mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Math
synced 2024-11-13 17:56:59 +00:00
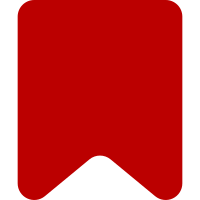
* eslint-config-wikimedia: 0.22.1 → 0.24.0 Resolve newly detected error: * mediawiki/no-nodelist-unsupported-methods Change-Id: If43479e88a3d39f507541f3f6b53f23004bd0e1e
54 lines
1.4 KiB
JavaScript
54 lines
1.4 KiB
JavaScript
( function () {
|
|
'use strict';
|
|
var previewType = 'math';
|
|
var api = new mw.Rest();
|
|
var isValidId = function ( qid ) {
|
|
return qid.match( /Q\d+/g ) === null;
|
|
};
|
|
var fetch = function ( qid ) {
|
|
return api.get( '/math/v0/popup/html/' + qid, {}, {
|
|
Accept: 'application/json; charset=utf-8',
|
|
'Accept-Language': mw.config.language
|
|
} );
|
|
};
|
|
var fetchPreviewForTitle = function ( title, el ) {
|
|
var deferred = $.Deferred();
|
|
var qidstr = el.parentNode.parentNode.dataset.qid;
|
|
if ( isValidId( qidstr ) ) {
|
|
return deferred.reject();
|
|
}
|
|
qidstr = qidstr.slice( 1 );
|
|
fetch( qidstr ).then( function ( body ) {
|
|
var model = {
|
|
title: body.title,
|
|
url: body.canonicalurl,
|
|
languageCode: body.pagelanguagehtmlcode,
|
|
languageDirection: body.pagelanguagedir,
|
|
extract: body.extract,
|
|
type: previewType,
|
|
thumbnail: undefined,
|
|
pageId: body.pageId
|
|
};
|
|
deferred.resolve( model );
|
|
} );
|
|
return deferred.promise();
|
|
};
|
|
// popups require title attributes
|
|
[].forEach.call(
|
|
document.querySelectorAll( '.mwe-math-element[data-qid] img' ),
|
|
function ( node ) {
|
|
if ( isValidId( node.parentNode.parentNode.dataset.qid ) ) {
|
|
node.dataset.title = 'math-unique-identifier';
|
|
}
|
|
}
|
|
);
|
|
module.exports = {
|
|
type: previewType,
|
|
selector: '.mwe-math-element[data-qid] img',
|
|
gateway: {
|
|
fetch: fetch,
|
|
fetchPreviewForTitle: fetchPreviewForTitle
|
|
}
|
|
};
|
|
}() );
|