mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Math
synced 2024-12-20 11:30:55 +00:00
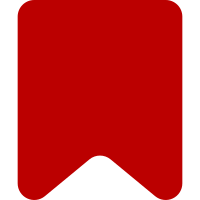
In I94887f6ccb45072180a318776ae96aead8658a18 some ams macros were not taken into account. Add \ to respective AMS macros. Bug: T380184 Change-Id: I7c59569fb7242f4f6123047003de6cc86ce868ea
73 lines
2.2 KiB
PHP
73 lines
2.2 KiB
PHP
<?php
|
|
|
|
namespace MediaWiki\Extension\Math\Tests\WikiTexVC\MMLmappings;
|
|
|
|
use MediaWiki\Extension\Math\WikiTexVC\MMLmappings\AMSMappings;
|
|
use MediaWiki\Extension\Math\WikiTexVC\MMLmappings\BaseParsing;
|
|
use PHPUnit\Framework\TestCase;
|
|
|
|
/**
|
|
* @covers \MediaWiki\Extension\Math\WikiTexVC\MMLmappings\AMSMappings
|
|
*/
|
|
class AmsMappingsTest extends TestCase {
|
|
|
|
public function testGetAll() {
|
|
$all = AMSMappings::getAll();
|
|
$this->assertIsArray( $all );
|
|
$this->assertNotEmpty( $all );
|
|
}
|
|
|
|
public function provideTestCases(): array {
|
|
// the second argument is an array of known problems which should be removed in the future
|
|
return [
|
|
'amsmacros' => [ 'amsmacros', [ 'MultiIntegral', 'HandleTag', 'HandleNoTag', 'HandleRef', 'HandleDeclareOp',
|
|
'HandleShove' ] ],
|
|
];
|
|
}
|
|
|
|
/**
|
|
* @dataProvider provideTestCases
|
|
*/
|
|
public function testValidMethods( $setName, $knownProblems = [] ) {
|
|
foreach ( AMSMappings::getAll()[$setName] as $symbol => $payload ) {
|
|
$methodName = is_array( $payload ) ? $payload[0] : $payload;
|
|
if ( in_array( $methodName, $knownProblems ) ) {
|
|
continue;
|
|
}
|
|
$this->assertTrue( method_exists( BaseParsing::class, $methodName ),
|
|
'Method ' . $methodName . ' for symbol ' . $symbol . ' does not exist in BaseParsing' );
|
|
|
|
}
|
|
}
|
|
|
|
public function testGetOperatorByKey() {
|
|
$this->assertEquals( '⨌', AMSMappings::getOperatorByKey( '\\iiiint' )[0] );
|
|
}
|
|
|
|
public function testGetMathDelimiterByKey() {
|
|
$this->assertEquals( '|', AMSMappings::getMathDelimiterByKey( '\\lvert' )[0] );
|
|
}
|
|
|
|
public function testGetSymbolDelimiterByKey() {
|
|
$this->assertEquals( '⌜', AMSMappings::getSymbolDelimiterByKey( '\\ulcorner' )[0] );
|
|
}
|
|
|
|
public function testGetMacroByKey() {
|
|
$this->assertEquals( 'Tilde', AMSMappings::getMacroByKey( '\\nobreakspace' )[0] );
|
|
$this->assertEquals( 'macro', AMSMappings::getMacroByKey( '\\implies' )[0] );
|
|
}
|
|
|
|
public function testGetInstance() {
|
|
$this->assertInstanceOf( AMSMappings::class, AMSMappings::getInstance() );
|
|
}
|
|
|
|
public function testGetIdentifierByKey() {
|
|
$this->assertEquals( 'Γ', AMSMappings::getIdentifierByKey( '\\varGamma' )[0] );
|
|
}
|
|
|
|
public function testGetEnvironmentByKey() {
|
|
$this->assertEquals( 'EqnArray', AMSMappings::getEnvironmentByKey( 'align' )[0] );
|
|
}
|
|
|
|
}
|