mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/OATHAuth
synced 2024-09-23 18:30:39 +00:00
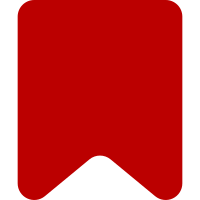
* array() -> [] * spacing fixes * dirname( __FILE__ ) -> __DIR__ * Add phpcs style checks using latest mediawiki-codesniffer to keep things clean. Co-Authored-By: Bryan Davis <bd808@wikimedia.org> Change-Id: I95735f928d3e5d6ac9d2a10d92b40ed01cf2737c
58 lines
897 B
PHP
58 lines
897 B
PHP
<?php
|
|
|
|
/**
|
|
* Class representing a user from OATH's perspective
|
|
*
|
|
* @ingroup Extensions
|
|
*/
|
|
class OATHUser {
|
|
/** @var User */
|
|
private $user;
|
|
|
|
/** @var OATHAuthKey|null */
|
|
private $key;
|
|
|
|
/**
|
|
* @param User $user
|
|
* @param OATHAuthKey $key
|
|
*/
|
|
public function __construct( User $user, OATHAuthKey $key = null ) {
|
|
$this->user = $user;
|
|
$this->key = $key;
|
|
}
|
|
|
|
/**
|
|
* @return User
|
|
*/
|
|
public function getUser() {
|
|
return $this->user;
|
|
}
|
|
|
|
/**
|
|
* @return String
|
|
*/
|
|
public function getAccount() {
|
|
global $wgSitename;
|
|
|
|
return "$wgSitename:{$this->user->getName()}";
|
|
}
|
|
|
|
/**
|
|
* Get the key associated with this user.
|
|
*
|
|
* @return null|OATHAuthKey
|
|
*/
|
|
public function getKey() {
|
|
return $this->key;
|
|
}
|
|
|
|
/**
|
|
* Set the key associated with this user.
|
|
*
|
|
* @param OATHAuthKey|null $key
|
|
*/
|
|
public function setKey( OATHAuthKey $key = null ) {
|
|
$this->key = $key;
|
|
}
|
|
}
|