mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Math
synced 2024-11-13 17:56:59 +00:00
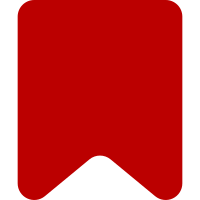
The following sniffs are failing and were disabled: * MediaWiki.Commenting.FunctionComment.DuplicateReturn * MediaWiki.Commenting.FunctionComment.MissingParamComment * MediaWiki.Commenting.FunctionComment.MissingParamName * MediaWiki.Commenting.FunctionComment.MissingParamTag * MediaWiki.Commenting.FunctionComment.MissingReturn * MediaWiki.Commenting.FunctionComment.ParamNameNoMatch * MediaWiki.Commenting.FunctionComment.WrongStyle * MediaWiki.FunctionComment.Missing.Protected * MediaWiki.FunctionComment.Missing.Public * MediaWiki.WhiteSpace.SpaceBeforeSingleLineComment.NewLineComment Change-Id: I46c900a5652a6560d18be6cd67badc37ed7f8d97
51 lines
1.2 KiB
PHP
51 lines
1.2 KiB
PHP
<?php
|
|
|
|
use DataValues\StringValue;
|
|
use ValueFormatters\Exceptions\MismatchingDataValueTypeException;
|
|
use ValueValidators\Error;
|
|
use ValueValidators\Result;
|
|
use ValueValidators\ValueValidator;
|
|
|
|
// @author Duc Linh Tran, Julian Hilbig, Moritz Schubotz
|
|
|
|
class MathValidator implements ValueValidator {
|
|
|
|
/**
|
|
* Validates a value with MathInputCheckRestbase
|
|
*
|
|
* @param mixed $value The value to validate
|
|
*
|
|
* @return \ValueValidators\Result
|
|
* @throws ValueFormatters\Exceptions\MismatchingDataValueTypeException
|
|
*/
|
|
public function validate( $value ) {
|
|
if ( !( $value instanceof StringValue ) ) {
|
|
throw new MismatchingDataValueTypeException( 'StringValue', get_class( $value ) );
|
|
}
|
|
|
|
// get input String from value
|
|
$tex = $value->getValue();
|
|
|
|
$checker = new MathInputCheckRestbase( $tex );
|
|
if ( $checker->isValid() ) {
|
|
return Result::newSuccess();
|
|
}
|
|
|
|
// TeX string is not valid
|
|
return Result::newError(
|
|
[
|
|
Error::newError( null, null, 'malformed-value', [ $checker->getError() ] )
|
|
]
|
|
);
|
|
}
|
|
|
|
/**
|
|
* @see ValueValidator::setOptions()
|
|
*
|
|
* @param array $options
|
|
*/
|
|
public function setOptions( array $options ) {
|
|
// Do nothing. This method shouldn't even be in the interface.
|
|
}
|
|
}
|