mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Math
synced 2024-09-24 10:49:18 +00:00
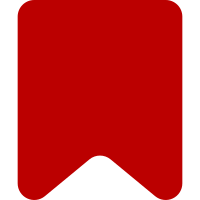
The LaTeXML class contains some code that is not specific to LaTeXML but to MathML. This code can be shared between LaTeXML and Mathoid. This change introduces a new class called MathMathML and moves the shared code from the LaTeXML class to this new class. Bug: 65973 Change-Id: I50517ba83f9a0d2aa8e237f062f18e4319ddbac8
158 lines
4.3 KiB
PHP
158 lines
4.3 KiB
PHP
<?php
|
|
/**
|
|
* Test the MathML output format.
|
|
*
|
|
* @group Math
|
|
*/
|
|
class MathMathMLTest extends MediaWikiTestCase {
|
|
|
|
// State-variables for HTTP Mockup classes
|
|
public static $content = null;
|
|
public static $good = false;
|
|
public static $html = false;
|
|
public static $timeout = false;
|
|
|
|
/**
|
|
* Set the mock values for the HTTP Mockup classes
|
|
*
|
|
* @param boolean $good
|
|
* @param mixed $html HTML of the error message or false if no error is present.
|
|
* @param boolean $timeout true if
|
|
*/
|
|
public static function setMockValues( $good, $html, $timeout ) {
|
|
self::$good = $good;
|
|
self::$html = $html;
|
|
self::$timeout = $timeout;
|
|
}
|
|
|
|
/**
|
|
* Tests behavior of makeRequest() that communicates with the host.
|
|
* Testcase: Invalid request.
|
|
* @covers MathTexvc::makeRequest
|
|
*/
|
|
public function testMakeRequestInvalid() {
|
|
self::setMockValues( false, false, false );
|
|
$url = 'http://example.com/invalid';
|
|
|
|
$renderer = $this->getMockBuilder( 'MathMathML' )
|
|
->setMethods( NULL )
|
|
->disableOriginalConstructor()
|
|
->getMock();
|
|
$requestReturn = $renderer->makeRequest( $url, 'a+b', $res, $error
|
|
, 'MathMLHttpRequestTester' );
|
|
$this->assertEquals( false, $requestReturn
|
|
, "requestReturn is false if HTTP::post returns false." );
|
|
$this->assertEquals( false, $res
|
|
, "res is false if HTTP:post returns false." );
|
|
$errmsg = wfMessage( 'math_invalidresponse', '', $url, '' )->inContentLanguage()->escaped();
|
|
$this->assertContains( $errmsg, $error
|
|
, "return an error if HTTP::post returns false" );
|
|
}
|
|
|
|
/**
|
|
* Tests behavior of makeRequest() that communicates with the host.
|
|
* Testcase: Valid request.
|
|
* @covers MathTexvc::makeRequest
|
|
*/
|
|
public function testMakeRequestSuccess() {
|
|
self::setMockValues( true, true, false );
|
|
$url = 'http://example.com/valid';
|
|
$renderer = $this->getMockBuilder( 'MathMathML' )
|
|
->setMethods( NULL )
|
|
->disableOriginalConstructor()
|
|
->getMock();
|
|
$requestReturn = $renderer->makeRequest( $url, 'a+b', $res, $error
|
|
, 'MathMLHttpRequestTester' );
|
|
$this->assertEquals( true, $requestReturn, "successful call return" );
|
|
$this->isTrue( $res, "successfull call" );
|
|
$this->assertEquals( $error, '', "successfull call errormessage" );
|
|
}
|
|
|
|
/**
|
|
* Tests behavior of makeRequest() that communicates with the host.
|
|
* Testcase: Timeout.
|
|
* @covers MathMathML::makeRequest
|
|
*/
|
|
public function testMakeRequestTimeout() {
|
|
self::setMockValues( false, true, true );
|
|
$url = 'http://example.com/timeout';
|
|
$renderer = $this->getMockBuilder( 'MathMathML' )
|
|
->setMethods( NULL )
|
|
->disableOriginalConstructor()
|
|
->getMock();
|
|
$requestReturn = $renderer->makeRequest( $url, '$\longcommand$', $res
|
|
, $error, 'MathMLHttpRequestTester' );
|
|
$this->assertEquals( false, $requestReturn, "timeout call return" );
|
|
$this->assertEquals( false, $res, "timeout call return" );
|
|
$errmsg = wfMessage( 'math_timeout', '', $url )->inContentLanguage()->escaped();
|
|
$this->assertContains( $errmsg, $error, "timeout call errormessage" );
|
|
}
|
|
|
|
|
|
/**
|
|
* Checks if a String is a valid MathML element
|
|
* @covers MathMathML::isValidXML
|
|
*/
|
|
public function testisValidXML() {
|
|
$renderer = $this->getMockBuilder( 'MathMathML' )
|
|
->setMethods( NULL )
|
|
->disableOriginalConstructor()
|
|
->getMock();
|
|
$validSample = '<math>content</math>';
|
|
$invalidSample = '<notmath />';
|
|
$this->assertTrue( $renderer->isValidMathML( $validSample ), 'test if math expression is valid mathml sample' );
|
|
$this->assertFalse( $renderer->isValidMathML( $invalidSample ), 'test if math expression is invalid mathml sample' );
|
|
}
|
|
|
|
}
|
|
|
|
/**
|
|
* Helper class for testing
|
|
* @author physikerwelt
|
|
* @see MWHttpRequestTester
|
|
*
|
|
*/
|
|
class MathMLHttpRequestTester {
|
|
|
|
public static function factory() {
|
|
return new MathMLHttpRequestTester();
|
|
}
|
|
|
|
public static function execute() {
|
|
return new MathMLTestStatus();
|
|
}
|
|
|
|
public static function getContent() {
|
|
return MathMathMLTest::$content;
|
|
}
|
|
|
|
}
|
|
|
|
/**
|
|
* Helper class for testing
|
|
* @author physikerwelt
|
|
* @see Status
|
|
*/
|
|
class MathMLTestStatus {
|
|
|
|
static function isGood() {
|
|
return MathMathMLTest::$good;
|
|
}
|
|
|
|
static function hasMessage( $s ) {
|
|
if ( $s == 'http-timed-out' ) {
|
|
return MathMathMLTest::$timeout;
|
|
} else {
|
|
return false;
|
|
}
|
|
}
|
|
|
|
static function getHtml() {
|
|
return MathMathMLTest::$html;
|
|
}
|
|
|
|
static function getWikiText() {
|
|
return MathMathMLTest::$html;
|
|
}
|
|
|
|
} |