mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Popups
synced 2024-11-15 11:46:55 +00:00
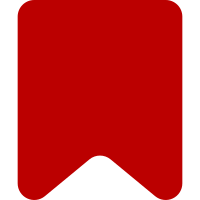
Whenever an HTTP request sequence is started, i.e. wait for the fetch
start time, issue a network request, and return the result, abort the
process if the results are known to no longer be needed. This occurs
when a user has dwelt upon one link and then abandoned it either during
the fetch start wait time or during the fetch network request itself.
This change is accomplished by preserving the pending promises in two
actions, LINK_DWELL and FETCH_START, and whenever the ABANDON_START
action is issued, it now aborts any previously pending XHR-like promise,
called a "AbortPromise" which is just a thenable with an abort() method.
There is a similar concept in Core:
ecc812f06e/resources/src/mediawiki.api/index.js
.
Aborting pending requests has big implications for client and server
logging as requests are quickly canceled, especially on slower
connections. These differences can be observed on the network tab of
DevTools and the log in Redux DevTools.
Consider, for instance, the scenario of dwelling upon and quickly
abandoning a single link prior to this patch:
BOOT EVENT_LOGGED LINK_DWELL FETCH_START ABANDON_START FETCH_END STATSV_LOGGED ABANDON_END EVENT_LOGGED FETCH_COMPLETE
And after this patch when the fetch timer is canceled (prior to an
actual network request):
BOOT EVENT_LOGGED LINK_DWELL ABANDON_START ABANDON_END EVENT_LOGGED
In the above sequence, FETCH_* and STATSV_LOGGED actions never occur.
And after this patch when the network request itself is canceled:
BOOT EVENT_LOGGED LINK_DWELL FETCH_START ABANDON_START FETCH_FAILED STATSV_LOGGED FETCH_COMPLETE ABANDON_END EVENT_LOGGED
FETCH_FAILED occurs intentionally, STATSV_LOGGED and FETCH_COMPLETE
still happen even though the fetch didn't complete successfully, and
FETCH_END doesn't.
Additionally, since less data is transmitted, it's possible that the
timing and success rate of logging will improve on low bandwidth
connections.
Also, this patch tries to revise the JSDocs where possible to support
type checking and fix a call to the missing assert.fail() function in
changeListener.test.js.
Bug: T197700
Change-Id: I9a73b3086fc8fb0edd897a347b5497d5362e20ef
64 lines
1.2 KiB
JavaScript
64 lines
1.2 KiB
JavaScript
import registerChangeListener from '../../src/changeListener';
|
|
|
|
const stubStore = ( () => {
|
|
let state;
|
|
|
|
return {
|
|
getState() {
|
|
return state;
|
|
},
|
|
setState( value ) {
|
|
state = value;
|
|
}
|
|
};
|
|
} )();
|
|
|
|
QUnit.module( 'ext.popups/changeListener' );
|
|
|
|
QUnit.test( 'it should only call the callback when the state has changed', function ( assert ) {
|
|
const spy = this.sandbox.spy();
|
|
let boundChangeListener;
|
|
|
|
stubStore.subscribe = ( changeListener ) => {
|
|
boundChangeListener = changeListener;
|
|
};
|
|
|
|
registerChangeListener( stubStore, spy );
|
|
|
|
assert.expect( 4, 'All assertions are executed.' );
|
|
|
|
stubStore.setState( {} );
|
|
|
|
if ( !boundChangeListener ) {
|
|
assert.ok( false, 'The change listener was not bound.' );
|
|
}
|
|
boundChangeListener();
|
|
boundChangeListener();
|
|
|
|
assert.strictEqual( spy.callCount, 1, 'The spy was called once.' );
|
|
assert.ok(
|
|
spy.calledWith(
|
|
undefined, // The initial internal state of the change listener.
|
|
{}
|
|
),
|
|
'The spy was called with the correct arguments.'
|
|
);
|
|
|
|
stubStore.setState( {
|
|
foo: 'bar'
|
|
} );
|
|
|
|
boundChangeListener();
|
|
|
|
assert.strictEqual( spy.callCount, 2, 'The spy was called twice.' );
|
|
assert.ok(
|
|
spy.calledWith(
|
|
{},
|
|
{
|
|
foo: 'bar'
|
|
}
|
|
),
|
|
'The spy was called with the correct arguments.'
|
|
);
|
|
} );
|