mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Nuke
synced 2024-11-15 03:35:39 +00:00
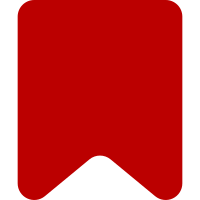
For example: * Remove redundant PHPDoc blocks that just duplicate existing information and aren't needed any more with our current PHPCS rule set. * Add some very obvious type declarations. * Use ??= where it makes sense. Change-Id: I5c182cc961c6eeccead79ff49b0376eee2418acf
47 lines
909 B
PHP
47 lines
909 B
PHP
<?php
|
|
|
|
namespace MediaWiki\Extension\Nuke\Hooks;
|
|
|
|
use MediaWiki\HookContainer\HookContainer;
|
|
use MediaWiki\Title\Title;
|
|
|
|
/**
|
|
* Handle running Nuke's hooks
|
|
* @author DannyS712
|
|
*/
|
|
class NukeHookRunner implements NukeDeletePageHook, NukeGetNewPagesHook {
|
|
|
|
private HookContainer $hookContainer;
|
|
|
|
public function __construct( HookContainer $hookContainer ) {
|
|
$this->hookContainer = $hookContainer;
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
*/
|
|
public function onNukeDeletePage( Title $title, string $reason, bool &$deletionResult ) {
|
|
return $this->hookContainer->run(
|
|
'NukeDeletePage',
|
|
[ $title, $reason, &$deletionResult ]
|
|
);
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
*/
|
|
public function onNukeGetNewPages(
|
|
string $username,
|
|
?string $pattern,
|
|
?int $namespace,
|
|
int $limit,
|
|
array &$pages
|
|
) {
|
|
return $this->hookContainer->run(
|
|
'NukeGetNewPages',
|
|
[ $username, $pattern, $namespace, $limit, &$pages ]
|
|
);
|
|
}
|
|
|
|
}
|