mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Popups
synced 2024-11-15 11:46:55 +00:00
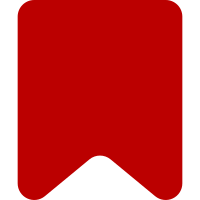
Why: Because they are the approved standard by TC39 and Ecma for JavaScript modules. Changes: * Wrap mw-node-qunit in run.js to register babel to transpile modules for node v6 * Change all sources in src/ to use ES modules * Change constants.js to be able to run without jQuery.bracketedDevicePixelRatio given ES modules are hoisted to the top by spec so we can't patch globals before importing it * Change all tests in tests/node-qunit/ to use ES modules * Drop usage of mock-require given ES modules are easy to stub with sinon Additional changes: * Rename tests/node-qunit/renderer.js to renderer.test.js to follow the convention of all the other files * Make npm run test:node run only .test.js test files so that it doesn't run the stubs.js or run.js file. Bug: T171951 Change-Id: I17a0b76041d5e2fd18e2d54950d9d7c0db99a941
95 lines
2.1 KiB
JavaScript
95 lines
2.1 KiB
JavaScript
import { createStubUser, createStubMap } from './stubs';
|
|
import createUserSettings from '../../src/userSettings';
|
|
|
|
QUnit.module( 'ext.popups/userSettings', {
|
|
beforeEach: function () {
|
|
var stubUser = createStubUser( /* isAnon = */ true );
|
|
|
|
this.storage = createStubMap();
|
|
this.userSettings = createUserSettings( this.storage, stubUser );
|
|
}
|
|
} );
|
|
|
|
QUnit.test( '#getIsEnabled should return false if Page Previews have been disabled', function ( assert ) {
|
|
assert.expect( 2 );
|
|
|
|
this.userSettings.setIsEnabled( false );
|
|
|
|
assert.notOk( this.userSettings.getIsEnabled() );
|
|
|
|
// ---
|
|
|
|
this.userSettings.setIsEnabled( true );
|
|
|
|
assert.ok(
|
|
this.userSettings.getIsEnabled(),
|
|
'#getIsEnabled should return true if Page Previews have been enabled'
|
|
);
|
|
} );
|
|
|
|
QUnit.test( '#hasIsEnabled', function ( assert ) {
|
|
var getStub;
|
|
|
|
assert.expect( 3 );
|
|
|
|
assert.notOk(
|
|
this.userSettings.hasIsEnabled(),
|
|
'#hasIsEnabled should return false if the storage is empty.'
|
|
);
|
|
|
|
// ---
|
|
|
|
this.userSettings.setIsEnabled( false );
|
|
|
|
assert.ok(
|
|
this.userSettings.hasIsEnabled(),
|
|
'#hasIsEnabled should return true even if "isEnabled" has been set to falsy.'
|
|
);
|
|
|
|
// ---
|
|
|
|
getStub = this.sandbox.stub( this.storage, 'get' ).returns( false );
|
|
|
|
assert.notOk(
|
|
this.userSettings.hasIsEnabled(),
|
|
'#hasIsEnabled should return false if the storage is disabled.'
|
|
);
|
|
|
|
getStub.restore();
|
|
} );
|
|
|
|
QUnit.test( '#getPreviewCount should return the count as a number', function ( assert ) {
|
|
assert.expect( 3 );
|
|
|
|
assert.strictEqual(
|
|
this.userSettings.getPreviewCount(),
|
|
0,
|
|
'#getPreviewCount returns 0 when the storage is empty.'
|
|
);
|
|
|
|
// ---
|
|
|
|
this.storage.set( 'ext.popups.core.previewCount', false );
|
|
|
|
assert.strictEqual(
|
|
this.userSettings.getPreviewCount(),
|
|
-1,
|
|
'#getPreviewCount returns -1 when the storage isn\'t available.'
|
|
);
|
|
|
|
// ---
|
|
|
|
this.storage.set( 'ext.popups.core.previewCount', '111' );
|
|
|
|
assert.strictEqual(
|
|
this.userSettings.getPreviewCount(),
|
|
111
|
|
);
|
|
} );
|
|
|
|
QUnit.test( '#setPreviewCount should store the count as a string', function ( assert ) {
|
|
this.userSettings.setPreviewCount( 222 );
|
|
|
|
assert.strictEqual( this.storage.get( 'ext.popups.core.previewCount' ), '222' );
|
|
} );
|