mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/PageImages
synced 2024-11-15 12:00:40 +00:00
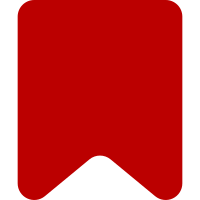
Titles that correspond to foreign files aren't included in the set of titles returned by ApiPageSet#getGoodTitles. However, since ApiQueryPageImages#execute finds files with wfFindFile, these "missing" titles can be included. Add the ApiQueryPageImages#getTitles helper function, which returns the set of "good" titles union the set of missing titles in the File namespace. Also, register the PHPUnit tests directory in the UnitTestsList hook handler. The original version of this patch was reverted due to a (stupid) bug wherein a fatal error was triggered because there was no check for there being no missing titles in the File namespace [0] and, consequently, an invalid operand was being passed to the array union operator. This bug is fixed as well as proven to work with a simple set of test cases. [0] https://git.wikimedia.org/blob/mediawiki%2Fextensions%2FPageImages.git/47137e7ee671c89667d620bc04ac7649b1d9af96/ApiQueryPageImages.php#L31 Bug: T98791 Bug: T114417 Change-Id: I923e88dde3a8ced4921b4192d90b4f3dc4b19e7b
84 lines
1.7 KiB
PHP
84 lines
1.7 KiB
PHP
<?php
|
|
|
|
namespace Tests;
|
|
|
|
use PHPUnit_Framework_TestCase;
|
|
use ApiPageSet;
|
|
use ApiQueryPageImages;
|
|
use Title;
|
|
|
|
class ApiPageSetStub extends ApiPageSet {
|
|
|
|
public function __construct( $goodTitles, $missingTitlesByNamespace ) {
|
|
$this->goodTitles = $goodTitles;
|
|
$this->missingTitlesByNamespace = $missingTitlesByNamespace;
|
|
}
|
|
|
|
public function getGoodTitles() {
|
|
return $this->goodTitles;
|
|
}
|
|
|
|
public function getMissingTitlesByNamespace() {
|
|
return $this->missingTitlesByNamespace;
|
|
}
|
|
}
|
|
|
|
class ApiQueryPageImagesProxy extends ApiQueryPageImages {
|
|
|
|
public function __construct( ApiPageSet $pageSet ) {
|
|
$this->pageSet = $pageSet;
|
|
}
|
|
|
|
public function getPageSet() {
|
|
return $this->pageSet;
|
|
}
|
|
|
|
public function getTitles() {
|
|
return parent::getTitles();
|
|
}
|
|
}
|
|
|
|
class ApiQueryPageImagesTest extends PHPUnit_Framework_TestCase {
|
|
|
|
/**
|
|
* @dataProvider provideGetTitles
|
|
*/
|
|
public function testGetTitles( $titles, $missingTitlesByNamespace, $expected ) {
|
|
$pageSet = new ApiPageSetStub( $titles, $missingTitlesByNamespace );
|
|
$queryPageImages = new ApiQueryPageImagesProxy( $pageSet );
|
|
|
|
$this->assertEquals( $expected, $queryPageImages->getTitles() );
|
|
}
|
|
|
|
public function provideGetTitles() {
|
|
return array(
|
|
array(
|
|
array( Title::newFromText( 'Foo' ) ),
|
|
array(),
|
|
array( Title::newFromText( 'Foo' ) ),
|
|
),
|
|
array(
|
|
array( Title::newFromText( 'Foo' ) ),
|
|
array(
|
|
NS_TALK => array(
|
|
'Bar' => -1,
|
|
),
|
|
),
|
|
array( Title::newFromText( 'Foo' ) ),
|
|
),
|
|
array(
|
|
array( Title::newFromText( 'Foo' ) ),
|
|
array(
|
|
NS_FILE => array(
|
|
'Bar' => -1,
|
|
),
|
|
),
|
|
array(
|
|
0 => Title::newFromText( 'Foo' ),
|
|
-1 => Title::newFromText( 'Bar', NS_FILE ),
|
|
),
|
|
),
|
|
);
|
|
}
|
|
}
|