mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/MultimediaViewer
synced 2024-09-25 03:09:40 +00:00
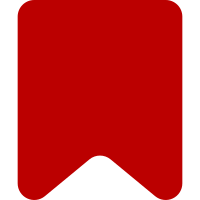
Fetch more license information from the imageinfo API (as needed by the reuse panel), store it in a new model Change-Id: I331598b55b86d13e23a8bcd58c3ad8fcab749e6f Mingle: https://wikimedia.mingle.thoughtworks.com/projects/multimedia/cards/369
276 lines
10 KiB
JavaScript
276 lines
10 KiB
JavaScript
( function ( mw, $ ) {
|
||
var thingsShouldBeEmptied = [
|
||
'$license',
|
||
'$title',
|
||
'$credit',
|
||
'$username',
|
||
'$location',
|
||
'$repo',
|
||
'$datetime'
|
||
],
|
||
|
||
thingsShouldHaveEmptyClass = [
|
||
'$license',
|
||
'$credit',
|
||
'$usernameLi',
|
||
'$locationLi',
|
||
'$repoLi',
|
||
'$datetimeLi',
|
||
'$progress'
|
||
];
|
||
|
||
QUnit.module( 'mmv.ui.metadataPanel', QUnit.newMwEnvironment() );
|
||
|
||
QUnit.test( 'The panel is emptied properly when necessary', thingsShouldBeEmptied.length + thingsShouldHaveEmptyClass.length + 2, function ( assert ) {
|
||
var i,
|
||
$qf = $( '#qunit-fixture' ),
|
||
panel = new mw.mmv.ui.MetadataPanel( $qf, $( '<div>' ).appendTo( $qf ) );
|
||
|
||
panel.empty();
|
||
|
||
for ( i = 0; i < thingsShouldBeEmptied.length; i++ ) {
|
||
assert.strictEqual( panel[thingsShouldBeEmptied[i]].text(), '', 'We successfully emptied the ' + thingsShouldBeEmptied[i] + ' element' );
|
||
}
|
||
|
||
for ( i = 0; i < thingsShouldHaveEmptyClass.length; i++ ) {
|
||
assert.strictEqual( panel[thingsShouldHaveEmptyClass[i]].hasClass( 'empty' ), true, 'We successfully applied the empty class to the ' + thingsShouldHaveEmptyClass[i] + ' element' );
|
||
}
|
||
|
||
assert.ok( !panel.$dragIcon.hasClass( 'pointing-down' ), 'We successfully reset the chevron' );
|
||
assert.ok( !panel.$container.hasClass( 'invite' ), 'We successfully reset the invite' );
|
||
} );
|
||
|
||
QUnit.test( 'Setting repository information in the UI works as expected', 3, function ( assert ) {
|
||
var $qf = $( '#qunit-fixture' ),
|
||
panel = new mw.mmv.ui.MetadataPanel( $qf, $( '<div>' ).appendTo( $qf ) );
|
||
|
||
panel.setRepoDisplay( 'Example Wiki' );
|
||
assert.strictEqual( panel.$repo.text(), 'Learn more on Example Wiki', 'Text set to something useful for remote wiki - if this fails it might be because of localisation' );
|
||
|
||
panel.setRepoDisplay();
|
||
assert.strictEqual( panel.$repo.text(), 'Learn more on ' + mw.config.get( 'wgSiteName' ), 'Text set to something useful for local wiki - if this fails it might be because of localisation' );
|
||
|
||
panel.setFilePageLink( 'https://commons.wikimedia.org/wiki/File:Foobar.jpg' );
|
||
assert.strictEqual( panel.$repo.prop( 'href' ), 'https://commons.wikimedia.org/wiki/File:Foobar.jpg', 'The file link was set successfully.' );
|
||
} );
|
||
|
||
QUnit.test( 'Setting location information works as expected', 6, function ( assert ) {
|
||
var $qf = $( '#qunit-fixture' ),
|
||
panel = new mw.mmv.ui.MetadataPanel( $qf, $( '<div>' ).appendTo( $qf ) ),
|
||
fileName = 'Foobar.jpg',
|
||
latitude = 12.3456789,
|
||
longitude = 98.7654321,
|
||
imageData = {
|
||
latitude: latitude,
|
||
longitude: longitude,
|
||
hasCoords: function() { return true; },
|
||
title: mw.Title.newFromText( 'File:Foobar.jpg' )
|
||
};
|
||
|
||
panel.setLocationData( imageData );
|
||
|
||
assert.strictEqual(
|
||
panel.$location.text(),
|
||
'Location: 12° 20′ 44.44″ N, 98° 45′ 55.56″ E',
|
||
'Location text is set as expected - if this fails it may be due to i18n issues.'
|
||
);
|
||
|
||
assert.strictEqual(
|
||
panel.$location.prop( 'href' ),
|
||
'http://tools.wmflabs.org/geohack/geohack.php?pagename=File:' + fileName + '¶ms=' + latitude + '_N_' + longitude + '_E_&language=en',
|
||
'Location URL is set as expected'
|
||
);
|
||
|
||
latitude = -latitude;
|
||
longitude = -longitude;
|
||
imageData.latitude = latitude;
|
||
imageData.longitude = longitude;
|
||
panel.setLocationData( imageData );
|
||
|
||
assert.strictEqual(
|
||
panel.$location.text(),
|
||
'Location: 12° 20′ 44.44″ S, 98° 45′ 55.56″ W',
|
||
'Location text is set as expected - if this fails it may be due to i18n issues.'
