mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/MultimediaViewer
synced 2024-09-25 11:17:54 +00:00
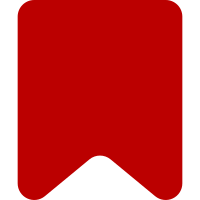
When opening the lightbox, the image might stay small because of a race condition. This is fixed using a deferred object. Change-Id: I4e93c7d26da47e5177abeb1dac841bf6c25a9022 Mingle: https://wikimedia.mingle.thoughtworks.com/projects/multimedia/cards/138
112 lines
2.5 KiB
JavaScript
112 lines
2.5 KiB
JavaScript
( function ( $ ) {
|
|
/**
|
|
* @class
|
|
* @constructor
|
|
* @param {string} src The URL (possibly relative) to the image
|
|
*/
|
|
function LightboxImage( src ) {
|
|
this.src = src;
|
|
|
|
lightboxHooks.callAll( 'modifyImageObject', this );
|
|
}
|
|
|
|
var LIP = LightboxImage.prototype;
|
|
|
|
/**
|
|
* The URL of the image (in the size we intend use to display the it in the lightbox)
|
|
* @type {String}
|
|
* @protected
|
|
*/
|
|
LIP.src = null;
|
|
|
|
/**
|
|
* The URL of a placeholder while the image loads. Typically a smaller version of the image, which is already
|
|
* loaded in the browser.
|
|
* @type {String}
|
|
* @protected
|
|
*/
|
|
LIP.initialSrc = null;
|
|
|
|
LIP.getImageElement = function () {
|
|
var ele,
|
|
$deferred = $.Deferred(),
|
|
image = this;
|
|
|
|
lightboxHooks.callAll( 'beforeFetchImage', this );
|
|
|
|
ele = new Image();
|
|
ele.addEventListener( 'error', $deferred.reject );
|
|
ele.addEventListener( 'load', function() { $deferred.resolve( image, ele ); } );
|
|
ele.src = this.src || this.initialSrc;
|
|
|
|
lightboxHooks.callAll( 'modifyImageElement', ele );
|
|
|
|
return $deferred;
|
|
};
|
|
|
|
// Assumes that the parent element's size is the maximum size.
|
|
LIP.autoResize = function ( ele, $parent ) {
|
|
function updateRatios() {
|
|
if ( imgHeight ) {
|
|
imgHeightRatio = parentHeight / imgHeight;
|
|
}
|
|
|
|
if ( imgWidth ) {
|
|
imgWidthRatio = parentWidth / imgWidth;
|
|
}
|
|
}
|
|
|
|
var imgWidthRatio, imgHeightRatio, parentWidth, parentHeight,
|
|
$img = $( ele ),
|
|
imgWidth = $img.width(),
|
|
imgHeight = $img.height();
|
|
|
|
$parent = $parent || $img.parent();
|
|
parentWidth = $parent.width();
|
|
parentHeight = $parent.height();
|
|
|
|
if ( this.globalMaxWidth && parentWidth > this.globalMaxWidth ) {
|
|
parentWidth = this.globalMaxWidth;
|
|
}
|
|
|
|
if ( this.globalMaxHeight && parentHeight > this.globalMaxHeight ) {
|
|
parentHeight = this.globalMaxHeight;
|
|
}
|
|
|
|
updateRatios();
|
|
|
|
if ( imgWidth > parentWidth ) {
|
|
imgHeight *= imgWidthRatio || 1;
|
|
imgWidth = parentWidth;
|
|
updateRatios();
|
|
}
|
|
|
|
if ( imgHeight > parentHeight ) {
|
|
imgWidth *= imgHeightRatio || 1;
|
|
imgHeight = parentHeight;
|
|
updateRatios();
|
|
}
|
|
|
|
if ( imgWidth < parentWidth && imgHeight < parentHeight ) {
|
|
if ( imgWidth === 0 && imgHeight === 0 ) {
|
|
// Only set one
|
|
imgWidth = parentWidth;
|
|
imgHeight = null;
|
|
} else {
|
|
if ( imgHeightRatio > imgWidthRatio ) {
|
|
imgWidth *= imgHeightRatio;
|
|
imgHeight = parentHeight;
|
|
} else {
|
|
imgHeight *= imgWidthRatio;
|
|
imgWidth = parentWidth;
|
|
}
|
|
updateRatios();
|
|
}
|
|
}
|
|
|
|
$img.width( imgWidth ).height( imgHeight );
|
|
};
|
|
|
|
window.LightboxImage = LightboxImage;
|
|
}( jQuery ) );
|