mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/PageImages
synced 2024-11-15 03:43:46 +00:00
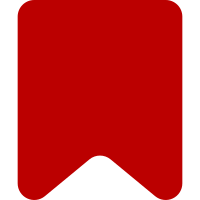
This tag is useless and does nothing without og:title and
og:description also being present, which is not the case
right now. A more complete patch should re-introduce all
three tags in one go.
It is also questionable if this tag belongs to this
extension, because it is explicitly said that the image is
an optional element of a Twitter card. og:title and
og:description are not optional. og:description would
probably be set by the TextExtract extension. This means
this Twitter card tag belongs more to TextExtracts and not
to PageImages.
This reverts commit 2e83a2c1dc
.
Bug: T157145
Change-Id: I17ffe8f83d91156a79facb4c35b4a15ecc49f108
218 lines
5.4 KiB
PHP
218 lines
5.4 KiB
PHP
<?php
|
|
|
|
/**
|
|
* @license WTFPL 2.0
|
|
* @author Max Semenik
|
|
* @author Brad Jorsch
|
|
* @author Thiemo Mättig
|
|
*/
|
|
class PageImages {
|
|
|
|
/**
|
|
* Page property used to store the best page image information.
|
|
* If the best image is the same as the best image with free license,
|
|
* then nothing is stored under this property.
|
|
* Note changing this value is not advised as it will invalidate all
|
|
* existing page property names on a production instance
|
|
* and cause them to be regenerated.
|
|
* @see PageImages::PROP_NAME_FREE
|
|
*/
|
|
const PROP_NAME = 'page_image';
|
|
|
|
/**
|
|
* Page property used to store the best free page image information
|
|
* Note changing this value is not advised as it will invalidate all
|
|
* existing page property names on a production instance
|
|
* and cause them to be regenerated.
|
|
*/
|
|
const PROP_NAME_FREE = 'page_image_free';
|
|
|
|
/**
|
|
* Get property name used in page_props table. When a page image
|
|
* is stored it will be stored under this property name on the corresponding
|
|
* article.
|
|
*
|
|
* @param bool $isFree Whether the image is a free-license image
|
|
* @return string
|
|
*/
|
|
public static function getPropName( $isFree ) {
|
|
return $isFree ? self::PROP_NAME_FREE : self::PROP_NAME;
|
|
}
|
|
|
|
/**
|
|
* Returns page image for a given title
|
|
*
|
|
* @param Title $title: Title to get page image for
|
|
*
|
|
* @return File|bool
|
|
*/
|
|
public static function getPageImage( Title $title ) {
|
|
$dbr = wfGetDB( DB_SLAVE );
|
|
$fileName = $dbr->selectField( 'page_props',
|
|
[ 'pp_value' ],
|
|
[ 'pp_page' => $title->getArticleID(), 'pp_propname' => [ self::PROP_NAME, self::PROP_NAME_FREE ] ],
|
|
__METHOD__,
|
|
[ 'ORDER BY' => 'pp_propname' ]
|
|
);
|
|
|
|
$file = false;
|
|
if ( $fileName ) {
|
|
$file = wfFindFile( $fileName );
|
|
}
|
|
|
|
return $file;
|
|
}
|
|
|
|
/**
|
|
* InfoAction hook handler, adds the page image to the info=action page
|
|
*
|
|
* @see https://www.mediawiki.org/wiki/Manual:Hooks/InfoAction
|
|
*
|
|
* @param IContextSource $context
|
|
* @param array[] &$pageInfo
|
|
*/
|
|
public static function onInfoAction( IContextSource $context, &$pageInfo ) {
|
|
global $wgThumbLimits;
|
|
|
|
$imageFile = self::getPageImage( $context->getTitle() );
|
|
if ( !$imageFile ) {
|
|
// The page has no image
|
|
return;
|
|
}
|
|
|
|
$thumbSetting = $context->getUser()->getOption( 'thumbsize' );
|
|
$thumbSize = $wgThumbLimits[$thumbSetting];
|
|
|
|
$thumb = $imageFile->transform( [ 'width' => $thumbSize ] );
|
|