|
||
);
|
||
|
||
assert.strictEqual(
|
||
panel.$location.prop( 'href' ),
|
||
'http://tools.wmflabs.org/geohack/geohack.php?pagename=File:' + fileName + '¶ms=' + ( - latitude) + '_S_' + ( - longitude ) + '_W_&language=en',
|
||
'Location URL is set as expected'
|
||
);
|
||
|
||
latitude = 0;
|
||
longitude = 0;
|
||
imageData.latitude = latitude;
|
||
imageData.longitude = longitude;
|
||
panel.setLocationData( imageData );
|
||
|
||
assert.strictEqual(
|
||
panel.$location.text(),
|
||
'Location: 0° 0′ 0″ N, 0° 0′ 0″ E',
|
||
'Location text is set as expected - if this fails it may be due to i18n issues.'
|
||
);
|
||
|
||
assert.strictEqual(
|
||
panel.$location.prop( 'href' ),
|
||
'http://tools.wmflabs.org/geohack/geohack.php?pagename=File:' + fileName + '¶ms=' + latitude + '_N_' + longitude + '_E_&language=en',
|
||
'Location URL is set as expected'
|
||
);
|
||
} );
|
||
|
||
QUnit.test( 'Setting image information works as expected', 15, function ( assert ) {
|
||
var gender,
|
||
$qf = $( '#qunit-fixture' ),
|
||
panel = new mw.mmv.ui.MetadataPanel( $qf, $( '<div>' ).appendTo( $qf ) ),
|
||
title = 'Foo bar',
|
||
image = {
|
||
filePageTitle : mw.Title.newFromText( 'File:' + title + '.jpg' )
|
||
},
|
||
imageData = {
|
||
title: image.filePageTitle,
|
||
url: 'https://upload.wikimedia.org/wikipedia/commons/3/3a/Foobar.jpg',
|
||
descriptionUrl: 'https://commons.wikimedia.org/wiki/File:Foobar.jpg',
|
||
hasCoords: function() { return false; }
|
||
},
|
||
repoData = {
|
||
getArticlePath : function() { return 'Foo'; }
|
||
},
|
||
localUsage = {},
|
||
globalUsage = {},
|
||
oldMoment = window.moment;
|
||
|
||
/*window.moment = function( date ) {
|
||
// This has no effect for now, since writing this test revealed that our moment.js
|
||
// doesn't have any language configuration
|
||
return oldMoment( date ).lang( 'fr' );
|
||
};*/
|
||
|
||
panel.setImageInfo( image, imageData, repoData, localUsage, globalUsage, gender );
|
||
|
||
assert.strictEqual( panel.$title.text(), title, 'Title is correctly set' );
|
||
assert.ok( panel.$credit.hasClass( 'empty' ), 'Credit is empty' );
|
||
assert.ok( panel.$license.hasClass( 'empty' ), 'License is empty' );
|
||
assert.ok( panel.$usernameLi.hasClass( 'empty' ), 'Username is empty' );
|
||
assert.ok( panel.$datetimeLi.hasClass( 'empty' ), 'Date/Time is empty' );
|
||
assert.ok( panel.$locationLi.hasClass( 'empty' ), 'Location is empty' );
|
||
|
||
imageData.creationDateTime = '2013-08-26T14:41:02Z';
|
||
imageData.uploadDateTime = '2013-08-25T14:41:02Z';
|
||
imageData.source = '<b>Lost</b><a href="foo">Bar</a>';
|
||
imageData.author = 'Bob';
|
||
imageData.isCcLicensed = function() { return true; };
|
||
imageData.license = new mw.mmv.model.License( 'CC-BY-2.0', 'cc-by-2.0' );
|
||
gender = 'female';
|
||
imageData.lastUploader = 'Ursula';
|
||
|
||
panel.setImageInfo( image, imageData, repoData, localUsage, globalUsage, gender );
|
||
|
||
assert.strictEqual( panel.$title.text(), title, 'Title is correctly set' );
|
||
assert.ok( !panel.$credit.hasClass( 'empty' ), 'Credit is not empty' );
|
||
assert.ok( !panel.$datetimeLi.hasClass( 'empty' ), 'Date/Time is not empty' );
|
||
assert.strictEqual( panel.$source.html(), 'Lost<a href=\"foo\">Bar</a>', 'Source is correctly set' );
|
||
assert.ok( panel.$datetime.text().indexOf( 'August 26 2013' ) > 0, 'Correct date is displayed' );
|
||
assert.strictEqual( panel.$author.text(), imageData.author, 'Author is correctly set' );
|
||
assert.strictEqual( panel.$license.text(), 'CC BY 2.0', 'License is correctly set' );
|
||
assert.ok( panel.$username.text().indexOf( imageData.lastUploader ) > 0, 'Correct username is displayed' );
|
||
|
||
imageData.creationDateTime = undefined;
|
||
