mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Popups
synced 2024-11-15 19:50:04 +00:00
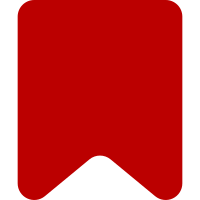
Supporting changes: * Remove the preview.previousActiveLink property from the state tree as it's unnecessary. Change-Id: I657decf9425a7a9e2b27a798ed60b162569661d8
157 lines
4.2 KiB
JavaScript
157 lines
4.2 KiB
JavaScript
( function ( mw, $ ) {
|
|
mw.popups.reducers = {};
|
|
|
|
/**
|
|
* Creates the next state tree from the current state tree and some updates.
|
|
*
|
|
* In [change listeners](/doc/change_listeners.md), for example, we talk about
|
|
* the previous state and the current state (the `prevState` and `state`
|
|
* parameters, respectively). Since
|
|
* [reducers](http://redux.js.org/docs/basics/Reducers.html) take the current
|
|
* state and an action and make updates, "next state" seems appropriate.
|
|
*
|
|
* @param {Object} state
|
|
* @param {Object} updates
|
|
* @return {Object}
|
|
*/
|
|
function nextState( state, updates ) {
|
|
var result = $.extend( {}, state ),
|
|
key;
|
|
|
|
for ( key in updates ) {
|
|
if ( updates.hasOwnProperty( key ) ) {
|
|
result[key] = updates[key];
|
|
}
|
|
}
|
|
|
|
return result;
|
|
}
|
|
|
|
/**
|
|
* Reducer for actions that modify the state of the preview model
|
|
*
|
|
* @param {Object} state before action
|
|
* @param {Object} action Redux action that modified state.
|
|
* Must have `type` property.
|
|
* @return {Object} state after action
|
|
*/
|
|
mw.popups.reducers.preview = function ( state, action ) {
|
|
if ( state === undefined ) {
|
|
state = {
|
|
enabled: undefined,
|
|
sessionToken: undefined,
|
|
pageToken: undefined,
|
|
linkInteractionToken: undefined,
|
|
activeLink: undefined,
|
|
interactionStarted: undefined,
|
|
isDelayingFetch: false,
|
|
isFetching: false
|
|
};
|
|
}
|
|
|
|
switch ( action.type ) {
|
|
case mw.popups.actionTypes.BOOT:
|
|
return nextState( state, {
|
|
enabled: action.isUserInCondition,
|
|
sessionToken: action.sessionToken,
|
|
pageToken: action.pageToken
|
|
} );
|
|
case mw.popups.actionTypes.LINK_DWELL:
|
|
return nextState( state, {
|
|
activeLink: action.el,
|
|
interactionStarted: action.interactionStarted,
|
|
isDelayingFetch: true,
|
|
linkInteractionToken: action.linkInteractionToken
|
|
} );
|
|
case mw.popups.actionTypes.LINK_ABANDON:
|
|
return nextState( state, {
|
|
activeLink: undefined,
|
|
interactionStarted: undefined,
|
|
isDelayingFetch: false,
|
|
linkInteractionToken: undefined
|
|
} );
|
|
case mw.popups.actionTypes.LINK_CLICK:
|
|
return nextState( state, {
|
|
isDelayingFetch: false
|
|
} );
|
|
case mw.popups.actionTypes.FETCH_START:
|
|
return nextState( state, {
|
|
isDelayingFetch: false,
|
|
isFetching: true,
|
|
fetchResponse: undefined
|
|
} );
|
|
case mw.popups.actionTypes.FETCH_END:
|
|
return nextState( state, {
|
|
isFetching: false,
|
|
fetchResponse: action.response
|
|
} );
|
|
case mw.popups.actionTypes.FETCH_FAILED:
|
|
return nextState( state, {
|
|
isFetching: false,
|
|
fetchResponse: action.response // To catch error data if it exists
|
|
} );
|
|
default:
|
|
return state;
|
|
}
|
|
};
|
|
|
|
/**
|
|
* Reducer for actions that modify the state of the view
|
|
*
|
|
* @param {Object} state before action
|
|
* @param {Object} action Redux action that modified state.
|
|
* Must have `type` property.
|
|
* @return {Object} state after action
|
|
*/
|
|
mw.popups.reducers.renderer = function ( state, action ) {
|
|
if ( state === undefined ) {
|
|
state = {
|
|
isAnimating: false,
|
|
isInteractive: false,
|
|
showSettings: false
|
|
};
|
|
}
|
|
|
|
switch ( action.type ) {
|
|
case mw.popups.actionTypes.PREVIEW_ANIMATING:
|
|
return $.extend( {}, state, {
|
|
isAnimating: true,
|
|
isInteractive: false,
|
|
showSettings: false
|
|
} );
|
|
case mw.popups.actionTypes.PREVIEW_INTERACTIVE:
|
|
return $.extend( OO.copy( state ), {
|
|
isAnimating: false,
|
|
isInteractive: true,
|
|
showSettings: false
|
|
} );
|
|
case mw.popups.actionTypes.PREVIEW_CLICK:
|
|
return $.extend( OO.copy( state ), {
|
|
isAnimating: false,
|
|
isInteractive: false,
|
|
showSettings: false
|
|
} );
|
|
case mw.popups.actionTypes.COG_CLICK:
|
|
return $.extend( OO.copy( state ), {
|
|
isAnimating: true,
|
|
isInteractive: false,
|
|
showSettings: true
|
|
} );
|
|
case mw.popups.actionTypes.SETTINGS_DIALOG_INTERACTIVE:
|
|
return $.extend( OO.copy( state ), {
|
|
isAnimating: false,
|
|
isInteractive: true,
|
|
showSettings: true
|
|
} );
|
|
case mw.popups.actionTypes.SETTINGS_DIALOG_CLOSED:
|
|
return $.extend( OO.copy( state ), {
|
|
isAnimating: false,
|
|
isInteractive: false,
|
|
showSettings: false
|
|
} );
|
|
default:
|
|
return state;
|
|
}
|
|
};
|
|
}( mediaWiki, jQuery ) );
|