mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/MultimediaViewer
synced 2024-09-25 11:17:54 +00:00
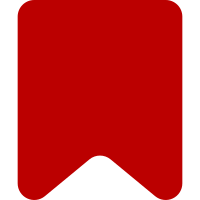
* moves generic logic into ThumbnailSizeCalculator class * moves UI-specific logic into interface class * fixes bug where non-bucketed sizes were served on devices with non-standard pixel density * fixes bug where bucketed size was compared to css size instead of screen size for resizing Change-Id: I8ba3380b74fcc8fb0a6ecc3f3140627411851ad0 Mingle: https://wikimedia.mingle.thoughtworks.com/projects/multimedia/cards/196
156 lines
5 KiB
PHP
156 lines
5 KiB
PHP
<?php
|
|
/*
|
|
* This file is part of the MediaWiki extension MultimediaViewer.
|
|
*
|
|
* MultimediaViewer is free software: you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation, either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* MultimediaViewer is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with MultimediaViewer. If not, see <http://www.gnu.org/licenses/>.
|
|
*
|
|
* @file
|
|
* @ingroup extensions
|
|
* @author Mark Holmquist <mtraceur@member.fsf.org>
|
|
* @copyright Copyright © 2013, Mark Holmquist
|
|
*/
|
|
|
|
class MultimediaViewerHooks {
|
|
/** Link to more information about this module */
|
|
protected static $infoLink = '//mediawiki.org/wiki/Special:MyLanguage/Multimedia/About_Media_Viewer';
|
|
|
|
/** Link to a page where this module can be discussed */
|
|
protected static $discussionLink = '//mediawiki.org/wiki/Special:MyLanguage/Talk:Multimedia/About_Media_Viewer';
|
|
|
|
/**
|
|
* Handler for all places where we add the modules
|
|
* Could be on article pages or on Category pages
|
|
* @param OutputPage $out
|
|
* @return bool
|
|
*/
|
|
protected static function getModules( &$out ) {
|
|
if ( class_exists( 'BetaFeatures')
|
|
&& !BetaFeatures::isFeatureEnabled( $out->getUser(), 'multimedia-viewer' ) ) {
|
|
return true;
|
|
}
|
|
|
|
$out->addModules( array( 'mmv' ) );
|
|
|
|
return true;
|
|
}
|
|
|
|
/**
|
|
* Handler for BeforePageDisplay hook
|
|
* Add JavaScript to the page when an image is on it
|
|
* and the user has enabled the feature if BetaFeatures is installed
|
|
* @param OutputPage $out
|
|
* @param Skin $skin
|
|
* @return bool
|
|
*/
|
|
public static function getModulesForArticle( &$out, &$skin ) {
|
|
if ( count( $out->getFileSearchOptions() ) > 0 ) {
|
|
return self::getModules( $out );
|
|
}
|
|
|
|
return true;
|
|
}
|
|
|
|
/**
|
|
* Handler for CategoryPageView hook
|
|
* Add JavaScript to the page if there are images in the category
|
|
* @param CategoryPage $catPage
|
|
* @return bool
|
|
*/
|
|
public static function getModulesForCategory( &$catPage ) {
|
|
$title = $catPage->getTitle();
|
|
$cat = Category::newFromTitle( $title );
|
|
if ( $cat->getFileCount() > 0 ) {
|
|
$out = $catPage->getContext()->getOutput();
|
|
return self::getModules( $out );
|
|
}
|
|
|
|
return true;
|
|
}
|
|
|
|
// Add a beta preference to gate the feature
|
|
public static function getBetaPreferences( $user, &$prefs ) {
|
|
global $wgExtensionAssetsPath;
|
|
|
|
$dir = RequestContext::getMain()->getLanguage()->getDir();
|
|
|
|
$prefs['multimedia-viewer'] = array(
|
|
'label-message' => 'multimediaviewer-pref',
|
|
'desc-message' => 'multimediaviewer-pref-desc',
|
|
'info-link' => self::$infoLink,
|
|
'discussion-link' => self::$discussionLink,
|
|
'screenshot' => "$wgExtensionAssetsPath/MultimediaViewer/viewer-$dir.svg",
|
|
);
|
|
|
|
return true;
|
|
}
|
|
|
|
/**
|
|
* Export variables used in both PHP and JS to keep DRY
|
|
* @param array $vars
|
|
* @return bool
|
|
*/
|
|
public static function resourceLoaderGetConfigVars( &$vars ) {
|
|
global $wgAPIPropModules, $wgNetworkPerformanceSamplingFactor;
|
|
$vars['wgMultimediaViewer'] = array(
|
|
'infoLink' => self::$infoLink,
|
|
'discussionLink' => self::$discussionLink,
|
|
'globalUsageAvailable' => isset( $wgAPIPropModules['globalusage'] ),
|
|
);
|
|
$vars['wgNetworkPerformanceSamplingFactor'] = $wgNetworkPerformanceSamplingFactor;
|
|
return true;
|
|
}
|
|
|
|
/**
|
|
* Get modules for testing our JavaScript
|
|
* @param array $testModules
|
|
* @param ResourceLoader resourceLoader
|
|
* @return bool
|
|
*/
|
|
public static function getTestModules( array &$testModules, ResourceLoader &$resourceLoader ) {
|
|
$testModules['qunit']['mmv.tests'] = array(
|
|
'scripts' => array(
|
|
'tests/qunit/mmv.testhelpers.js',
|
|
'tests/qunit/mmv.test.js',
|
|
'tests/qunit/mmv.model.test.js',
|
|
'tests/qunit/mmv.ThumbnailWidthCalculator.test.js',
|
|
'tests/qunit/provider/mmv.provider.Api.test.js',
|
|
'tests/qunit/mmv.performance.test.js',
|
|
'tests/qunit/provider/mmv.provider.ImageUsage.test.js',
|
|
'tests/qunit/provider/mmv.provider.GlobalUsage.test.js',
|
|
'tests/qunit/provider/mmv.provider.ImageInfo.test.js',
|
|
'tests/qunit/provider/mmv.provider.FileRepoInfo.test.js',
|
|
'tests/qunit/provider/mmv.provider.ThumbnailInfo.test.js',
|
|
'tests/qunit/provider/mmv.provider.UserInfo.test.js',
|
|
'tests/qunit/provider/mmv.provider.Image.test.js',
|
|
'tests/qunit/mmv.lightboxinterface.test.js',
|
|
'tests/qunit/mmv.ui.categories.test.js',
|
|
'tests/qunit/mmv.ui.description.test.js',
|
|
'tests/qunit/mmv.ui.fileUsage.test.js',
|
|
'tests/qunit/mmv.ui.metadataPanel.test.js',
|
|
'tests/qunit/lightboximage.test.js',
|
|
'tests/qunit/lightboxinterface.test.js',
|
|
'tests/qunit/multilightbox.test.js',
|
|
'tests/qunit/mmv.logger.test.js',
|
|
),
|
|
'dependencies' => array(
|
|
'mmv',
|
|
),
|
|
'localBasePath' => __DIR__,
|
|
'remoteExtPath' => 'MultimediaViewer',
|
|
);
|
|
|
|
return true;
|
|
}
|
|
}
|