mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Math
synced 2024-11-13 17:56:59 +00:00
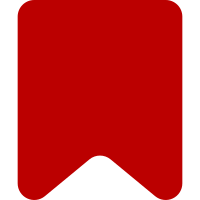
Set to true per default. In order to be able to switch it off in production for a coordinated deployment. Change-Id: I520b2ff1ae6dc3bad4b6067f0b673821d3c01cb4
69 lines
2.1 KiB
PHP
69 lines
2.1 KiB
PHP
<?php
|
|
|
|
use ValueFormatters\FormatterOptions;
|
|
use ValueParsers\StringParser;
|
|
use Wikibase\Repo\Parsers\WikibaseStringValueNormalizer;
|
|
use Wikibase\Repo\WikibaseRepo;
|
|
|
|
class MathWikidataHook {
|
|
|
|
/**
|
|
* Add Datatype "Math" to the Wikibase Repository
|
|
*/
|
|
public static function onWikibaseRepoDataTypes( array &$dataTypeDefinitions ) {
|
|
global $wgMathEnableWikibaseDataType;
|
|
|
|
if ( !$wgMathEnableWikibaseDataType ) {
|
|
return;
|
|
}
|
|
|
|
$dataTypeDefinitions['PT:math'] = array(
|
|
'value-type' => 'string',
|
|
'validator-factory-callback' => function() {
|
|
// load validator builders
|
|
$factory = WikibaseRepo::getDefaultValidatorBuilders();
|
|
|
|
// initialize an array with string validators
|
|
// returns an array of validators
|
|
// that add basic string validation such as preventing empty strings
|
|
$validators = $factory->buildStringValidators();
|
|
$validators[] = new MathValidator();
|
|
return $validators;
|
|
},
|
|
'parser-factory-callback' => function( ParserOptions $options ) {
|
|
$repo = WikibaseRepo::getDefaultInstance();
|
|
$normalizer = new WikibaseStringValueNormalizer( $repo->getStringNormalizer() );
|
|
return new StringParser( $normalizer );
|
|
},
|
|
'formatter-factory-callback' => function( $format, FormatterOptions $options ) {
|
|
global $wgOut;
|
|
$styles = array( 'ext.math.desktop.styles', 'ext.math.scripts', 'ext.math.styles' );
|
|
$wgOut->addModuleStyles( $styles );
|
|
return new MathFormatter( $format );
|
|
},
|
|
);
|
|
}
|
|
|
|
/*
|
|
* Add Datatype "Math" to the Wikibase Client
|
|
*/
|
|
public static function onWikibaseClientDataTypes( array &$dataTypeDefinitions ) {
|
|
global $wgMathEnableWikibaseDataType;
|
|
|
|
if ( !$wgMathEnableWikibaseDataType ) {
|
|
return;
|
|
}
|
|
|
|
$dataTypeDefinitions['PT:math'] = array(
|
|
'value-type' => 'string',
|
|
'formatter-factory-callback' => function( $format, FormatterOptions $options ) {
|
|
global $wgOut;
|
|
$styles = array( 'ext.math.desktop.styles', 'ext.math.scripts', 'ext.math.styles' );
|
|
$wgOut->addModuleStyles( $styles );
|
|
return new MathFormatter( $format );
|
|
},
|
|
);
|
|
}
|
|
|
|
}
|