if ( !$thumb ) {
|
|
return;
|
|
}
|
|
$imageHtml = $thumb->toHtml(
|
|
[
|
|
'alt' => $imageFile->getTitle()->getText(),
|
|
'desc-link' => true,
|
|
]
|
|
);
|
|
|
|
$pageInfo['header-basic'][] = [
|
|
$context->msg( 'pageimages-info-label' ),
|
|
$imageHtml
|
|
];
|
|
}
|
|
|
|
/**
|
|
* ApiOpenSearchSuggest hook handler, enhances ApiOpenSearch results with this extension's data
|
|
*
|
|
* @param array[] &$results
|
|
*/
|
|
public static function onApiOpenSearchSuggest( array &$results ) {
|
|
global $wgPageImagesExpandOpenSearchXml;
|
|
|
|
if ( !$wgPageImagesExpandOpenSearchXml || !count( $results ) ) {
|
|
return;
|
|
}
|
|
|
|
$pageIds = array_keys( $results );
|
|
$data = self::getImages( $pageIds, 50 );
|
|
foreach ( $pageIds as $id ) {
|
|
if ( isset( $data[$id]['thumbnail'] ) ) {
|
|
$results[$id]['image'] = $data[$id]['thumbnail'];
|
|
} else {
|
|
$results[$id]['image'] = null;
|
|
}
|
|
}
|
|
}
|
|
|
|
/**
|
|
* SpecialMobileEditWatchlist::images hook handler, adds images to mobile watchlist A-Z view
|
|
*
|
|
* @param IContextSource $context
|
|
* @param array[] $watchlist
|
|
* @param array[] &$images
|
|
*/
|
|
public static function onSpecialMobileEditWatchlist_images(
|
|
IContextSource $context, array $watchlist, array &$images
|
|
) {
|
|
$ids = [];
|
|
foreach ( $watchlist as $ns => $pages ) {
|
|
foreach ( array_keys( $pages ) as $dbKey ) {
|
|
$title = Title::makeTitle( $ns, $dbKey );
|
|
// Getting page ID here is safe because SpecialEditWatchlist::getWatchlistInfo()
|
|
// uses LinkBatch
|
|
$id = $title->getArticleID();
|
|
if ( $id ) {
|
|
$ids[$id] = $dbKey;
|
|
}
|
|
}
|
|
}
|
|
|
|
$data = self::getImages( array_keys( $ids ) );
|
|
foreach ( $data as $id => $page ) {
|
|
if ( isset( $page['pageimage'] ) ) {
|
|
$images[ $page['ns'] ][ $ids[$id] ] = $page['pageimage'];
|
|
}
|
|
}
|
|
}
|
|
|
|
/**
|
|
* Returns image information for pages with given ids
|
|
*
|
|
* @param int[] $pageIds
|
|
* @param int $size
|
|
*
|
|
* @return array[]
|
|
*/
|
|
private static function getImages( array $pageIds, $size = 0 ) {
|
|
$request = [
|
|
'action' => 'query',
|
|
'prop' => 'pageimages',
|
|
'piprop' => 'name',
|
|
'pageids' => implode( '|', $pageIds ),
|
|
'pilimit' => 'max',
|
|
];
|
|
|
|
if ( $size ) {
|
|
$request['piprop'] = 'thumbnail';
|
|
$request['pithumbsize'] = $size;
|
|
}
|
|
|
|
$api = new ApiMain( new FauxRequest( $request ) );
|
|
$api->execute();
|
|
|
|
if ( defined( 'ApiResult::META_CONTENT' ) ) {
|
|
return (array)$api->getResult()->getResultData( [ 'query', 'pages' ],
|
|
[ 'Strip' => 'base' ] );
|
|
} else {
|
|
$data = $api->getResultData();
|
|
if ( isset( $data['query']['pages'] ) ) {
|
|
return $data['query']['pages'];
|
|
}
|
|
return [];
|
|
}
|
|
}
|
|
|
|
public static function onRegistration() {
|
|
define( 'PAGE_IMAGES_INSTALLED', true );
|
|
}
|
|
|
|
/**
|
|
* @param OutputPage &$out
|
|
* @param Skin &$skin
|
|
*/
|
|
public static function onBeforePageDisplay( OutputPage &$out, Skin &$skin ) {
|
|
$imageFile = self::getPageImage( $out->getContext()->getTitle() );
|
|
if ( !$imageFile ) {
|
|
return;
|
|
}
|
|
|
|
// See https://developers.facebook.com/docs/sharing/best-practices?locale=en_US#tags
|
|
$thumb = $imageFile->transform( [ 'width' => 1200 ] );
|
|
if ( !$thumb ) {
|
|
return;
|
|
}
|
|
|
|
$out->addMeta( 'og:image', wfExpandUrl( $thumb->getUrl(), PROTO_CANONICAL ) );
|
|
}
|
|
|
|
}
|