used in place of an `mw.User`
|
|
* instance.
|
|
*
|
|
* @param {boolean} isAnon The return value of the `#isAnon`.
|
|
* @return {Object}
|
|
*/
|
|
export function createStubUser( isAnon ) {
|
|
return {
|
|
getPageviewToken() {
|
|
return '9876543210';
|
|
},
|
|
isAnon() {
|
|
return isAnon;
|
|
},
|
|
sessionId() {
|
|
return '0123456789';
|
|
}
|
|
};
|
|
}
|
|
|
|
/**
|
|
* Creates a **minimal** stub that can be used in place of an `mw.Map`
|
|
* instance.
|
|
*
|
|
* @return {mw.Map}
|
|
*/
|
|
export function createStubMap() {
|
|
const m = new Map();
|
|
m.get = function ( key, fallback ) {
|
|
fallback = arguments.length > 1 ? fallback : null;
|
|
if ( typeof key === 'string' ) {
|
|
return m.has( key ) ? Map.prototype.get.call( m, key ) : fallback;
|
|
}
|
|
// Invalid selection key
|
|
return null;
|
|
};
|
|
m.remove = m.delete;
|
|
return m;
|
|
}
|
|
|
|
/**
|
|
* Creates a stub that can be used as a replacement to mw.experiements
|
|
*
|
|
* @param {string} bucket getBucket will respond with this bucket.
|
|
* @return {Object}
|
|
*/
|
|
export function createStubExperiments( bucket ) {
|
|
return {
|
|
getBucket() {
|
|
return bucket;
|
|
}
|
|
};
|
|
}
|
|
|
|
/**
|
|
* Creates a **minimal** stub that can be used in place of an instance of
|
|
* `mw.Title`.
|
|
*
|
|
* @param {number} namespace
|
|
* @param {string} name Page name without namespace prefix
|
|
* @param {string|null} [fragment]
|
|
* @return {Object}
|
|
*/
|
|
export function createStubTitle( namespace, name, fragment = null ) {
|
|
return {
|
|
namespace,
|
|
getPrefixedDb() {
|
|
return ( namespace ? `Namespace ${namespace}:` : '' ) + name;
|
|
},
|
|
getMainText() {
|
|
return name;
|
|
},
|
|
getNamespaceId() {
|
|
return namespace;
|
|
},
|
|
getUrl() {
|
|
return `/wiki/${this.getPrefixedDb()}`;
|
|
},
|
|
getFragment() {
|
|
return fragment;
|
|
}
|
|
};
|
|
}
|