mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/OATHAuth
synced 2024-11-12 09:37:23 +00:00
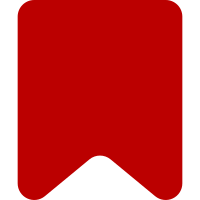
We need to pass the db name to getConnection, in addition to wfGetLB. Also, use core's CentralIdLookup for mapping local user to CentralId when using a central DB for OATH secret storage. Change-Id: I12a457633956a9a34dc5302ddcff468e31dd9cef
48 lines
1.2 KiB
PHP
48 lines
1.2 KiB
PHP
<?php
|
|
|
|
class OATHUserRepository {
|
|
private $dbr;
|
|
|
|
private $dbw;
|
|
|
|
public function __construct( LoadBalancer $lb ) {
|
|
global $wgOATHAuthDatabase;
|
|
$this->dbr = $lb->getConnection( DB_SLAVE, array(), $wgOATHAuthDatabase );
|
|
$this->dbw = $lb->getConnection( DB_MASTER, array(), $wgOATHAuthDatabase );
|
|
}
|
|
|
|
public function findByUser( User $user ) {
|
|
$oathUser = new OATHUser( $user, null );
|
|
|
|
$uid = CentralIdLookup::factory()->centralIdFromLocalUser( $user );
|
|
$res = $this->dbr->selectRow( 'oathauth_users', '*', array( 'id' => $uid ), __METHOD__ );
|
|
if ($res) {
|
|
$key = new OATHAuthKey( $res->secret, explode( ',', $res->scratch_tokens ) );
|
|
$oathUser->setKey( $key );
|
|
}
|
|
|
|
return $oathUser;
|
|
}
|
|
|
|
public function persist( OATHUser $user ) {
|
|
$this->dbw->replace(
|
|
'oathauth_users',
|
|
array( 'id' ),
|
|
array(
|
|
'id' => CentralIdLookup::factory()->centralIdFromLocalUser( $user->getUser() ),
|
|
'secret' => $user->getKey()->getSecret(),
|
|
'scratch_tokens' => implode( ',', $user->getKey()->getScratchTokens() ),
|
|
),
|
|
__METHOD__
|
|
);
|
|
}
|
|
|
|
public function remove( OATHUser $user ) {
|
|
$this->dbw->delete(
|
|
'oathauth_users',
|
|
array( 'id' => CentralIdLookup::factory()->centralIdFromLocalUser( $user->getUser() ) ),
|
|
__METHOD__
|
|
);
|
|
}
|
|
}
|