mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/OATHAuth
synced 2024-09-24 10:49:57 +00:00
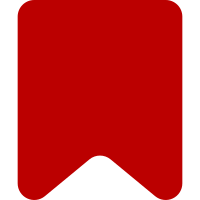
The issuer name is an optional but important feature that allows the user to differentiate between different accounts used in the same authenticator app. While we currently use a prefix in the user account name, declaring an issuer makes it easier for the user to differentiate. Bug: T150596 Change-Id: I741dd671e79e0326dfe97bdaaf63b3997960d115
67 lines
1.1 KiB
PHP
67 lines
1.1 KiB
PHP
<?php
|
|
|
|
/**
|
|
* Class representing a user from OATH's perspective
|
|
*
|
|
* @ingroup Extensions
|
|
*/
|
|
class OATHUser {
|
|
/** @var User */
|
|
private $user;
|
|
|
|
/** @var OATHAuthKey|null */
|
|
private $key;
|
|
|
|
/**
|
|
* @param User $user
|
|
* @param OATHAuthKey $key
|
|
*/
|
|
public function __construct( User $user, OATHAuthKey $key = null ) {
|
|
$this->user = $user;
|
|
$this->key = $key;
|
|
}
|
|
|
|
/**
|
|
* @return User
|
|
*/
|
|
public function getUser() {
|
|
return $this->user;
|
|
}
|
|
|
|
/**
|
|
* @return String
|
|
*/
|
|
public function getIssuer() {
|
|
global $wgSitename, $wgOATHAuthAccountPrefix;
|
|
if ( $wgOATHAuthAccountPrefix !== false ) {
|
|
return $wgOATHAuthAccountPrefix;
|
|
}
|
|
return $wgSitename;
|
|
}
|
|
|
|
/**
|
|
* @return String
|
|
*/
|
|
public function getAccount() {
|
|
return $this->user->getName();
|
|
}
|
|
|
|
/**
|
|
* Get the key associated with this user.
|
|
*
|
|
* @return null|OATHAuthKey
|
|
*/
|
|
public function getKey() {
|
|
return $this->key;
|
|
}
|
|
|
|
/**
|
|
* Set the key associated with this user.
|
|
*
|
|
* @param OATHAuthKey|null $key
|
|
*/
|
|
public function setKey( OATHAuthKey $key = null ) {
|
|
$this->key = $key;
|
|
}
|
|
}
|