mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Math
synced 2024-11-13 17:56:59 +00:00
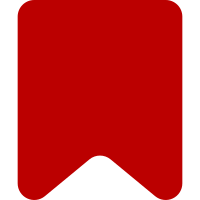
Actual changes: * Let the special page accept the subpage parameter, e.g. [[Special:MathWikibase/Q6203]]. * Fix some of the message escaping issues in SpecialMathWikibase. Messages that are just plain text and not supposed to contain any parameters, wikitext, HTML, or something like this should be properly escaped, either via Html::rawElement( $msg->escaped() ) or better Html::element( $msg->plain ). The later approach is cheaper. Other code cleanups that don't affect production: * Reduce the amount of duplicate code in SpecialMathWikibase. * Make use of the preg_match() return value. * Remove default parameters from parent::__construct() call. * Remove some auto-generated comments. * Remove some comments that don't add new information, but just repeat what the code already says. * Make use of PHPUnit's assertCount(). We can split this patch into smaller patches, if you prefer. This should especially be done if one of the changes is controversial, to not block the other changes. Please tell me. Change-Id: I8c20014435af3d2ac9c7381fe5fd00b9fe49b2c2
106 lines
3.3 KiB
PHP
106 lines
3.3 KiB
PHP
<?php
|
|
/**
|
|
* @author Moritz Schubotz
|
|
*/
|
|
require_once __DIR__ . '/../../../maintenance/Maintenance.php';
|
|
|
|
use MediaWiki\MediaWikiServices;
|
|
|
|
class WfTest extends Maintenance {
|
|
const REFERENCE_PAGE = 'mediawikiwiki:Extension:Math/CoverageTest';
|
|
|
|
public function __construct() {
|
|
parent::__construct();
|
|
$this->requireExtension( 'Math' );
|
|
$this->addDescription( 'Test Mathoid CLI' );
|
|
$this->addArg( 'page', "The page used for the testset generation.", false );
|
|
$this->addOption( 'offset', "If set the first n equations on the page are skipped", false,
|
|
true, "o" );
|
|
$this->addOption( 'length', "If set the only n equations were processed", false, true,
|
|
"l" );
|
|
$this->addOption( 'user', "User with rights to view the page", false, true, "u" );
|
|
}
|
|
|
|
private static function getMathTagsFromPage( $titleString ) {
|
|
global $wgEnableScaryTranscluding;
|
|
$title = Title::newFromText( $titleString );
|
|
if ( $title->exists() ) {
|
|
$article = new Article( $title );
|
|
$wikiText = $article->getPage()->getContent()->getNativeData();
|
|
} else {
|
|
if ( $title == self::REFERENCE_PAGE ) {
|
|
$wgEnableScaryTranscluding = true;
|
|
$parser = MediaWikiServices::getInstance()->getParserFactory()->create();
|
|
$wikiText = $parser->interwikiTransclude( $title, 'raw' );
|
|
} else {
|
|
return 'Page does not exist';
|
|
}
|
|
}
|
|
|
|
$wikiText = Sanitizer::removeHTMLcomments( $wikiText );
|
|
$wikiText = preg_replace( '#<nowiki>(.*)</nowiki>#', '', $wikiText );
|
|
$math = [];
|
|
Parser::extractTagsAndParams( [ 'math' ], $wikiText, $math );
|
|
|
|
return $math;
|
|
}
|
|
|
|
public function execute() {
|
|
echo "This test accesses the Mathoid CLI.\n";
|
|
$page = $this->getArg( 0, self::REFERENCE_PAGE );
|
|
$offset = $this->getOption( 'offset', 0 );
|
|
$length = $this->getOption( 'length', PHP_INT_MAX );
|
|
$userName = $this->getOption( 'user', 'Maintenance script' );
|
|
$wgUser = User::newFromName( $userName );
|
|
$allEquations = self::getMathTagsFromPage( $page );
|
|
if ( !is_array( $allEquations ) ) {
|
|
echo "Could not get equations from page '$page'\n";
|
|
echo $allEquations . PHP_EOL;
|
|
|
|
return;
|
|
} else {
|
|
echo 'got ' . count( $allEquations ) . " math tags. Start processing.";
|
|
}
|
|
$i = 0;
|
|
$rend = [];
|
|
foreach ( array_slice( $allEquations, $offset, $length, true ) as $input ) {
|
|
$output = MathRenderer::renderMath( $input[1], $input[2], 'mathml' );
|
|
$rend[] = [ MathRenderer::getRenderer( $input[1], $input[2], 'mathml' ), $input ];
|
|
$output = preg_replace( '#src="(.*?)/(([a-f]|\d)*).png"#', 'src="\2.png"', $output );
|
|
$parserTests[] = [ (string)$input[1], $output ];
|
|
$i++;
|
|
echo '.';
|
|
}
|
|
echo "Generated $i tests\n";
|
|
MathMathMLCli::batchEvaluate( $rend );
|
|
$retval = null;
|
|
$stdout = "[
|
|
{
|
|
\"query\": {
|
|
\"q\": \"E=mc^{2}\"
|
|
}}]";
|
|
|
|
// $f = TempFSFile::factory( 'mathoid', 'json', wfTempDir() );
|
|
// $f->autocollect();
|
|
// $fhandle = fopen( $f->getPath(), 'w' );
|
|
// if ( $fhandle ) {
|
|
// fwrite( $fhandle, $stdout );
|
|
// fclose( $fhandle );
|
|
// }
|
|
// $contents =
|
|
// wfShellExec( '/tmp/mathoid/cli.js -c /tmp/mathoid/config.dev.yaml ' . $f->getPath(),
|
|
// $retval );
|
|
// $contents =
|
|
// wfShellExecMath( '/tmp/mathoid/cli.js -c /tmp/mathoid/config.dev.yaml ',
|
|
// $retval, [], [], [], $stdout );
|
|
|
|
// if ( $retval == 0 ) {
|
|
// $res = json_decode( $contents, true );
|
|
// echo "JSON result" . var_export( $res, false ) . "\n";
|
|
// }
|
|
}
|
|
}
|
|
|
|
$maintClass = WfTest::class;
|
|
require_once RUN_MAINTENANCE_IF_MAIN;
|