mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Math
synced 2024-12-12 07:55:11 +00:00
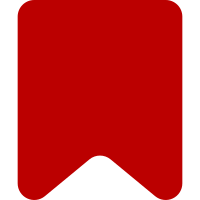
To reduce the complexity of the parse tree we remove the curly element which is used for grouping in TeX. Instead, we use use an attribute which defines if the group is put into curly brackets or not. The functionality of the curly element is transferred to the TexArray which was the only possible child of the curly element before. To ease the transition, we add a special constructor to TexArray. We could not measure any performance implications of this change. Bug: T333973 Change-Id: Idcb58694022831113bdc437576bb9f48658fff2f
60 lines
1.8 KiB
PHP
60 lines
1.8 KiB
PHP
<?php
|
|
|
|
namespace MediaWiki\Extension\Math\Tests\WikiTexVC\Nodes;
|
|
|
|
use ArgumentCountError;
|
|
use MediaWiki\Extension\Math\WikiTexVC\MMLnodes\MMLmrow;
|
|
use MediaWiki\Extension\Math\WikiTexVC\Nodes\DQ;
|
|
use MediaWiki\Extension\Math\WikiTexVC\Nodes\Literal;
|
|
use MediaWiki\Extension\Math\WikiTexVC\Nodes\TexArray;
|
|
use MediaWiki\Extension\Math\WikiTexVC\Nodes\TexNode;
|
|
use MediaWikiUnitTestCase;
|
|
use RuntimeException;
|
|
use TypeError;
|
|
|
|
/**
|
|
* @covers \MediaWiki\Extension\Math\WikiTexVC\Nodes\DQ
|
|
*/
|
|
class DQTest extends MediaWikiUnitTestCase {
|
|
|
|
public function testEmptyDQ() {
|
|
$this->expectException( ArgumentCountError::class );
|
|
new DQ();
|
|
throw new ArgumentCountError( 'Should not create an empty dq' );
|
|
}
|
|
|
|
public function testOneArgumentDQ() {
|
|
$this->expectException( ArgumentCountError::class );
|
|
new DQ( new Literal( 'a' ) );
|
|
throw new ArgumentCountError( 'Should not create a dq with one argument' );
|
|
}
|
|
|
|
public function testIncorrectTypeDQ() {
|
|
$this->expectException( TypeError::class );
|
|
new DQ( 'a', 'b' );
|
|
throw new RuntimeException( 'Should not create a dq with incorrect type' );
|
|
}
|
|
|
|
public function testBasicDQ() {
|
|
$dq = new DQ( new Literal( 'a' ), new Literal( 'b' ) );
|
|
$this->assertEquals( 'a_{b}', $dq->render(), 'Should create a basic dq' );
|
|
}
|
|
|
|
public function testGetters() {
|
|
$dq = new DQ( new Literal( 'a' ), new Literal( 'b' ) );
|
|
$this->assertNotEmpty( $dq->getBase() );
|
|
$this->assertNotEmpty( $dq->getDown() );
|
|
}
|
|
|
|
public function testEmptyBaseDQ() {
|
|
$dq = new DQ( new TexNode(), new Literal( 'b' ) );
|
|
$this->assertEquals( '_{b}', $dq->render(), 'Should create an empty base dq' );
|
|
}
|
|
|
|
public function testRenderEmptyDq() {
|
|
$dq = new DQ( TexArray::newCurly(), new Literal( 'b' ) );
|
|
$this->assertStringContainsString( ( new MMLmrow() )->getEmpty(), $dq->renderMML() );
|
|
}
|
|
|
|
}
|