mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Math
synced 2024-12-04 12:09:04 +00:00
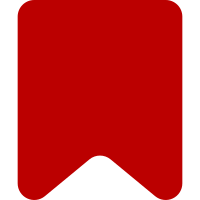
* add some basic tests * migrate MathWikibaseConnectorTest to a unit test Bug: T313331 Depends-On: Ic3b8d4f685d5cf648a02696284b6ee499502a12a Change-Id: I38d6425eb5e2c52ae4362c4b8656223a8d9d90a5
98 lines
3 KiB
PHP
98 lines
3 KiB
PHP
<?php
|
|
|
|
namespace MediaWiki\Extension\Math\Tests;
|
|
|
|
use HashConfig;
|
|
use MediaWiki\Extension\Math\MathWikibaseConfig;
|
|
use MediaWiki\Extension\Math\MathWikibaseConnector;
|
|
use MediaWiki\Languages\LanguageFactory;
|
|
use MediaWikiUnitTestCase;
|
|
use MWException;
|
|
use Site;
|
|
use TestLogger;
|
|
use Wikibase\Client\RepoLinker;
|
|
use Wikibase\DataAccess\DatabaseEntitySource;
|
|
use Wikibase\DataAccess\EntitySourceDefinitions;
|
|
use Wikibase\DataModel\Entity\BasicEntityIdParser;
|
|
use Wikibase\Lib\Store\EntityRevisionLookup;
|
|
use Wikibase\Lib\Store\FallbackLabelDescriptionLookupFactory;
|
|
use Wikibase\Lib\SubEntityTypesMapper;
|
|
use const MWException;
|
|
|
|
/**
|
|
* @covers \MediaWiki\Extension\Math\MathWikibaseConnector
|
|
*/
|
|
class MathWikibaseConnectorTest extends MediaWikiUnitTestCase {
|
|
|
|
private const EXAMPLE_URL = 'https://example.com/';
|
|
|
|
public function testGetUrl() {
|
|
$mathWikibase = $this->getWikibaseConnector();
|
|
$this->assertEquals( self::EXAMPLE_URL . 'wiki/Special:EntityPage/Q42',
|
|
$mathWikibase->buildURL( 'Q42' ) );
|
|
}
|
|
|
|
public function testFetchInvalidLanguage() {
|
|
$languageFactory = $this->createMock( LanguageFactory::class );
|
|
$languageFactory->method( 'getLanguage' )
|
|
->willThrowException( new MWException( 'Invalid code' ) );
|
|
$mathWikibase = $this->getWikibaseConnector( $languageFactory );
|
|
|
|
$this->expectException( 'InvalidArgumentException' );
|
|
$this->expectErrorMessage( 'Invalid language code specified.' );
|
|
$mathWikibase->fetchWikibaseFromId( 'Q1', '&' );
|
|
}
|
|
|
|
public function testFetchNonExistingId() {
|
|
$mathWikibase = $this->getWikibaseConnector();
|
|
$this->expectException( 'InvalidArgumentException' );
|
|
$this->expectErrorMessage( 'Non-existing Wikibase ID.' );
|
|
$mathWikibase->fetchWikibaseFromId( 'Q1', 'en' );
|
|
}
|
|
|
|
private function newConnector(): RepoLinker {
|
|
return new RepoLinker(
|
|
new EntitySourceDefinitions(
|
|
[
|
|
new DatabaseEntitySource(
|
|
'test',
|
|
'testdb',
|
|
[ 'item' => [ 'namespaceId' => 123, 'slot' => 'main' ] ],
|
|
self::EXAMPLE_URL . 'entity',
|
|
'',
|
|
'',
|
|
''
|
|
)
|
|
],
|
|
new SubEntityTypesMapper( [] )
|
|
),
|
|
self::EXAMPLE_URL,
|
|
'/wiki/$1',
|
|
'' );
|
|
}
|
|
|
|
/**
|
|
* @param LanguageFactory|null $languageFactory
|
|
* @return MathWikibaseConnector
|
|
*/
|
|
public function getWikibaseConnector( LanguageFactory $languageFactory = null ): MathWikibaseConnector {
|
|
$entityRevisionLookup = $this->createMock( EntityRevisionLookup::class );
|
|
$labelDescriptionLookupFactory = $this->createMock( FallbackLabelDescriptionLookupFactory::class );
|
|
$languageFactory = $languageFactory ?: $this->createMock( LanguageFactory::class );
|
|
return new MathWikibaseConnector( new MathWikibaseConfig(
|
|
new BasicEntityIdParser(),
|
|
$entityRevisionLookup,
|
|
$labelDescriptionLookupFactory,
|
|
new Site(),
|
|
new HashConfig( [
|
|
'MathWikibasePropertyIdHasPart' => 'P1',
|
|
'MathWikibasePropertyIdDefiningFormula' => 'P2',
|
|
'MathWikibasePropertyIdQuantitySymbol' => 'P3'
|
|
] ) ),
|
|
$this->newConnector(),
|
|
$languageFactory,
|
|
new TestLogger() );
|
|
}
|
|
|
|
}
|