panel.setImageInfo( image, imageData, repoData, localUsage, globalUsage, gender );
|
||
|
||
assert.ok( panel.$datetime.text().indexOf( 'August 25 2013' ) > 0, 'Correct date is displayed' );
|
||
|
||
window.moment = oldMoment;
|
||
} );
|
||
|
||
QUnit.test( 'Setting permission information works as expected', 1, function ( assert ) {
|
||
var $qf = $( '#qunit-fixture' ),
|
||
panel = new mw.mmv.ui.MetadataPanel( $qf, $( '<div>' ).appendTo( $qf ) );
|
||
|
||
panel.setPermission( 'Look at me, I am a permission!' );
|
||
assert.ok( panel.$permissionLink.is( ':visible' ) );
|
||
} );
|
||
|
||
QUnit.test( 'Date formatting', 1, function ( assert ) {
|
||
var $qf = $( '#qunit-fixture' ),
|
||
panel = new mw.mmv.ui.MetadataPanel( $qf, $( '<div>' ).appendTo( $qf ) ),
|
||
date1 = 'Garbage',
|
||
result = panel.formatDate( date1 );
|
||
|
||
assert.strictEqual( result, date1, 'Invalid date is correctly ignored' );
|
||
} );
|
||
|
||
QUnit.test( 'Progress bar', 10, function ( assert ) {
|
||
var $qf = $( '#qunit-fixture' ),
|
||
panel = new mw.mmv.ui.MetadataPanel( $qf, $( '<div>' ).appendTo( $qf ) ),
|
||
oldAnimate = $.fn.animate;
|
||
|
||
assert.ok( panel.$progress.hasClass( 'empty' ), 'Progress bar is hidden' );
|
||
assert.strictEqual( panel.$percent.width(), 0, 'Progress bar\'s indicator is at 0' );
|
||
|
||
$.fn.animate = function ( target ) {
|
||
$( this ).css( target );
|
||
|
||
assert.strictEqual( target.width, '50%', 'Animation should go to 50%' );
|
||
};
|
||
|
||
panel.percent( 50 );
|
||
|
||
assert.ok( !panel.$progress.hasClass( 'empty' ), 'Progress bar is visible' );
|
||
assert.strictEqual( panel.$percent.width(), $qf.width() / 2, 'Progress bar\'s indicator is at half' );
|
||
|
||
$.fn.animate = function () {
|
||
assert.ok( false, 'Going to 0% should not animate' );
|
||
};
|
||
|
||
panel.percent( 0 );
|
||
|
||
assert.ok( panel.$progress.hasClass( 'empty' ), 'Progress bar is hidden' );
|
||
assert.strictEqual( panel.$percent.width(), 0, 'Progress bar\'s indicator is at 0' );
|
||
|
||
$.fn.animate = function ( target, duration, transition, callback ) {
|
||
$( this ).css( target );
|
||
|
||
assert.strictEqual( target.width, '100%', 'Animation should go to 100%' );
|
||
|
||
if ( callback !== undefined ) {
|
||
callback();
|
||
}
|
||
};
|
||
|
||
panel.percent( 100 );
|
||
|
||
assert.ok( panel.$progress.hasClass( 'empty' ), 'Progress bar is hidden' );
|
||
assert.strictEqual( panel.$percent.width(), 0, 'Progress bar\'s indicator is at 0' );
|
||
|
||
$.fn.animate = oldAnimate;
|
||
} );
|
||
|
||
QUnit.test( 'Metadata div is only animated once', 6, function ( assert ) {
|
||
localStorage.removeItem( 'mmv.hasOpenedMetadata' );
|
||
|
||
var $qf = $( '#qunit-fixture' ),
|
||
panel = new mw.mmv.ui.MetadataPanel( $qf, $( '<div>' ).appendTo( $qf ) );
|
||
|
||
panel.animateMetadataOnce();
|
||
|
||
assert.ok( panel.hasAnimatedMetadata,
|
||
'The first call to animateMetadataOnce set hasAnimatedMetadata to true' );
|
||
assert.ok( !$qf.hasClass( 'invited' ),
|
||
'After the first call to animateMetadataOnce led to an animation' );
|
||
assert.ok( $qf.hasClass( 'invite' ),
|
||
'The first call to animateMetadataOnce led to an animation' );
|
||
|
||
$qf.removeClass( 'invite' );
|
||
|
||
panel.animateMetadataOnce();
|
||
|
||
assert.strictEqual( panel.hasAnimatedMetadata, true, 'The second call to animateMetadataOnce did not change the value of hasAnimatedMetadata' );
|
||
assert.ok( $qf.hasClass( 'invited' ),
|
||
'After the second call to animateMetadataOnce the div is shown right away' );
|
||
assert.ok( !$qf.hasClass( 'invite' ),
|
||
'The second call to animateMetadataOnce did not lead to an animation' );
|
||
|
||
$qf.removeClass( 'invited' );
|
||
} );
|
||
}( mediaWiki, jQuery ) );
|