mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/OATHAuth
synced 2024-11-13 18:16:56 +00:00
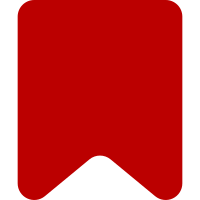
Add a new internal action=oathvalidate Action API module that can be used to validate an OATH token collected from a user. Using the module requires the 'oathauth-api-all' permission introduced in I4884f6e. Attempts to call the action for a given user are rate limited to only allow 10 failures per minute using the new 'badoath' key. The check is primarily useful as an internal network service in an environment where MediaWiki and other applications are sharing the same backing authentication store (e.g. LDAP) and the non-MediaWiki applications would like to respect the OATH protections enabled on the MediaWiki install. Complete usage in an LDAP shared auth environment would look something like: * Authenticate a user with the LDAP server via auth-bind * Call action=query&meta=oath as a privileged user to check for OATH protection. * If OATH is active for the account, prompt the user for their current OATH token. * Call action=oathvalidate as a privileged user to validate the token. * If validation succeeds, complete authentication. * If validation fails, do not authenticate the user. Bug: T144712 Change-Id: I1b18d9f3b99364fc47c760bdfc2047c1cbb5c04a
91 lines
2.5 KiB
PHP
91 lines
2.5 KiB
PHP
<?php
|
|
/**
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License along
|
|
* with this program; if not, write to the Free Software Foundation, Inc.,
|
|
* 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.
|
|
* http://www.gnu.org/copyleft/gpl.html
|
|
*/
|
|
|
|
/**
|
|
* Query module to check if a user has OATH authentication enabled.
|
|
*
|
|
* Usage requires the 'oathauth-api-all' grant which is not given to any group
|
|
* by default. Use of this API is security sensitive and should not be granted
|
|
* lightly. Configuring a special 'oathauth' user group is recommended.
|
|
*
|
|
* @ingroup API
|
|
* @ingroup Extensions
|
|
*/
|
|
class ApiQueryOATH extends ApiQueryBase {
|
|
public function __construct( $query, $moduleName ) {
|
|
parent::__construct( $query, $moduleName, 'oath' );
|
|
}
|
|
|
|
public function execute() {
|
|
$params = $this->extractRequestParams();
|
|
if ( $params['user'] === null ) {
|
|
$params['user'] = $this->getUser()->getName();
|
|
}
|
|
|
|
if ( !$this->getUser()->isAllowed( 'oathauth-api-all' ) ) {
|
|
$this->dieUsage(
|
|
'You do not have permission to check OATH status',
|
|
'permissiondenied'
|
|
);
|
|
}
|
|
|
|
$user = User::newFromName( $params['user'] );
|
|
if ( $user === false ) {
|
|
$this->dieUsageMsg( [ 'noname', $params['user'] ] );
|
|
}
|
|
|
|
$result = $this->getResult();
|
|
$data = [
|
|
ApiResult::META_BC_BOOLS => [ 'enabled' ],
|
|
'enabled' => false,
|
|
];
|
|
|
|
if ( !$user->isAnon() ) {
|
|
$oathUser = OATHAuthHooks::getOATHUserRepository()
|
|
->findByUser( $user );
|
|
$data['enabled'] = $oathUser && $oathUser->getKey() !== null;
|
|
}
|
|
$result->addValue( 'query', $this->getModuleName(), $data );
|
|
}
|
|
|
|
public function getCacheMode( $params ) {
|
|
return 'private';
|
|
}
|
|
|
|
public function isInternal() {
|
|
return true;
|
|
}
|
|
|
|
public function getAllowedParams() {
|
|
return [
|
|
'user' => [
|
|
ApiBase::PARAM_TYPE => 'user',
|
|
],
|
|
];
|
|
}
|
|
|
|
protected function getExamplesMessages() {
|
|
return [
|
|
'action=query&meta=oath'
|
|
=> 'apihelp-query+oath-example-1',
|
|
'action=query&meta=oath&oathuser=Example'
|
|
=> 'apihelp-query+oath-example-2',
|
|
];
|
|
}
|
|
}
|