mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Math
synced 2024-12-20 03:20:45 +00:00
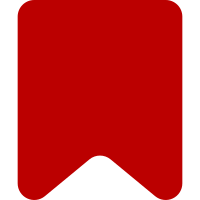
* Transform structure rich information on LaTeX length specifications introduced in I08996129beca9ad852d23f28d7136c982707aea1 into new TexNode class * Adjust rows, i.e., arrays to hold length information * Add tests Bug: T380654 Change-Id: I1aff53c7a5782c1333ed4c16639dfc660802931c
45 lines
1.3 KiB
PHP
45 lines
1.3 KiB
PHP
<?php
|
|
namespace MediaWiki\Extension\Math\Tests\WikiTexVC\Nodes;
|
|
|
|
use ArgumentCountError;
|
|
use InvalidArgumentException;
|
|
use MediaWiki\Extension\Math\WikiTexVC\Nodes\LengthSpec;
|
|
use MediaWikiUnitTestCase;
|
|
use TypeError;
|
|
|
|
/**
|
|
* @covers \MediaWiki\Extension\Math\WikiTexVC\Nodes\LengthSpec
|
|
*/
|
|
class LengthSpecTest extends MediaWikiUnitTestCase {
|
|
|
|
public function testEmptyLengthSpec() {
|
|
$this->expectException( ArgumentCountError::class );
|
|
new LengthSpec();
|
|
}
|
|
|
|
public function testInvalidNumber() {
|
|
$this->expectException( InvalidArgumentException::class );
|
|
new LengthSpec( '+', [ '1' ], 'pt' );
|
|
}
|
|
|
|
public function testNullUnit() {
|
|
$this->expectException( TypeError::class );
|
|
new LengthSpec( '+', [ '1', '.', '0' ], null );
|
|
}
|
|
|
|
public function testRenderLengthSpec() {
|
|
$lengthSpec = new LengthSpec( '+', [ [ '1' ], '.', [ '0' ] ], 'pt' );
|
|
$this->assertEquals( '[+1.0pt]', $lengthSpec->render() );
|
|
}
|
|
|
|
public function testRenderLengthSpec2() {
|
|
$lengthSpec = new LengthSpec( '+', [ '.', [ '0' ] ], 'pt' );
|
|
$this->assertEquals( '[+.0pt]', $lengthSpec->render() );
|
|
}
|
|
|
|
public function testRenderLengthSpecLong() {
|
|
$lengthSpec = new LengthSpec( null, [ [ '1', '2', '3' ], '.', [ '4', '5', '6' ] ], 'pt' );
|
|
$this->assertEquals( '[123.456pt]', $lengthSpec->render() );
|
|
}
|
|
}
|